Table of Contents
Checking Waypoint Arrival in Godot
Using Godot’s Built-in Functions
Godot provides several built-in functions to determine when a game character reaches a specific waypoint:
- Distance Checks: One of the simplest methods is to periodically check the distance between the character’s current position and the target waypoint. This can be achieved using the
Vector2/Vector3.distance_to()
function to see if the character is within a small threshold value of the waypoint. - AnimationPlayer Signals: If the movement is driven by animations, you can use signals from the
AnimationPlayer
node to trigger events when the character reaches the waypoint.
Implementing Pathfinding Algorithms
For more complex scenarios involving AI and pathfinding, you might use Godot’s Navigation2D or Navigation3D nodes:
Play free games on Playgama.com
- Path Following: Utilize the Navigation node to generate a path to the waypoint. By monitoring the points along the path, you can tell when the character reaches the desired waypoint.
- Waypoint Verification Techniques: Incorporate checkpoint validation logic within your pathfinding algorithms to confirm arrival at each waypoint in sequence.
Real-Time Monitoring
Use a signal or a callback function attached to the character’s movement update to evaluate whether the waypoint has been reached, ensuring real-time gameplay state monitoring.
Example Code Snippet
extends KinematicBody2D
var target_waypoint
var speed = 200
func _process(delta):
if target_waypoint:
var direction = (target_waypoint - position).normalized()
move_and_slide(direction * speed)
if position.distance_to(target_waypoint) < 5.0:
print("Waypoint reached!")
target_waypoint = null
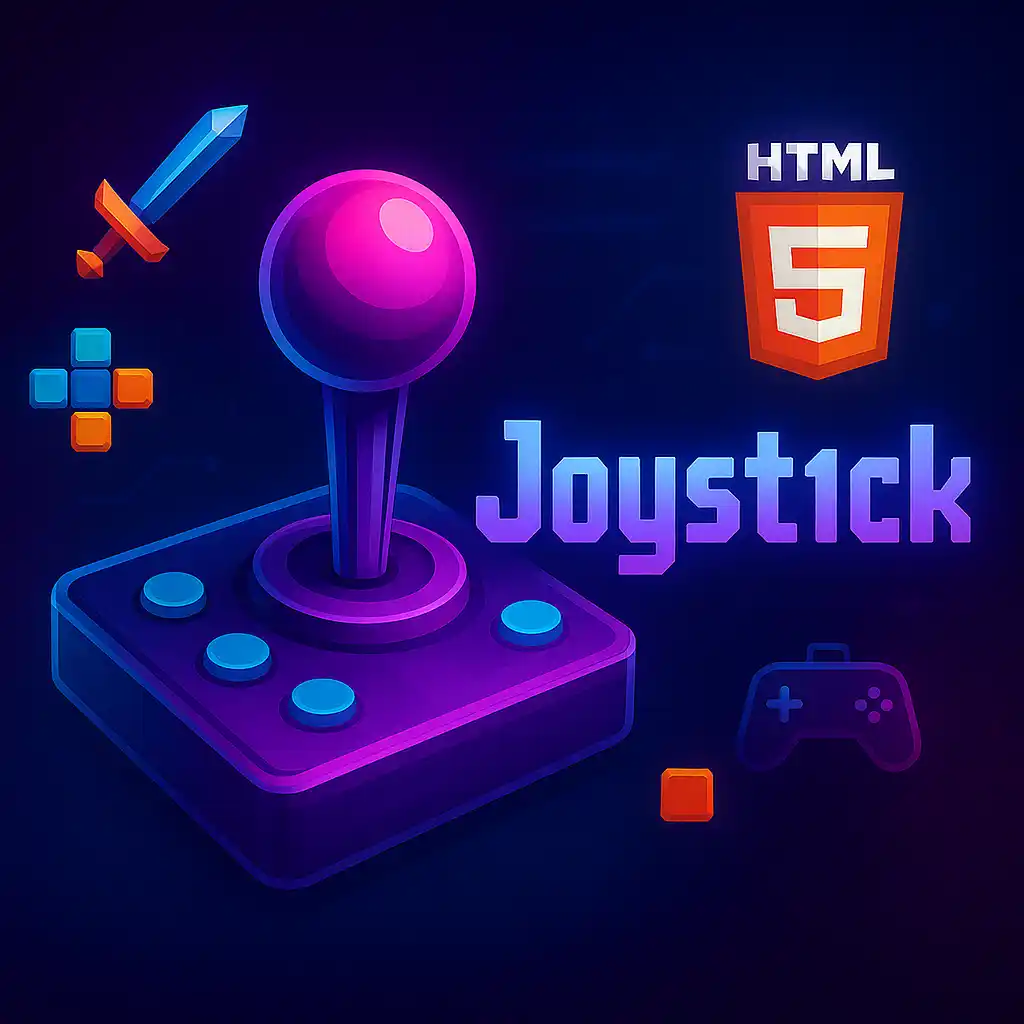