Table of Contents
Implementing a Dynamic Grid System in Godot
Creating a grid system that allows tiles to dynamically transition between rectangular and square shapes in the Godot engine involves several key steps and considerations.
Play free games on Playgama.com
Understanding Tile Movement and Transformations
- Grid Initialization: Start by initializing a grid using a two-dimensional array, where each cell holds properties defining its shape and state.
- Responsive Design: Utilize
GridMap
for a flexible layout that can adjust based on the tile’s shape. Each tile can be represented as an instance of aNode2D
with specific dimensions.
Dynamic Shape Adjustment
- Shape Variables: Define variables to control the width and height of each tile, allowing for dynamic changes. Example:
var tile_width = 64
var tile_height = 64
- Transformation Logic: Implement logic to modify these dimensions based on certain triggers or game events using GDScript:
func change_tile_shape(new_width, new_height):
tile_width = new_width
tile_height = new_height
for tile in grid:
tile.set_size(Vector2(tile_width, tile_height))
Rendering and Optimization
- Efficient Rendering: Optimize rendering by redrawing only the affected tiles when a change occurs. Utilize signals to detect when a tile’s shape or position is updated.
- Tilemap Optimization: Use Godot’s
TileMap
node to efficiently handle large grids and dynamic tile updates. Consider rendering only visible tiles usingculling
techniques to reduce performance overhead.
Advanced Grid Techniques
- Transformational Geometry: Apply mathematical transformations for more complex tile shape modifications. Leverage transformational geometry techniques to manage complex tile operations.
- Adaptive Grids: Implement adaptive grid logic to handle different device aspect ratios and resolutions efficiently, ensuring consistent tile behavior across platforms.
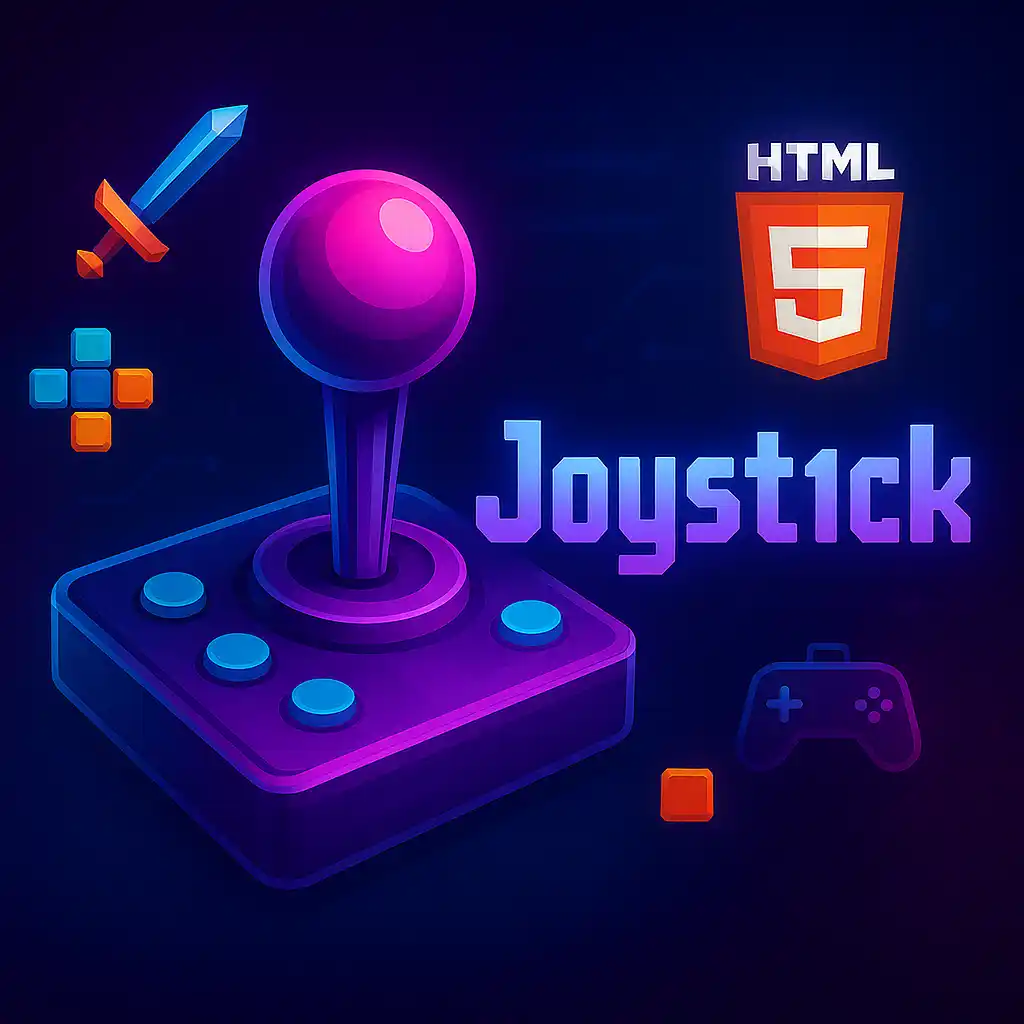