Table of Contents
Calculating a Character’s Movement Speed in a Racing Game
Understanding the Basics of Speed Calculation
In the context of racing games, a character’s movement speed can be defined as the distance they cover over a given time period, following the formula: Speed = Distance / Time
. Utilizing this formula within a game engine involves calculating real-time player movement and translating those movements into measurable speed metrics.
Implementing Speed Calculation in Unity
To implement movement speed calculation within Unity, you’ll need to script the logic using C#. Here’s a basic example:
Play free games on Playgama.com
using UnityEngine;public class SpeedCalculator : MonoBehaviour { private Vector3 lastPosition; public float speed; void Start() { lastPosition = transform.position; } void Update() { Vector3 currentPosition = transform.position; float distance = Vector3.Distance(currentPosition, lastPosition); float deltaTime = Time.deltaTime; speed = distance / deltaTime; lastPosition = currentPosition; }}
This script calculates the distance covered between frames and uses Unity’s Time.deltaTime
to compute the frame-to-frame time lapse, thus updating the speed continuously.
Advanced Considerations
- Vector Mechanics: For more complex movements, consider incorporating vector calculations to align speed with directional movement using
RigidBody.velocity
or manipulatingTransform.forward
withVector3
operations. - Acceleration: Factors like acceleration and drag force can be integrated to simulate more realistic physics using Unity’s physics engine capabilities.
Debugging and Accuracy
To ensure accuracy, deploy video analysis tools to verify the speed calculations align with expected real-world physics. Additionally, using Unity’s debugging tools will allow tracking variable changes in real-time for refinement and optimization.
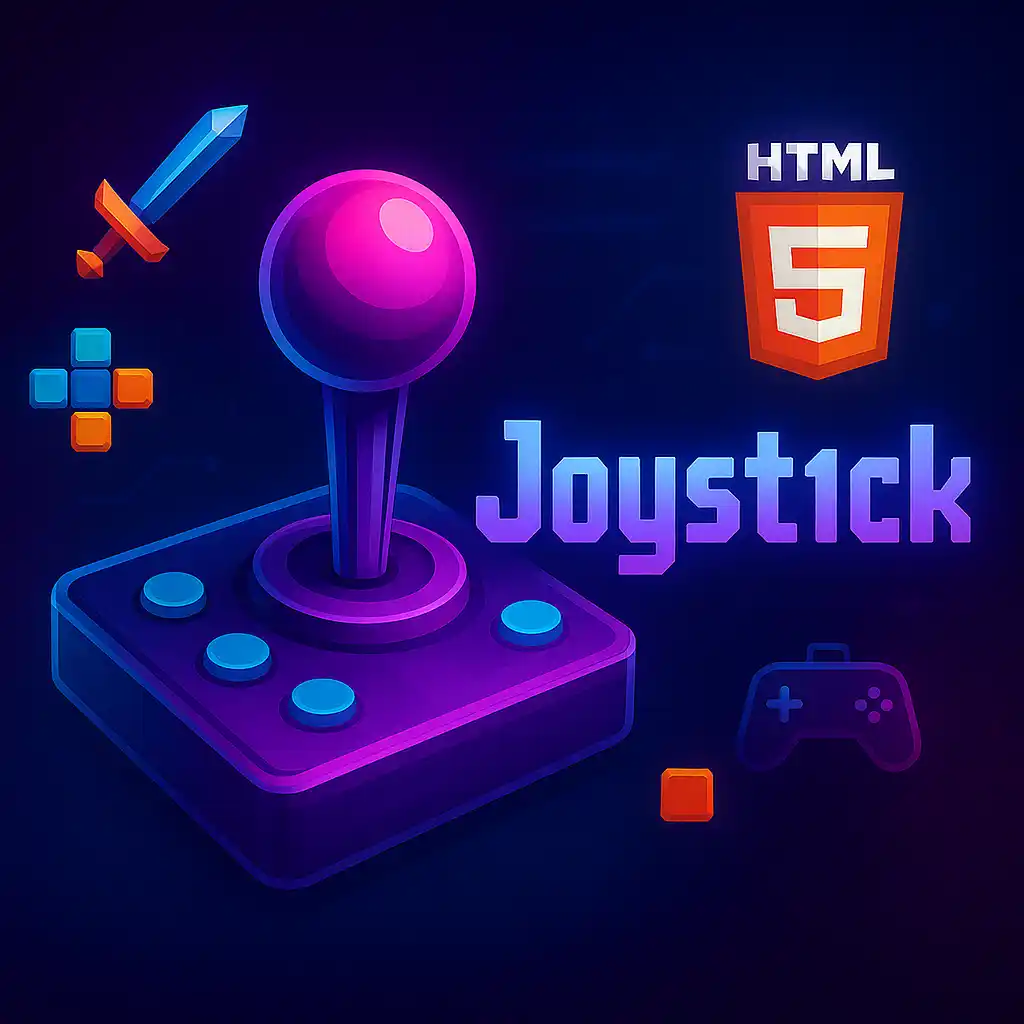