Table of Contents
- Using the ‘int’ Data Type in Game Development Scripting
- Integer Variable Declaration in Game Scripts
- Best Practices for Integer Data Types in Game Development
- Managing Game State with Integer Identifiers
- Performance Implications of Integers in Game Engines
- Example Use Cases
- Integer-Based Indexing in Game Data Retrieval Systems
Using the ‘int’ Data Type in Game Development Scripting
Integer Variable Declaration in Game Scripts
In C# or Python used for game development, the ‘int’ data type is commonly used to declare variables that need to store whole numbers. In C#, this is done simply with int variableName = value;
, which provides a 32-bit integer. Python, being dynamically typed, automatically handles the integer size, so you can just do variableName = value
for any whole number.
Best Practices for Integer Data Types in Game Development
- Consistency: Always use ‘int’ wherever integer operations are expected for clarity and maintainability in your codebase.
- Limits Awareness: Be mindful of the range limitations in C# (approximately -2 billion to +2 billion). Python does not have this issue, but performance can still be impacted by very large numbers.
Managing Game State with Integer Identifiers
‘int’ is often used for entity identification in games, making it easier to manage state and access game objects. For instance, assigning unique IDs to game characters can help in querying their states and managing interactions.
Play free games on Playgama.com
Performance Implications of Integers in Game Engines
Integers are typically more efficient than using floating-point numbers in terms of memory and speed, making them preferable for indexing, counters, and other non-fractional operations in game loops and logic.
Example Use Cases
// C# Example for Entity ID
public class GameEntity {
public int m_ID;
public GameEntity(int id) {
m_ID = id;
}
}
# Python Example for Scoring
player_score = 0
# When player scores
player_score += 10
Integer-Based Indexing in Game Data Retrieval Systems
In data structures used within game engines, integers often serve as offsets or indexes for quick data access, as seen in mesh offsets or buffer indexing.
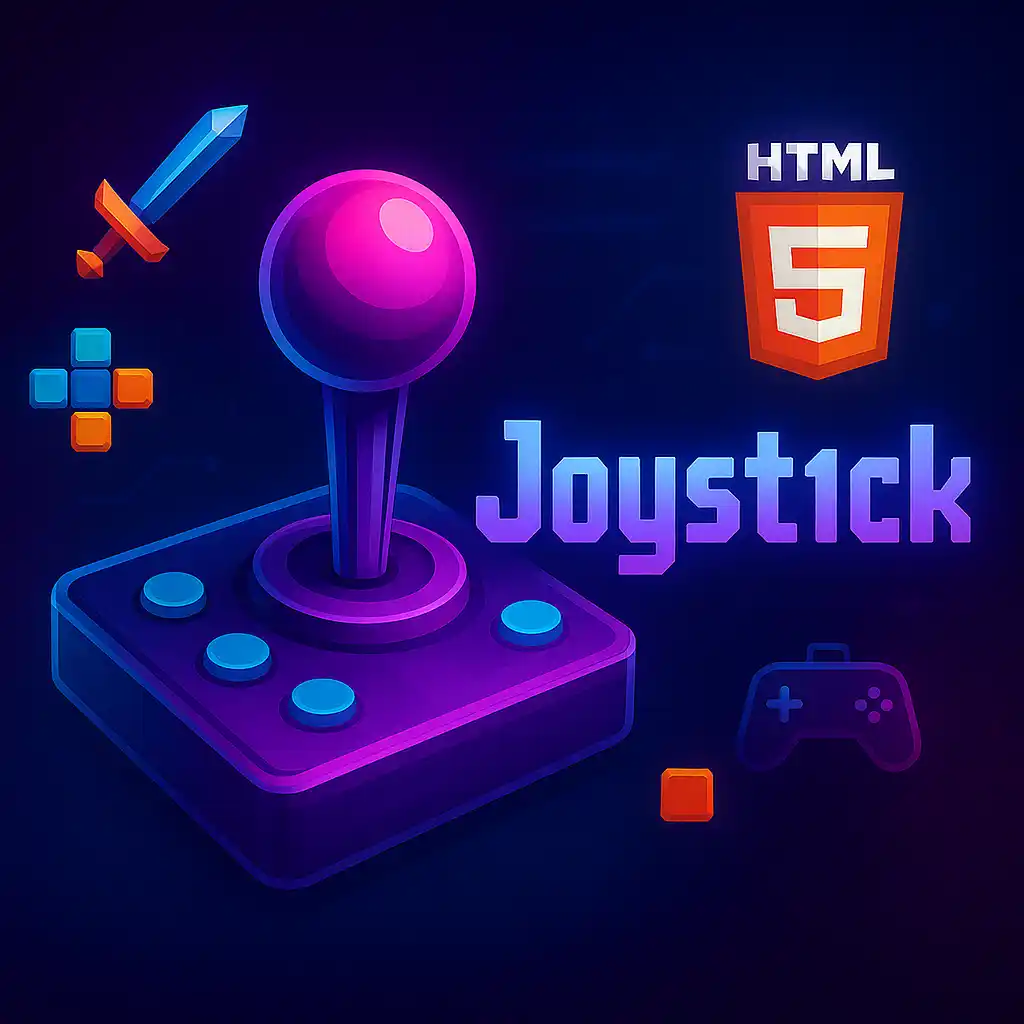