Table of Contents
Integrating Apple Pencil Support in iOS Drawing Applications
Integrating Apple Pencil support into an iOS drawing application requires a deep understanding of the UIKit framework as it is crucial for handling touch input from both fingers and styluses. Here’s a step-by-step approach to achieve optimal Apple Pencil integration:
1. Handling Pencil Input with UIKit
UIKit processes input from Apple Pencil using the UITouch
class. You can differentiate between touches from a finger and the stylus by using the type
property of UITouch
, which specifies the source of the touch.
Play free games on Playgama.com
func touchesBegan(_ touches: Set<UITouch>, with event: UIEvent?) {
for touch in touches {
if touch.type == .pencil {
// Handle Apple Pencil input
}
}
}
2. Implementing Pressure and Tilt Sensitivity
The pressure of the Apple Pencil can be accessed using the force
property of the UITouch
instance. For tilt details, make use of altitudeAngle
and azimuthAngle
:
let force = touch.force / touch.maximumPossibleForce
let tilt = touch.altitudeAngle
let azimuth = touch.azimuthAngle(in: view)
3. Enabling Palm Rejection
Palm rejection can be achieved by filtering out large touches or using Apple’s default handling that ignores non-pencil input when an active Apple Pencil touch is detected. You should ensure the primary view supports multiple touches and properly distinguishes between them, potentially requiring custom logic to filter palm events.
4. Optimizing Drawing Performance
- Reduce Latency: Lower the response time for rendering strokes by optimizing the drawing loop and leveraging iOS’s Metal API for better graphic performance.
- Handle Simultaneous Multitasking: Ensure your application manages multitouch input efficiently while maintaining smooth drawing performance.
5. Testing and Feedback
Consistently test the drawing app using different angles and pressures to ensure that tilt and pressure sensitivity are accurately represented. Encourage user feedback to refine the app’s usability and functionality.
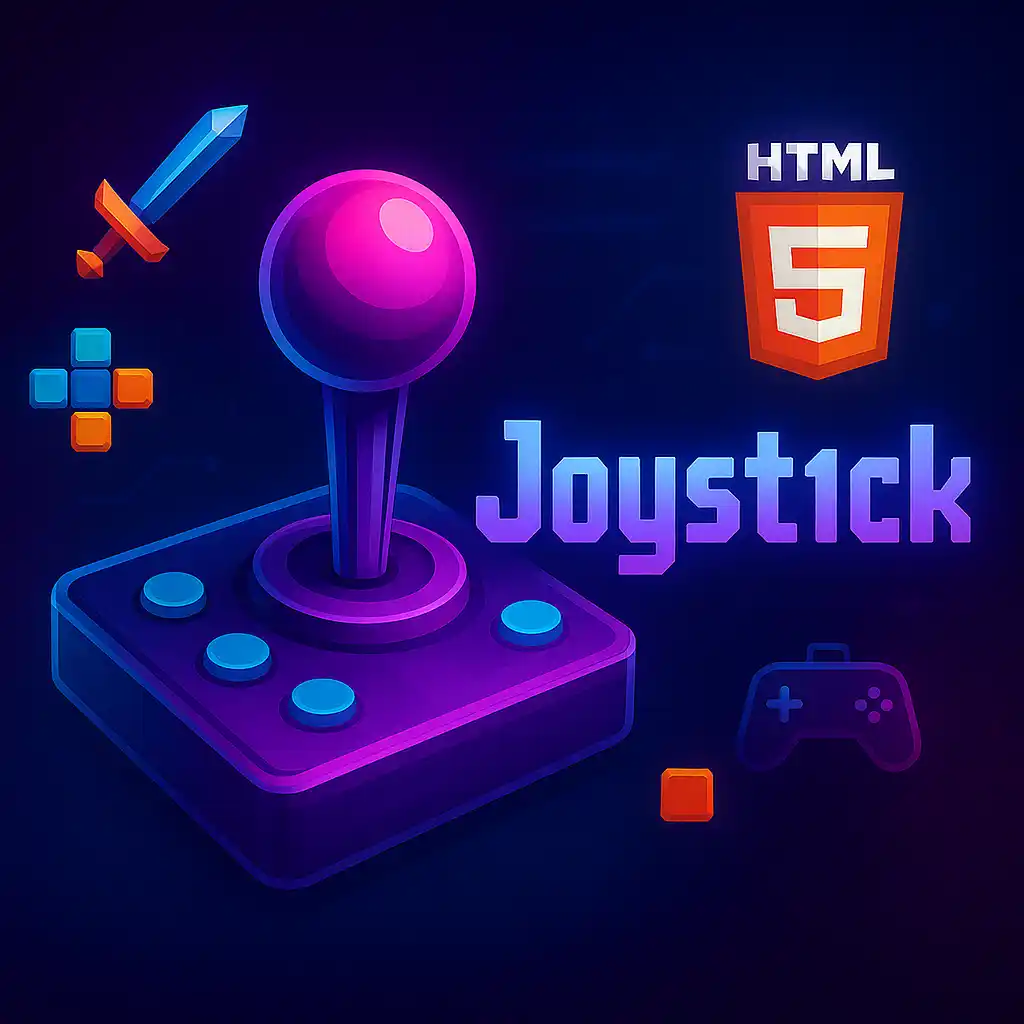