Table of Contents
Rendering a 3D Rectangle in Unity for Enhanced Environment Design
Rendering a 3D rectangle, or a cuboid
, in Unity requires a combination of precise modeling and efficient rendering techniques. Here are key methods to achieve optimal results:
1. Using Unity’s Mesh System
Unity’s built-in meshes allow for fast and efficient creation of 3D objects. You can create a cuboid
by:
Play free games on Playgama.com
- Utilizing the Primitive Objects: Instantly create a 3D rectangle using
GameObject > 3D Object > Cube
and modify its dimensions through the Transform component to fit the desired rectangular shape.
2. Applying Custom Shaders
For enhanced realism, apply custom shaders to your 3D rectangle:
- Standard Shader: Use Unity’s Standard Shader for basic materials and textures, leveraging its PBR capabilities.
- Custom Shader: Write custom shaders in ShaderLab allowing for unique visual effects and optimizations.
Shader "Custom/Rectangle" { Properties { _Color ("Color", Color) = (1,1,1,1) } SubShader { Pass { CGPROGRAM #pragma vertex vert #pragma fragment frag #include "UnityCG.cginc" struct appdata_t { float4 vertex : POSITION; }; struct v2f { float4 pos : SV_POSITION; }; v2f vert (appdata_t v) { v2f o; o.pos = UnityObjectToClipPos(v.vertex); return o; } half4 frag (v2f i) : SV_Target { return half4(1,0,0,1); } ENDCG } } }
3. Lighting and Shadows
Enhance the 3D rectangle’s presence in the environment by optimizing lighting:
- Global Illumination: Utilize Unity’s built-in lighting systems, like baked and real-time global illumination, to provide realistic lighting interactions.
- Shadows: Enable and configure shadows on your light sources to cast realistic shadows from the 3D rectangle onto the surrounding environment.
4. Efficient Rendering Strategies
To ensure performance optimization:
- Utilize Level of Detail (LOD): Implement LOD groups to manage detailed meshes only when necessary based on the camera distance.
- Batch Rendering: Combine multiple 3D rectangles or static geometry using static batching or GPU instancing to reduce draw calls.
5. Texture and Material Optimization
Use detailed textures sparingly and optimize materials:
- Texture Atlasing: Combine multiple textures into a single atlas to minimize texture swaps and boost performance.
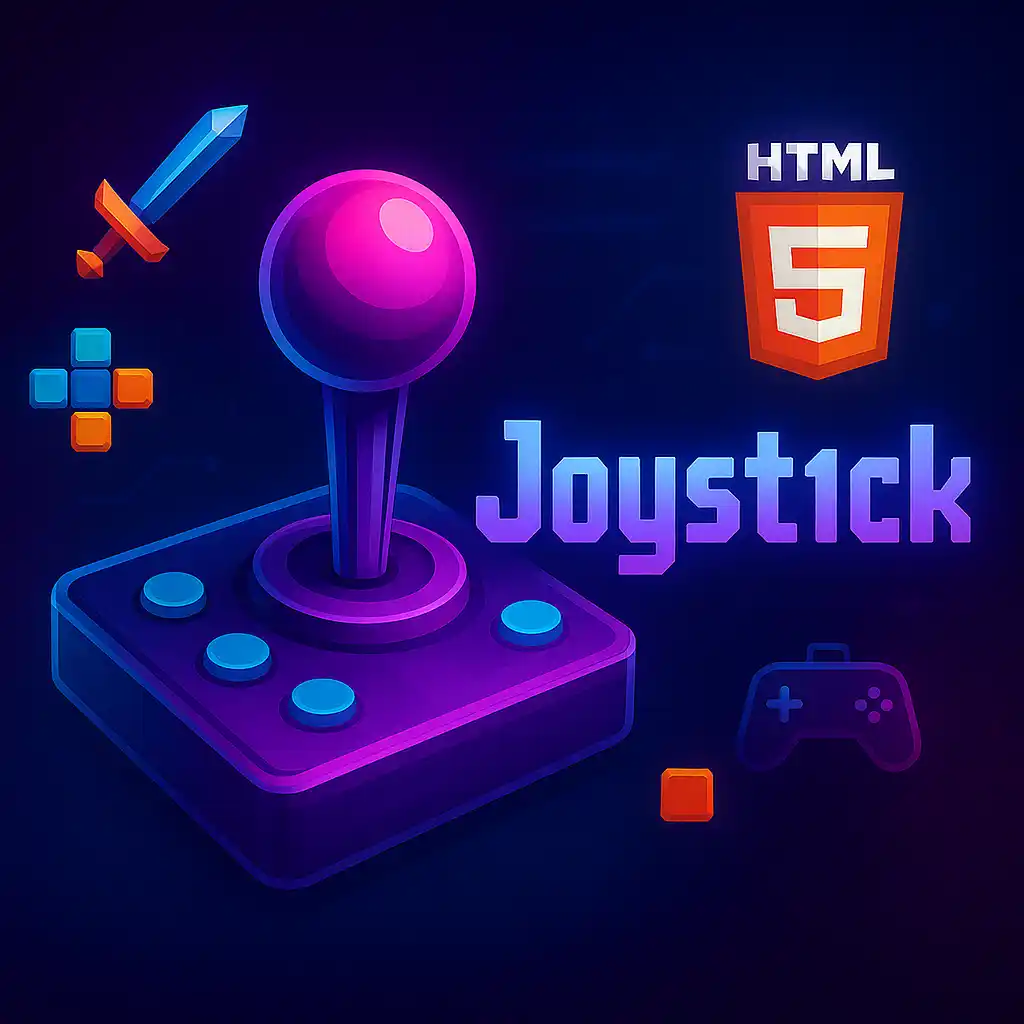