Table of Contents
Implementing AI Behavior for Poker Hands in Unity
Creating intelligent AI for poker games involves simulating human decision-making processes through strategic coding and algorithms. Below are the steps and considerations for implementing AI behavior to evaluate poker hands specifically for making and checking pairs in Unity.
1. Understanding Poker Hand Hierarchy
The basic poker hand hierarchy in terms of pairs includes:
Play free games on Playgama.com
- Single Pair: Two cards of the same rank.
- Two Pair: Two sets of two cards of the same rank.
2. Card Representation
In Unity, represent cards using a class or struct. For example:
public class Card { public int Rank; public char Suit; }
Here, Rank
could be values from 2 to Ace represented as integers, and Suit
as characters.
3. Detecting Pairs in Hand
Utilize a dictionary to track card rank frequencies. Here’s a basic implementation:
Dictionary<int, int> rankCount = new Dictionary<int, int>(); foreach (Card card in hand) { if (rankCount.ContainsKey(card.Rank)) { rankCount[card.Rank]++; } else { rankCount[card.Rank] = 1; } }
Check for pairs:
foreach (var count in rankCount.Values) { if (count == 2) { // Single pair logic } else if (count == 4) { // Two pair logic } }
4. AI Decision-Making Process
Incorporate AI decision-making for pair evaluation using rule-based or probabilistic methods:
- Rule-Based: Define conditions under which to check or make pairs using fixed rules based on game theory.
- Probabilistic Models: Use probability calculations to decide moves depending on the likelihood of winning with current pair configurations.
5. Enhanced AI with Machine Learning
Although simple rule-based systems suffice for basic behavior, incorporating machine learning can simulate more realistic AI decisions. Explore using models trained on historical game data to evaluate pair decisions dynamically.
Conclusion
Implementing AI for checking and making pairs requires a clear understanding of poker mechanics and robust coding within Unity. Leveraging advanced techniques like machine learning can further enhance AI’s competitiveness in poker simulations.
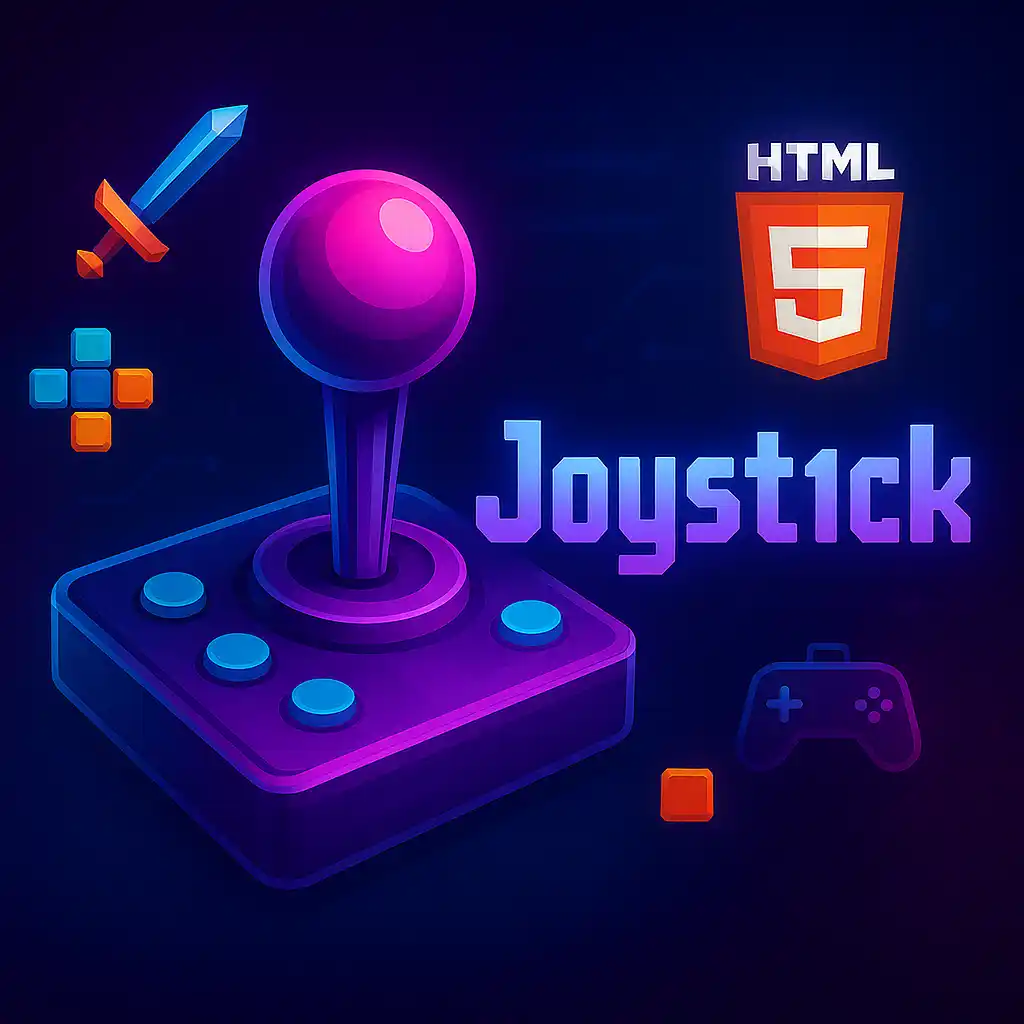