Table of Contents
- Troubleshooting and Fixing Camera Shaking Issues in Unity
- 1. Understand the Cause of Camera Shake
- 2. Unity Camera Stabilization Techniques
- 3. Camera Shake Reduction in Unity
- 4. Debugging Camera Movement Issues
- 5. Unity Scripting for Camera Control
- 6. Optimize Camera Dynamics in Unity
- 7. Troubleshoot Unity Camera Shake
Troubleshooting and Fixing Camera Shaking Issues in Unity
1. Understand the Cause of Camera Shake
Camera shaking in Unity can result from several factors such as inaccurate physics simulations, incorrect camera-parent relationships, or poorly handled input controls. Identifying the root cause is crucial before attempting any fixes.
2. Unity Camera Stabilization Techniques
- Rigidbody Interpolation: If your camera is attached to a Rigidbody, ensure that interpolation is enabled. This helps smooth out camera movements when following a dynamic object.
- FixedUpdate Method: Apply movement logic within the FixedUpdate method, which is aligned with the physics timestep, to minimize jittery effects.
3. Camera Shake Reduction in Unity
- Smooth Damp: Use
Vector3.SmoothDamp
for smoother transitions when moving or rotating the camera. It provides a dampened effect based on velocity smoothing. - Update Frequency: Ensure the camera updates are tied to the appropriate frequency, often using
LateUpdate()
to avoid dependency on frame rate.
4. Debugging Camera Movement Issues
- Debugging Tools: Utilize Unity’s debugging tools to visualize the camera’s position, using Gizmos to check for unintended movement or inappropriate rotations.
- Console Logs: Use
Debug.Log()
statements to monitor and confirm the expected movement path of your camera, which can help identify discrepancies.
5. Unity Scripting for Camera Control
using UnityEngine;
public class CameraController : MonoBehaviour {
public Transform target;
public float smoothTime = 0.3F;
private Vector3 velocity = Vector3.zero;
void LateUpdate() {
Vector3 targetPosition = target.TransformPoint(new Vector3(0, 5, -10));
transform.position = Vector3.SmoothDamp(transform.position, targetPosition, ref velocity, smoothTime);
}
}
6. Optimize Camera Dynamics in Unity
- Hierarchy Setup: Ensure that the camera is properly parented, ideally as a child of a stable object, to minimize unintended jitter from a dynamic scene.
- Entity-Component-System: Consider using Unity’s ECS for efficient data-oriented transformations and smoother camera operations in complex scenes.
7. Troubleshoot Unity Camera Shake
Investigate interaction problems with other scripts or unexpected behaviors due to physics calculations. Sometimes, even minor adjustments to collider properties and mass settings can lead to significant improvements in game performance and camera stability.
Play free games on Playgama.com
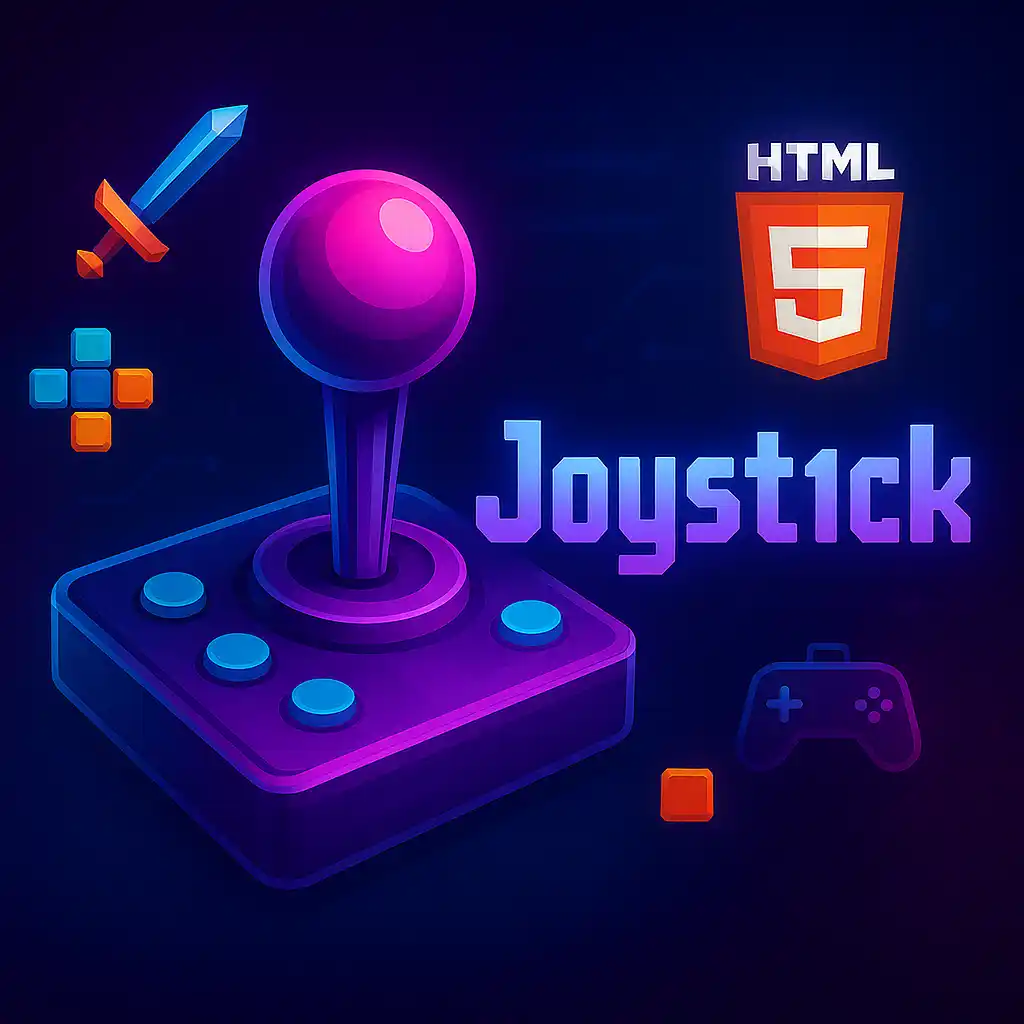