Table of Contents
Determining Vector Direction in Unity for Realistic Character Physics
In game development, especially when using Unity, determining the direction of a vector is crucial for implementing realistic physics-based character movement. A vector’s direction can be computed using its normalized form. This provides a unit vector that maintains the original vector’s direction.
Calculating a Normalized Vector
To find the normalized vector, you divide each component of the vector by its magnitude. Here’s a simple C# code snippet for Unity:
Play free games on Playgama.com
Vector3 direction = new Vector3(x, y, z);
float magnitude = direction.magnitude;
Vector3 normalizedDirection = direction / magnitude;
Using Vector Direction in Character Movement
Once you have the normalized direction, it’s useful for realistic character movement calculations:
- Apply Forces: Use the direction vector to apply forces in physics calculations, such as using
Rigidbody.AddForce()
for movement. - Rotation: Align the character’s orientation with the movement vector using functions like
Quaternion.LookRotation()
. - Collision Detection: Direction vectors can help predict and respond to collisions by projecting future positions.
Practical Example
Consider a scenario where you are implementing a simple forward movement system:
public class PlayerMovement : MonoBehaviour {
public float speed = 5.0f;
private Rigidbody rb;
void Start() {
rb = GetComponent<Rigidbody>();
}
void Update() {
// Get input and create vector
float moveHorizontal = Input.GetAxis("Horizontal");
float moveVertical = Input.GetAxis("Vertical");
Vector3 movement = new Vector3(moveHorizontal, 0.0f, moveVertical);
// Normalize vector to get direction
if (movement != Vector3.zero) {
Vector3 direction = movement.normalized;
rb.AddForce(direction * speed);
}
}
}
This script relies on vector direction to adjust the Rigidbody’s position according to player input, ensuring movement is smooth and realistic.
Conclusion
Understanding and utilizing vector direction is vital in developing realistic physics-based movement systems in Unity. Employ the strategies and code samples provided to enhance your game’s dynamics and realism.
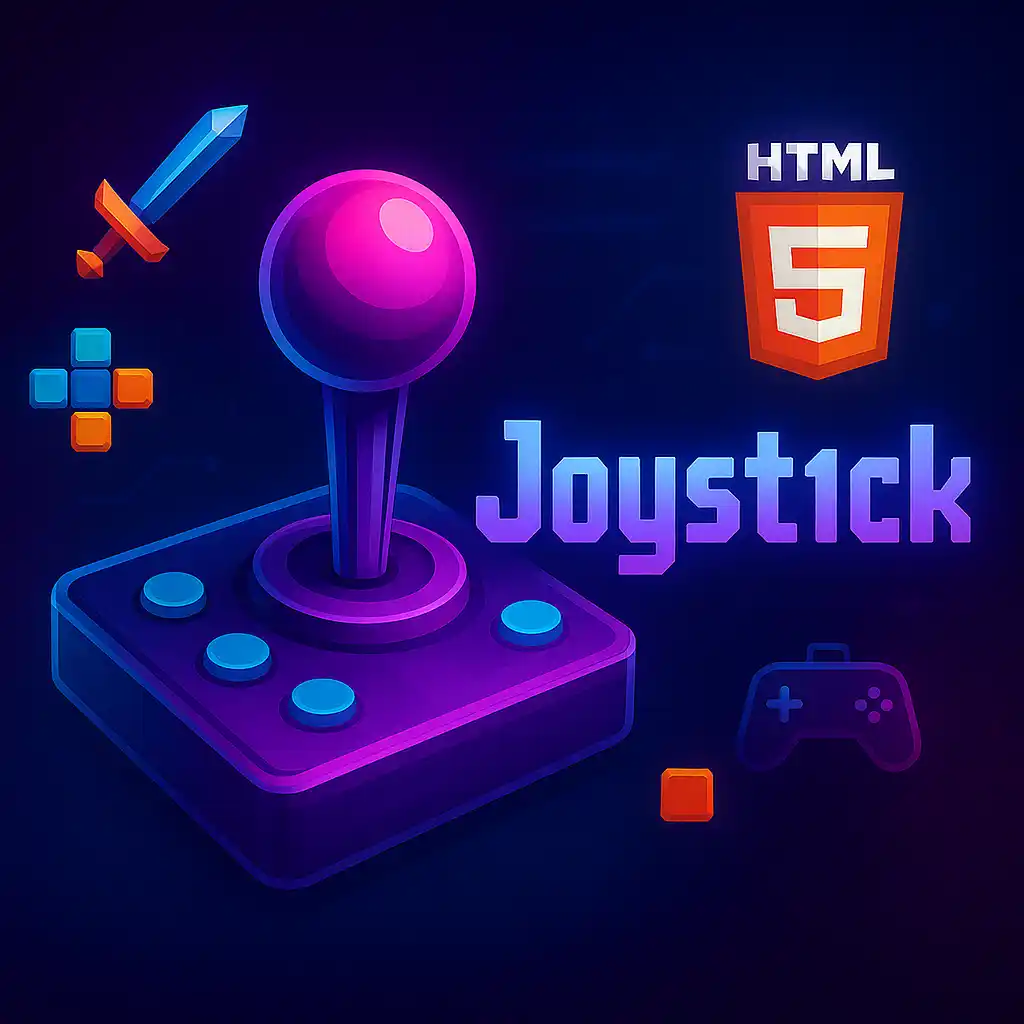