Table of Contents
Implementing a Minimize Feature in Unity
Overview
Implementing a minimize feature in a Unity PC game involves utilizing platform-specific libraries and Unity scripting to control the game window’s state. This ensures users can minimize the game window to the taskbar using a custom UI or system shortcut.
Step-by-Step Implementation
1. Using Windows Platform Specific Code
To control window states on a Windows platform, the User32.dll
library is essential. It allows manipulation of windows directly from C# scripts. Below is an example of how to use it:
Play free games on Playgama.com
using UnityEngine;
using System.Runtime.InteropServices;
public class WindowController : MonoBehaviour
{
[DllImport("user32.dll")]
private static extern int ShowWindow(System.IntPtr hWnd, int nCmdShow);
[DllImport("user32.dll")]
private static extern System.IntPtr GetForegroundWindow();
private const int SW_MINIMIZE = 6;
public void MinimizeWindow()
{
ShowWindow(GetForegroundWindow(), SW_MINIMIZE);
}
}
2. Integrating Unity UI
Create a button in your Unity UI that triggers the MinimizeWindow
method. This can be done by attaching the WindowController
script to a GameObject and linking the button’s OnClick
event:
- In the Unity Editor, create a new
Button
via theGameObject > UI > Button
. - Attach the
WindowController
script to a GameObject in your scene. - Link the button’s
OnClick
event to theMinimizeWindow
method.
3. Handling Platform Differences
Ensure your solution only runs on compatible platforms by using platform-dependent compilation flags:
#if UNITY_STANDALONE_WIN
// Windows-specific code here.
#endif
Best Practices
- Maintain Code Modularity: Separate your window handling logic from game logic to ensure cleaner code structure.
- Cross-Platform Considerations: Evaluate if similar features apply for MacOS or Linux when developing cross-platform games.
- Test Thoroughly: Ensure the minimize feature interacts smoothly with other UI elements and functionalities. Test edge cases like repeated minimize/maximize actions.
Conclusion
Integrating a minimize feature enhances user experience by aligning with standard desktop software behavior. Through proper library usage and Unity’s powerful scripting capabilities, developers can ensure seamless window state management.
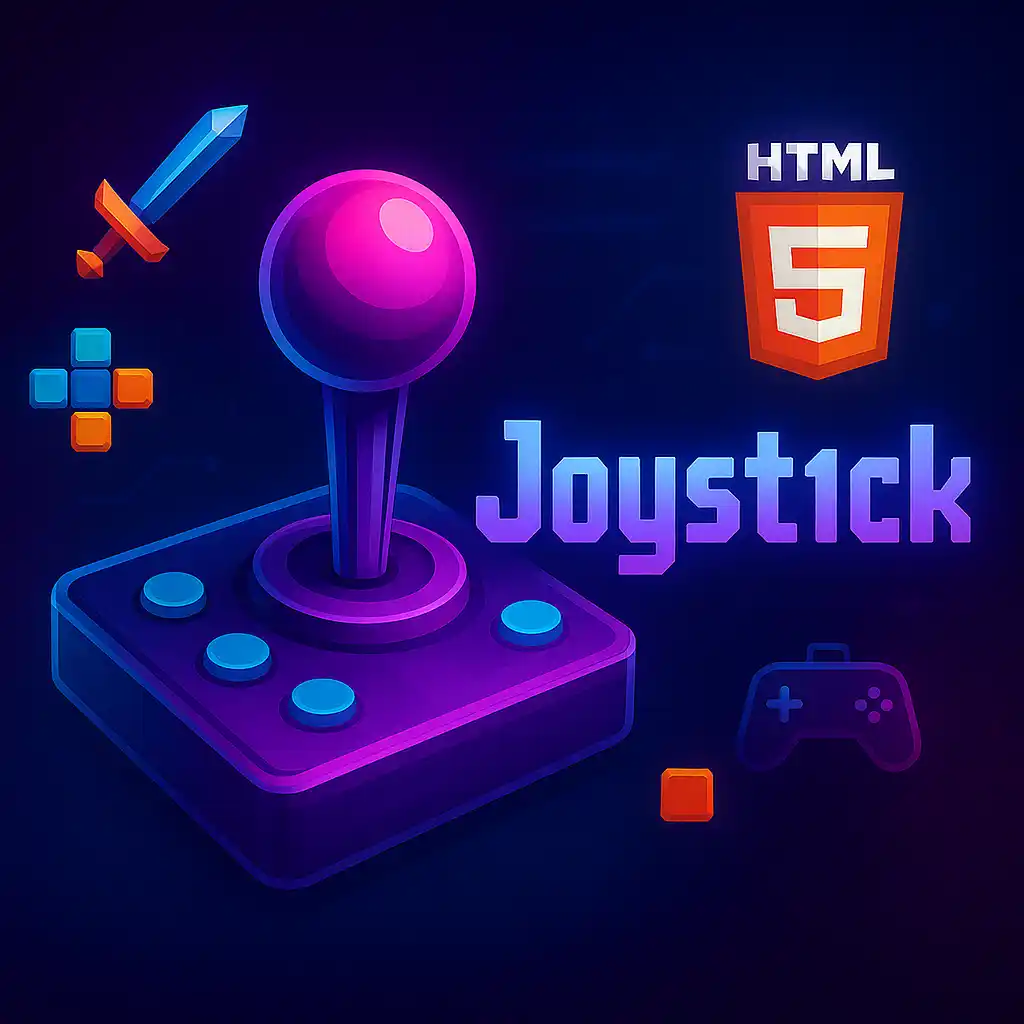