Table of Contents
Implementing an AI Behavior System for Enemy Attack Notifications in Unity
To successfully implement an AI behavior system that notifies players when an enemy is about to attack in an RPG game, you must consider several core components, including AI behavioral patterns, notification systems, and integration mechanics. Below is a step-by-step guide to developing this system in Unity.
Step 1: Designing Enemy AI Behavior Patterns
- State Machine Implementation: Use a state machine to handle different states such as patrolling, chasing, and attacking. Unity’s State Machine Behaviours can be leveraged to manage these states effectively.
- Attack Prediction Logic: Create a predictive logic that determines when an enemy will attack. This can be based on proximity to the player or a countdown timer after the player enters a specific zone.
Step 2: Player Notification System
- UI Alerts: Implement a UI system that uses visual indicators (e.g., icons or HUD elements) when an attack is imminent. Unity’s UI Canvas can be used to dynamically display these elements.
- Sound Cues: Utilize sound cues to alert players of incoming attacks. Attach audio sources to enemy objects and trigger these sounds based on enemy states.
Step 3: Integration of Real-Time Alerts
- Event-Driven Architecture: Use C# events to broadcast and listen for attack notifications, allowing real-time updates to the player’s UI and audio systems.
- Optimization: Ensure the system performs efficiently even with multiple enemies. Utilize Unity’s Profiler to identify bottlenecks and optimize your performance accordingly.
Example Code
public class EnemyAI : MonoBehaviour {
public event Action OnAttackPrepared;
private float attackCooldown = 5f;
private float lastAttackTime;
void Update() {
if (Time.time - lastAttackTime > attackCooldown) {
PrepareAttack();
lastAttackTime = Time.time;
}
}
private void PrepareAttack() {
// Logic to prepare attack
OnAttackPrepared?.Invoke();
}
}
This code snippet sets up an event-based system kicking off notifications when an enemy is set to attack. Adjust the cooldown and conditions based on AI design specifics.
Play free games on Playgama.com
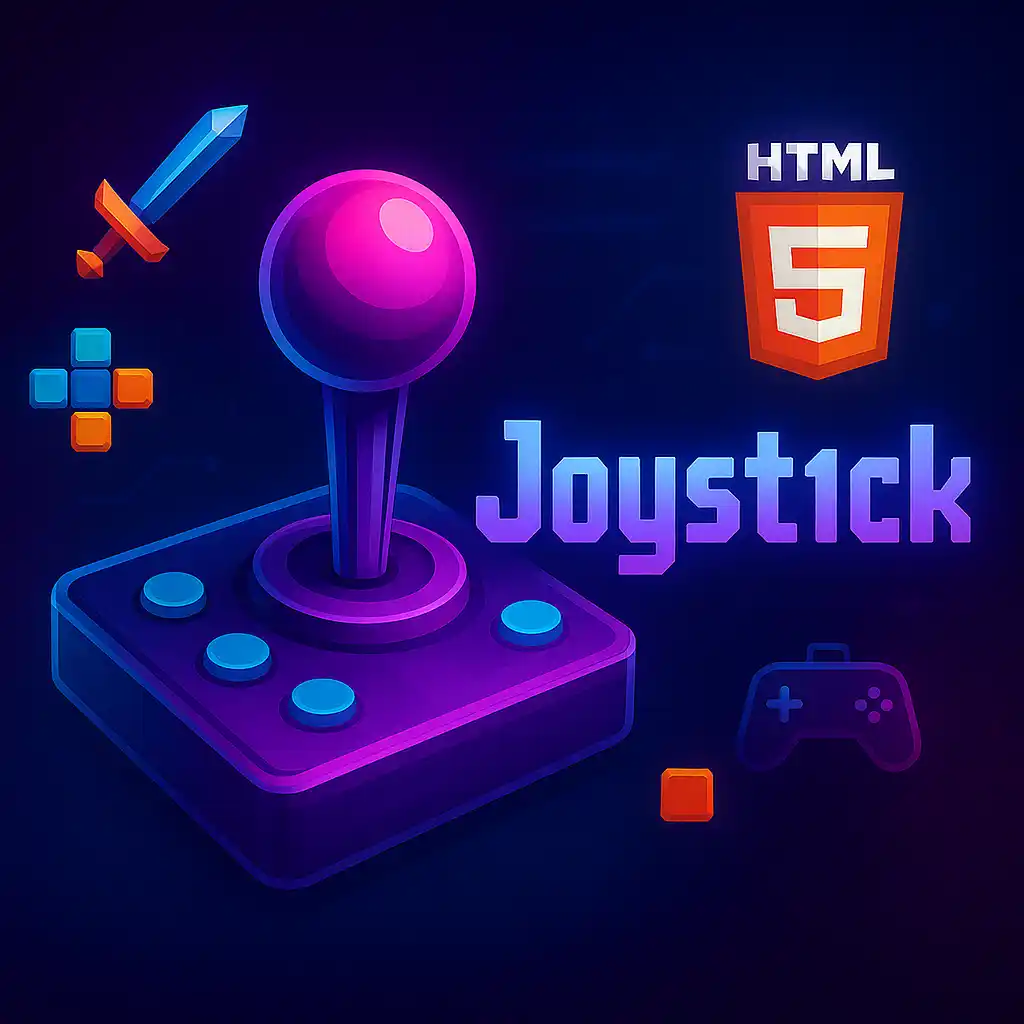