Table of Contents
Creating a Realistic Bow and Arrow Physics System in Unity
Understanding Bow Mechanics
To simulate a realistic bow and arrow physics system, it’s essential to understand the mechanics involved. A bow stores potential energy, which is transferred to the arrow as kinetic energy when released. The force applied to the arrow is dependent on the bowstring tension and the draw length.
Implementing Arrow Flight Dynamics
Realistic arrow flight dynamics can be achieved by considering physical forces such as gravity, drag, and lift. Use Unity’s Rigidbody component to handle these physics:
Play free games on Playgama.com
Rigidbody arrowRigidbody = arrowGameObject.GetComponent<Rigidbody>();
arrowRigidbody.useGravity = true;
arrowRigidbody.drag = 0.1f; // Adjust based on air resistance
Programming the Release Mechanism
To simulate the release of the bowstring and arrow launch, use the Unity physics engine to apply force:
float drawStrength = 50f; // Example force value
direction = (targetPosition - bowPosition).normalized;
arrowRigidbody.AddForce(direction * drawStrength, ForceMode.Impulse);
Enhancing Realism with Animation
A realistic bow and arrow system is complemented by animations that show the bowstring tension increasing and releasing. Use Unity’s Animator component to create blend shapes or skeletal animations for a more immersive effect.
Iterative Testing and Refinement
Game development stages require thorough testing and refinement. Use Unity’s Play Mode and Physics Debugging tools to iterate on bow strength, arrow mass, and damping values to ensure realism and engaging mechanics.
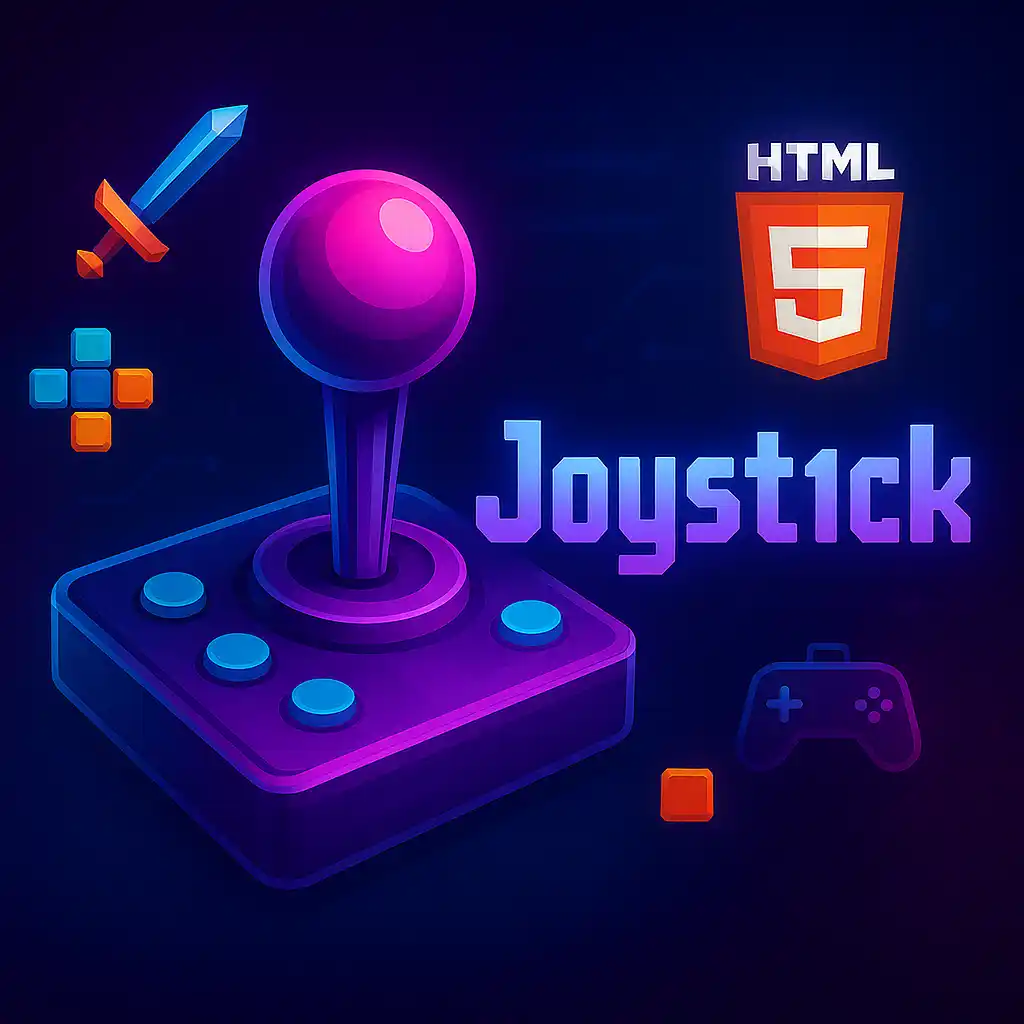