Table of Contents
Remapping WASD and Arrow Keys for Customizable Controls
Introduction to Keybinding
Providing customizable controls for players is crucial for enhancing the player experience, allowing for flexibility and accessibility in the game’s control scheme. This is particularly important in game development where player comfort and accessibility are priorities.
Implementing Keybinding in SFML
SFML (Simple and Fast Multimedia Library) offers a straightforward way to handle keyboard inputs, making it ideal for implementing custom key mappings:
Play free games on Playgama.com
#include <SFML/Graphics.hpp>
#include <map>
std::map<sf::Keyboard::Key, sf::Vector2f> keyBindings;
// Setting default keybindings
keyBindings[sf::Keyboard::W] = sf::Vector2f(0, -1);
keyBindings[sf::Keyboard::S] = sf::Vector2f(0, 1);
keyBindings[sf::Keyboard::A] = sf::Vector2f(-1, 0);
keyBindings[sf::Keyboard::D] = sf::Vector2f(1, 0);
sf::RenderWindow window(sf::VideoMode(800, 600), "Custom Controls");
while (window.isOpen()) {
sf::Event event;
while (window.pollEvent(event)) {
if (event.type == sf::Event::Closed)
window.close();
}
// Handle key inputs
for (auto &binding : keyBindings) {
if (sf::Keyboard::isKeyPressed(binding.first)) {
// Apply movement based on key binding
player.move(binding.second);
}
}
window.clear();
// Draw your game objects here
window.display();
}
Designing Custom Key Configurations
Allowing users to remap keys involves providing an interface where they can customize these keybindings. This could be a simple settings menu where key actions are visualized and can be re-assigned through either a key press or a dropdown menu.
Considerations for Different Input Schemes
- Compatibility: Ensure your keybinding implementation works across different keyboards and input devices.
- Feedback: Use visual or audio feedback when a key is successfully remapped.
- Saving Preferences: Implement a system to save and load user preferences, such as a configuration file or through player prefs.
Conclusion
Implementing remappable WASD and arrow keys using SFML allows developers to cater to various player preferences, enhancing the overall user experience. This not only makes your game more accessible but also more engaging for a diverse audience.
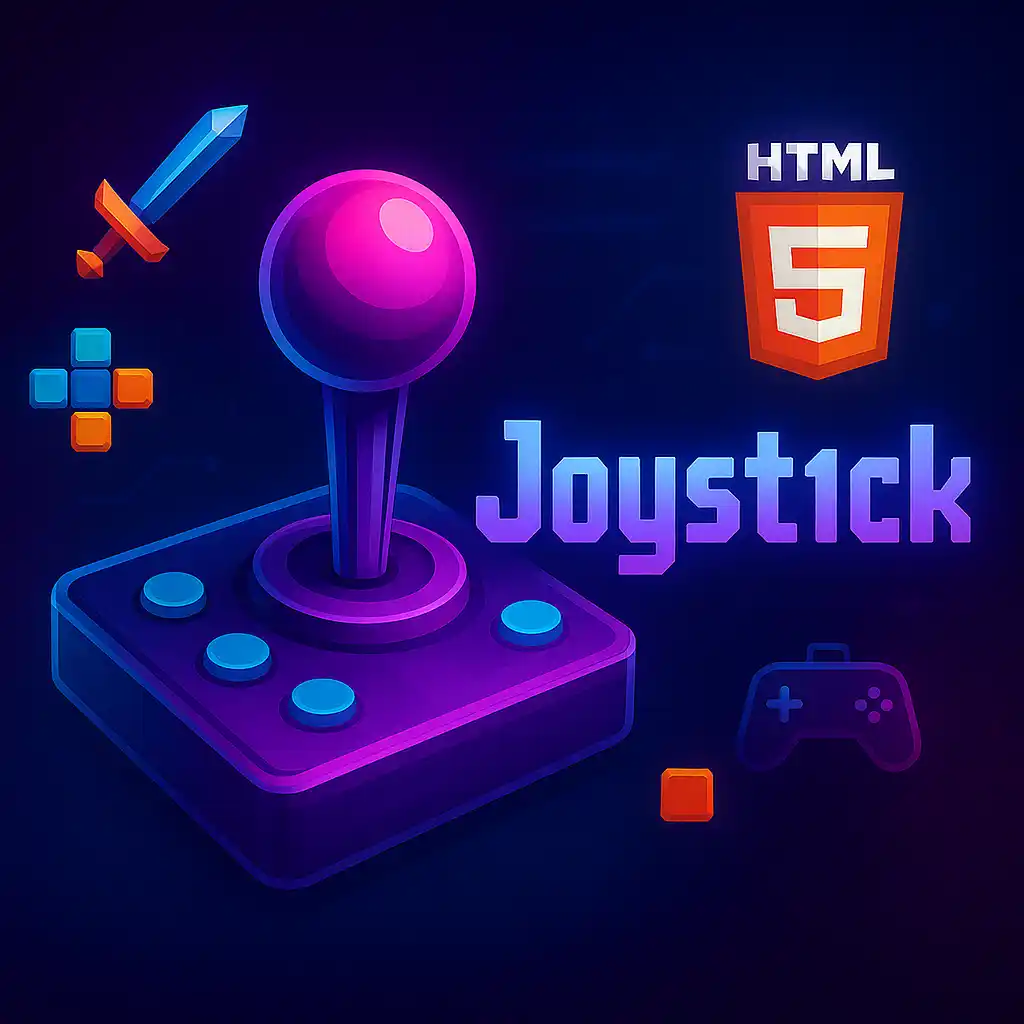