Table of Contents
Implementing a Physics System in Unity
Calculating an object’s velocity from its acceleration involves understanding the fundamental principles of physics and effectively applying them within Unity’s framework. Below, we outline the steps to achieve accurate velocity calculations using Unity’s scripting capabilities.
Understanding the Basics
- Acceleration: The rate of change of velocity of an object. It is usually determined by forces applied to the object and can be represented as a vector.
- Velocity: The speed of an object in a given direction. It is the integral of acceleration over time.
- Time Integration: A numerical method used to update physical quantities over time, crucial for converting an object’s acceleration into velocity.
Core Implementation Steps
- Define Object Properties:
public class PhysicsObject { public Vector3 acceleration; public Vector3 velocity; public float mass; }
- Apply Forces to Determine Acceleration:
public Vector3 CalculateAcceleration(Vector3 force) { return force / mass; }
- Integrate Acceleration to Update Velocity: Using simple integration methods like Euler or more advanced ones like Runge-Kutta:
- Euler Method:
void UpdateVelocity(float deltaTime) { velocity += acceleration * deltaTime; }
- Runge-Kutta Method (for more precision):
void UpdateVelocity(float deltaTime) { Vector3 k1 = acceleration * deltaTime; Vector3 k2 = (acceleration + k1 / 2) * deltaTime; velocity += 0.5f * (k1 + k2); }
- Euler Method:
Realtime Execution
Integrate this process within Unity’s update loop to ensure real-time physics calculations:
Play free games on Playgama.com
void Update() { float deltaTime = Time.deltaTime; acceleration = CalculateAcceleration(appliedForce); UpdateVelocity(deltaTime); transform.position += velocity * deltaTime; }
Advanced Considerations
- Numerical Stability: Ensure that the chosen method for integration provides stable and accurate results, especially when dealing with high-speed objects.
- Performance Optimization: Using fixed time steps (via FixedUpdate) can help achieve more consistent physics simulations.
By following this structured approach, developers can effectively simulate realistic motion dynamics in their Unity projects, enhancing the interactivity and authenticity of the game environment.
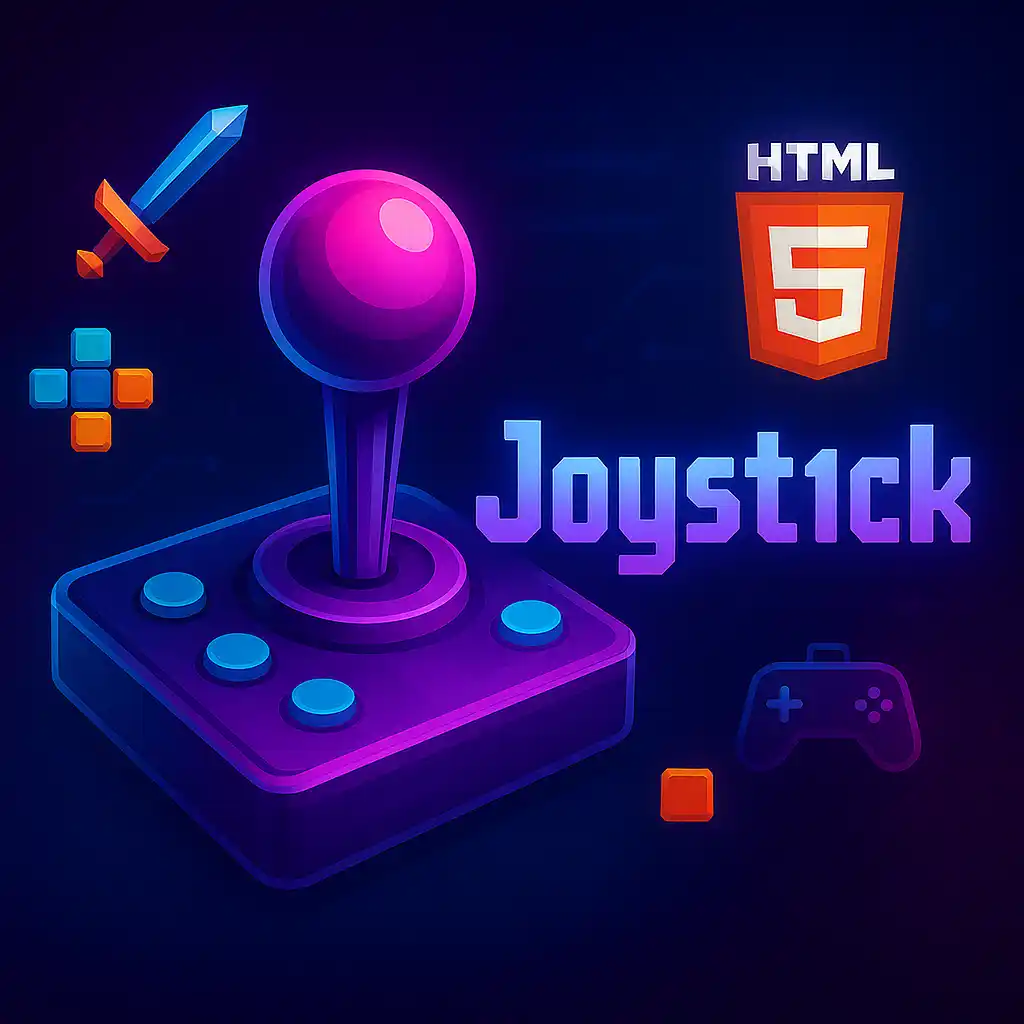