Table of Contents
Implementing 90-Degree Camera Rotation in Unreal Engine
Understanding Camera Components
Unreal Engine uses APlayerCameraManager
and ACameraActor
classes to control camera properties. To rotate the camera by 90 degrees, you must adjust these components effectively.
Step-by-Step Implementation
- Set Up Camera Reference:
ACameraActor* MyCamera = GetWorld()->SpawnActor
(ACameraActor::StaticClass()); - Define Rotation Parameters: Use the
FRotator
to specify the angle.FRotator NewRotation = FRotator(0.0f, 90.0f, 0.0f);
- Apply the Rotation: Adjust the camera’s rotation during a transition.
MyCamera->SetActorRotation(NewRotation);
- Smooth Transition:
Implement a blend for smoother transitions using Timeline or Tweening utilities in UE.float BlendTime = 1.0f; PlayerCameraManager->SetViewTargetWithBlend(MyCamera, BlendTime);
Best Practices
- Testing: Regularly test the transitions in various scenes to ensure optimal performance and visual alignment.
- Performance: Consider the impact on performance when implementing multiple transitions or in complex scenes.
Additional Resources
For further insights, refer to Unreal Engine’s official documentation on camera handling and rotation techniques.
Play free games on Playgama.com
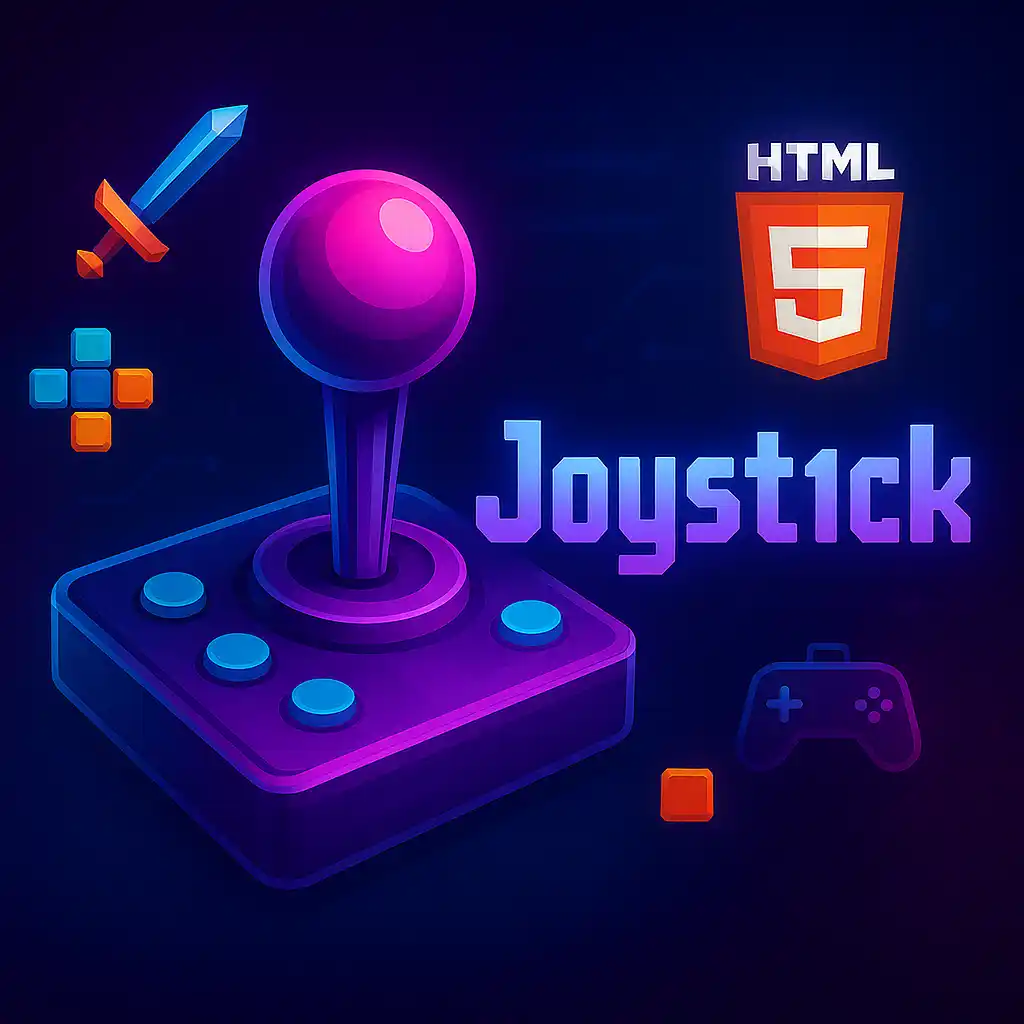