Table of Contents
Creating an Infinite Scrolling Background in Unity
To create an infinite scrolling background in a 2D game using Unity, you can leverage procedural generation techniques. This approach enables dynamic and seamless environments, enhancing immersion and reducing manual workload. Below is a step-by-step guide on setting it up:
Step 1: Set Up Your Assets
- Sprites: Prepare your textures or sprites to be used as background tiles. Ensure they tile seamlessly to avoid visible edges.
- Prefab Creation: Convert your tile sprite into a prefab for easy instantiation.
Step 2: Implement Scrolling Logic
Create a script to handle the background scrolling:
Play free games on Playgama.com
using UnityEngine;
public class InfiniteScroll : MonoBehaviour {
public float scrollSpeed = 2.0f;
private Vector3 startPosition;
private float tileSize;
void Start() {
startPosition = transform.position;
// Assuming the sprite is full width of the camera view
tileSize = GetComponent<Renderer>().bounds.size.x;
}
void Update() {
float newPosition = Mathf.Repeat(Time.time * -scrollSpeed, tileSize);
transform.position = startPosition + Vector3.right * newPosition;
}
}
Step 3: Repeat and Extend
To achieve true infinite scrolling, duplicate your background prefab along the x-axis at intervals equal to the tile size. Continuously reposition these tiles as they move out of view using a cyclical logic.
Step 4: Optimize Performance
- Use Background Layers: Split your background into multiple layers with varied scroll speeds (parallax effect).
- Pooling Strategy: Use object pooling to recycle background tiles for efficient performance and reduced overhead.
Additional Tips
- Leverage Unity’s built-in features like the ‘Order in Layer’ to manage overlapping tiles.
- Tweak the scroll speed and tile sizes for a smooth and appealing visual effect.
These steps incorporate procedural content generation principles to create engaging, algorithm-driven scenery loops. Such methods are crucial in dynamic 2D game environments, optimizing game world’s visual consistency and performance.
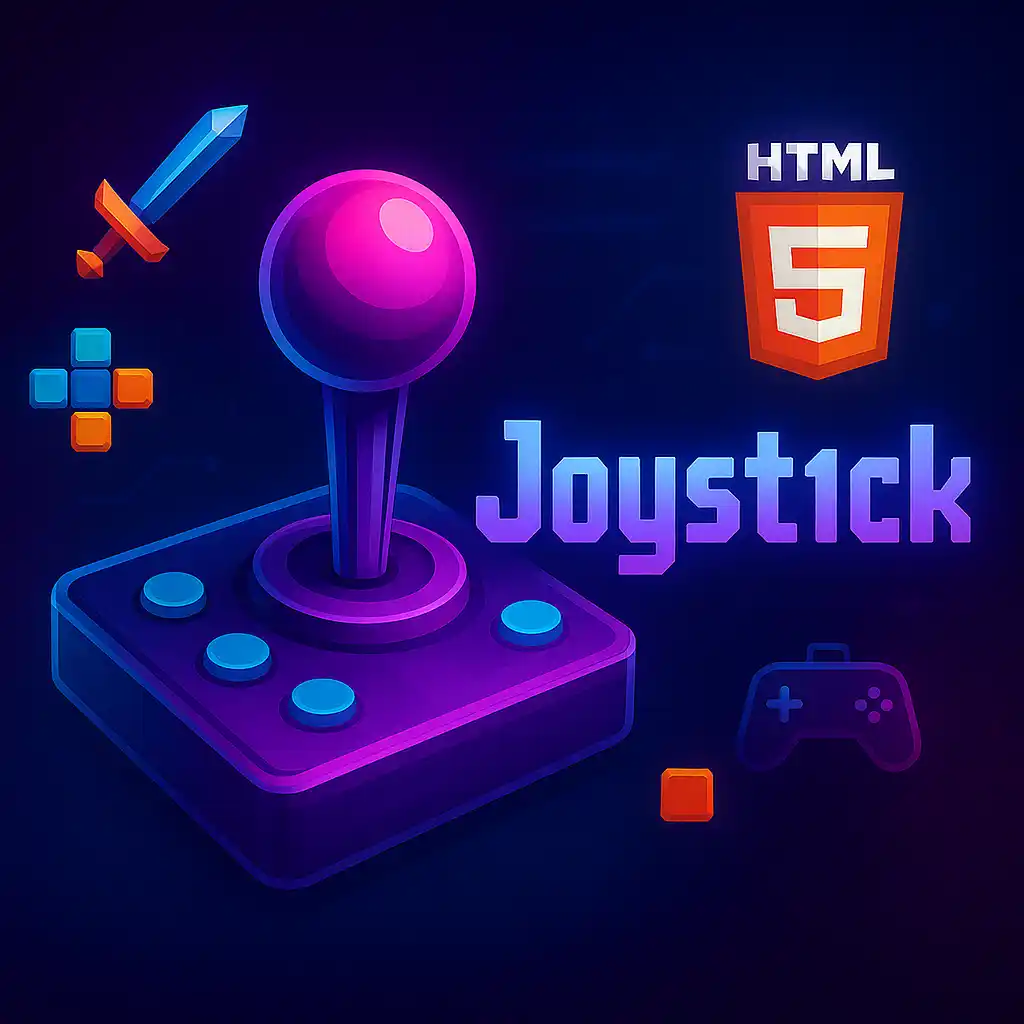