Table of Contents
Implementing Vector Subtraction for Directional Movement in Unity
In Unity, vector subtraction is a fundamental operation to determine the direction one object needs to move to reach another. By subtracting two Vector3 objects, you can calculate the direction vector, which is essential for character movement.
Understanding Vector Subtraction
The subtraction of two vectors A
and B
(i.e., C = A - B
) results in a vector C
pointing from B
to A
. This directional vector is crucial for calculating pathfinding or steering logic in games.
Play free games on Playgama.com
Example Code Snippet
using UnityEngine;
public class DirectionalMovement : MonoBehaviour
{
public Transform target;
void Update()
{
Vector3 direction = target.position - transform.position;
Debug.DrawLine(transform.position, target.position, Color.red);
// Normalize the direction to get a unit vector
Vector3 normalizedDirection = direction.normalized;
// Move towards the target
transform.position += normalizedDirection * Time.deltaTime;
}
}
Practical Applications
- Character Guide: Use this directional vector to create a smooth guiding system for NPCs or characters to move towards targets, interactive objects, or strategic points.
- Camera Movement: Applying vector subtraction helps in calculating paths for dynamic camera movement, ensuring the camera follows or aligns perfectly to a moving object.
Optimizing Vector Operations
While vector subtraction is straightforward, it’s important to be mindful of performance. Use Vector3.Normalize()
instead of direction.normalized
if calling it multiple times to avoid generating garbage. Additionally, consider caching the direction vector if it doesn’t change frequently within a frame to enhance performance.
Conclusion
Vector subtraction in Unity provides a simple yet powerful tool for managing directional movement in game development. By understanding and implementing this operation, developers can create smooth, controlled character and object navigation within their game worlds.
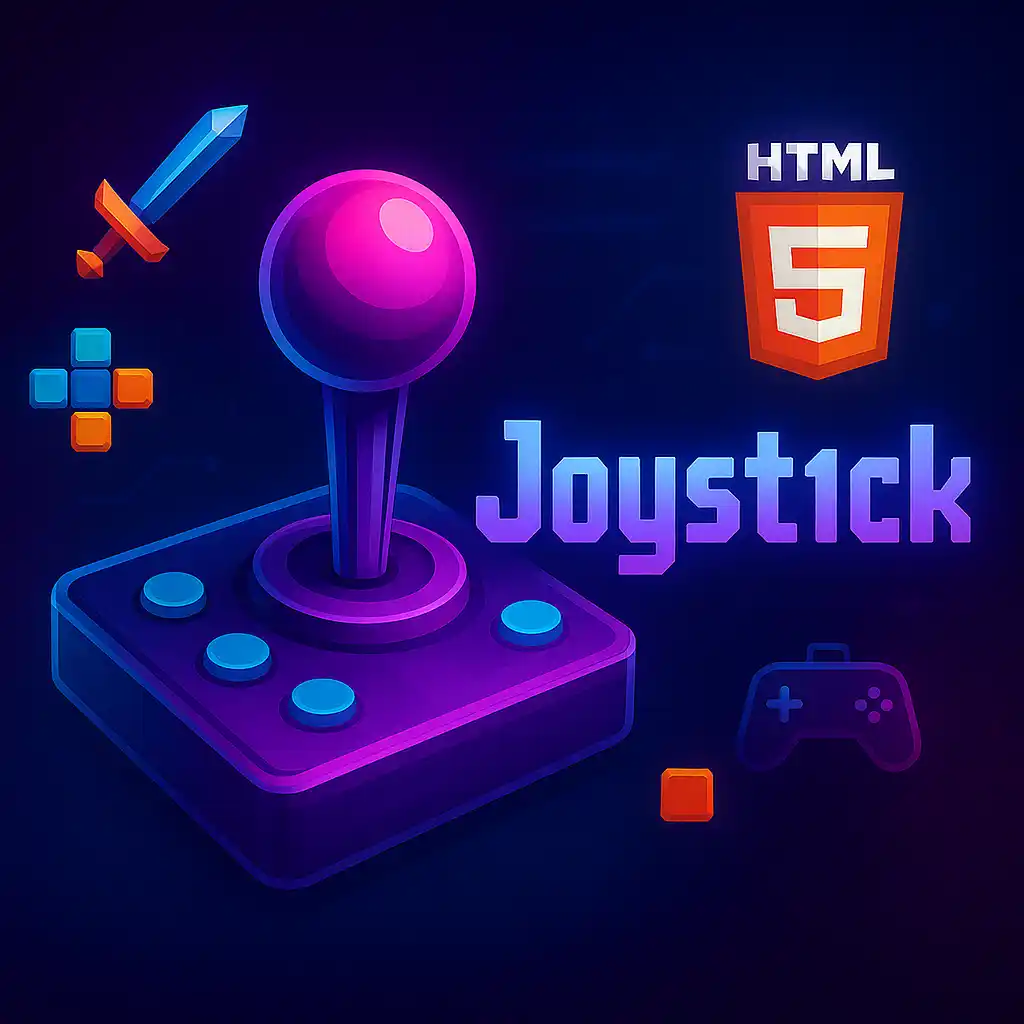