Table of Contents
Programmatically Drawing a Square in Unity
To draw a square programmatically in Unity, developers often opt for procedural mesh creation or using Unity’s inbuilt UI system. Below, I will detail both methods to demonstrate how to effectively generate a square at runtime.
Method 1: Procedural Mesh Creation
The procedural mesh method provides flexibility in rendering and is often used in scenarios requiring dynamic shape manipulation. Here’s a step-by-step implementation:
Play free games on Playgama.com
using UnityEngine;
public class DrawSquare : MonoBehaviour {
void Start() {
GameObject square = new GameObject("Square");
MeshFilter meshFilter = square.AddComponent<MeshFilter>();
MeshRenderer meshRenderer = square.AddComponent<MeshRenderer>();
Mesh mesh = new Mesh();
mesh.vertices = new Vector3[] {
new Vector3(0, 0, 0),
new Vector3(1, 0, 0),
new Vector3(0, 1, 0),
new Vector3(1, 1, 0)
};
mesh.triangles = new int[] { 0, 2, 1, 2, 3, 1 };
mesh.RecalculateNormals();
meshFilter.mesh = mesh;
}
}
This script creates a new GameObject with a MeshFilter and MeshRenderer. It defines vertices and triangles to form a square, and feeds this data to the Mesh object, rendering it on the screen.
Method 2: Using Unity’s UI System
Unity’s UI system provides a more straightforward approach for 2D games or game user interfaces:
- In the Unity Editor, create a new Canvas and attach an empty UI Image component.
- Set the Image’s ‘Sprite’ property to a default square sprite (or any square sprite you import).
- Programmatically manipulate its position and size through script as needed:
using UnityEngine;
using UnityEngine.UI;
public class DrawUISquare : MonoBehaviour {
public Image squareImage;
void Start() {
if (squareImage == null) return;
squareImage.rectTransform.sizeDelta = new Vector2(100, 100); // Set size
squareImage.color = Color.red; // Set color
}
}
This method leverages Unity’s UI components for simpler implementation scenarios focused on fixed-scale and position-independent squares.
Conclusion
The choice between procedural meshes and UI-based methods depends largely on the game’s requirements. Use procedural meshes for dynamic, transformable objects, and Unity’s UI for static interfaces or simple 2D games. Incorporating proper methods enhances both flexibility and efficiency in game development projects.
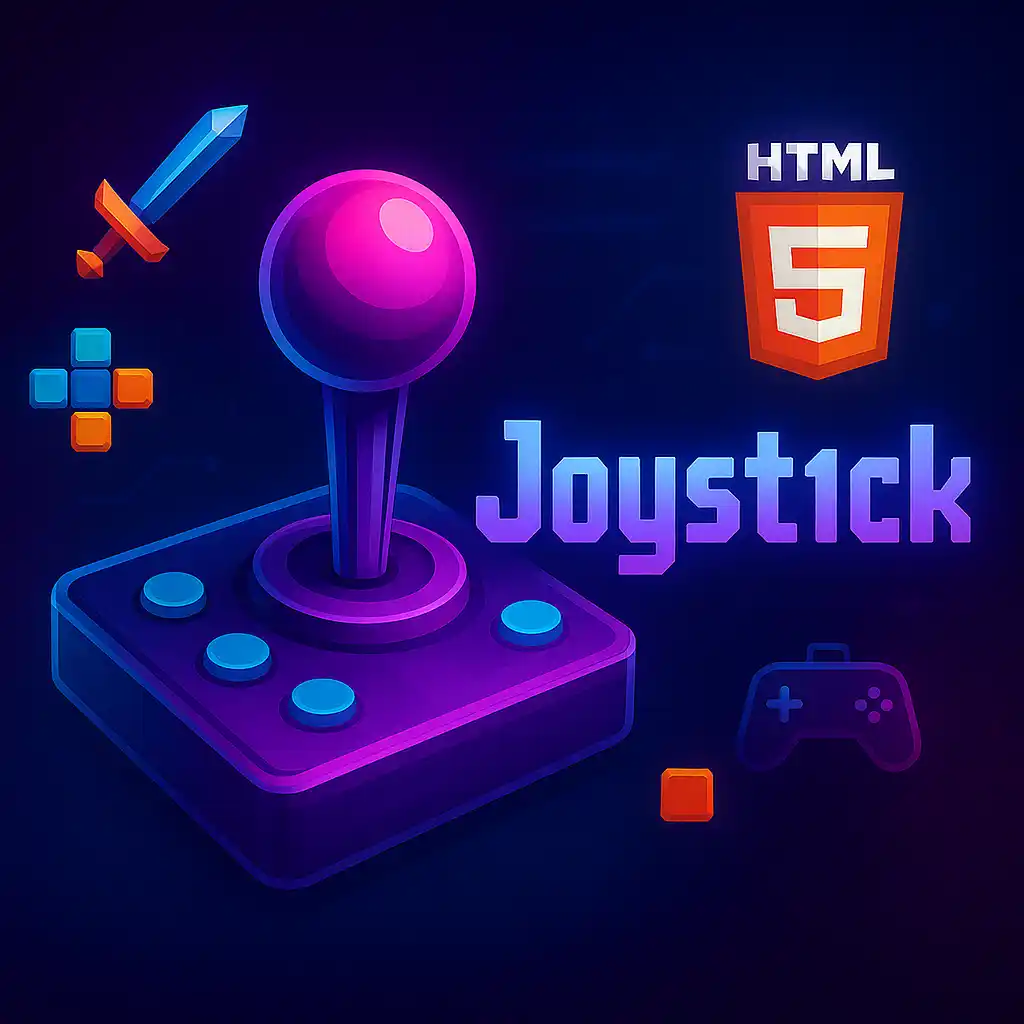