Table of Contents
Mechanics and Physics Calculations for a Long Jump Action in a Platformer Game
Understanding the Basics of Long Jump Mechanics
The long jump in a platformer like Super Mario 64 combines horizontal and vertical motion, allowing for precise control over the character’s trajectory. Key components include initial velocity, angle of jump, and gravitational force to simulate realistic movement.
Initial Setup
- Initial Velocity Calculation: The character’s speed when the jump is initiated affects how far they can go. This can be adjusted dynamically based on player speed inputs.
- Jump Angle: Typically set between 30 to 45 degrees; a critical factor in determining the height and distance of the jump. The angle can be influenced by in-game mechanics like power-ups or player control.
Physics Calculations
function calculateLongJump(initialSpeed, jumpAngle) {
const gravity = -9.8; // Earth's gravity constant in m/s^2
const angleInRadians = jumpAngle * (Math.PI / 180);
const horizontalSpeed = initialSpeed * Math.cos(angleInRadians);
const verticalSpeed = initialSpeed * Math.sin(angleInRadians);
let timeOfFlight = (2 * verticalSpeed) / -gravity;
let jumpDistance = horizontalSpeed * timeOfFlight;
return { timeOfFlight, jumpDistance };
}
Implementing in Unity
Use Unity’s Rigidbody component to apply calculated forces and ensure realistic physics interactions. Adjust the physics material properties to tweak friction and bounce for better control.
Play free games on Playgama.com
Rigidbody rb;
void Start() {
rb = GetComponent<Rigidbody>();
}
void LongJump(float initialSpeed, float jumpAngle) {
float radians = jumpAngle * Mathf.Deg2Rad;
Vector3 jumpForce = new Vector3(initialSpeed * Mathf.Cos(radians), initialSpeed * Mathf.Sin(radians), 0);
rb.AddForce(jumpForce, ForceMode.VelocityChange);
}
Tuning Player Experience
- Jump Adjustments: Use player feedback to refine jump height and distance, ensuring a fun and responsive experience.
- Animation Integration: Sync the physical jump actions with the character animations for smooth transitions and realistic appearances.
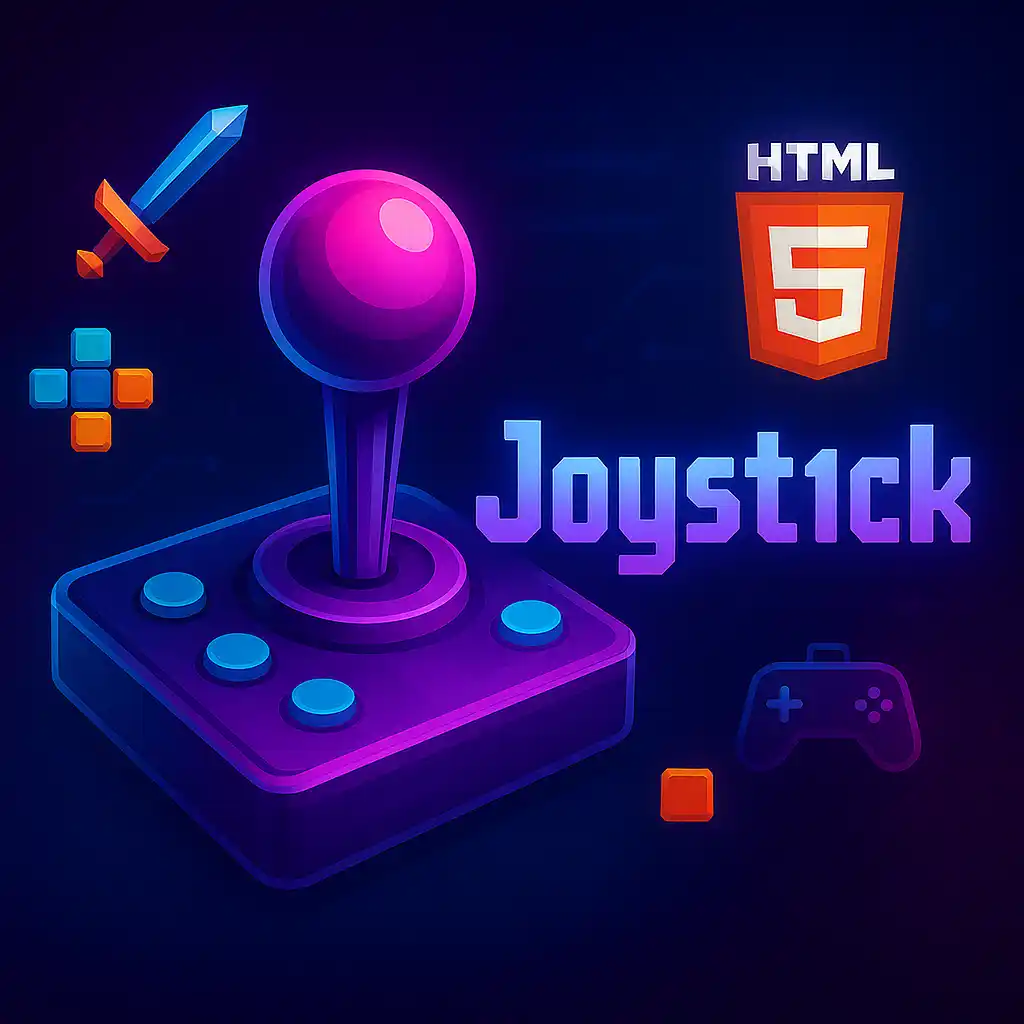