Table of Contents
Calculating Velocity for Realistic Physics in Racing Games
Calculating velocity is crucial for realistic physics in racing games, particularly when simulating forward motion, drag, and braking. To compute the velocity of a character or vehicle in a racing game, you can use the relationship between force, mass, and acceleration as defined by Newton’s Second Law.
Basic Formula
The fundamental formula to calculate velocity (v
) from force (F
), mass (m
), and initial velocity (v0
) is:
Play free games on Playgama.com
v = v0 + (F / m) * t
Where t
represents the time over which the force is applied.
Using Unity’s Physics System
- Apply Force: Use
Rigidbody.AddForce
to apply a force to the racing object. This method respects physics-based interactions and will alter velocity based on the force applied:
Rigidbody rb = GetComponent<Rigidbody>();
rb.AddForce(transform.forward * thrust);
- Retrieve Velocity: Unity’s
Rigidbody.velocity
property can then be used to obtain the current velocity vector of the body:
Vector3 currentVelocity = rb.velocity;
Ensuring Realism
- Drag and Resistance: Utilize
Rigidbody.drag
to simulate air resistance and friction that naturally slow down moving objects. - Braking: Apply a counterforce or increase drag to mimic braking effects.
Tuning and Testing
Regular tuning and testing are essential. Use Unity’s Play Mode to adjust and observe the effects of different forces and drag coefficients to achieve a realistic driving experience.
Parameter | Effect |
---|---|
mass |
Higher mass requires more force to achieve the same acceleration. |
drag |
Mimics air resistance affecting velocity over time. |
force |
Directly influences acceleration and thus velocity. |
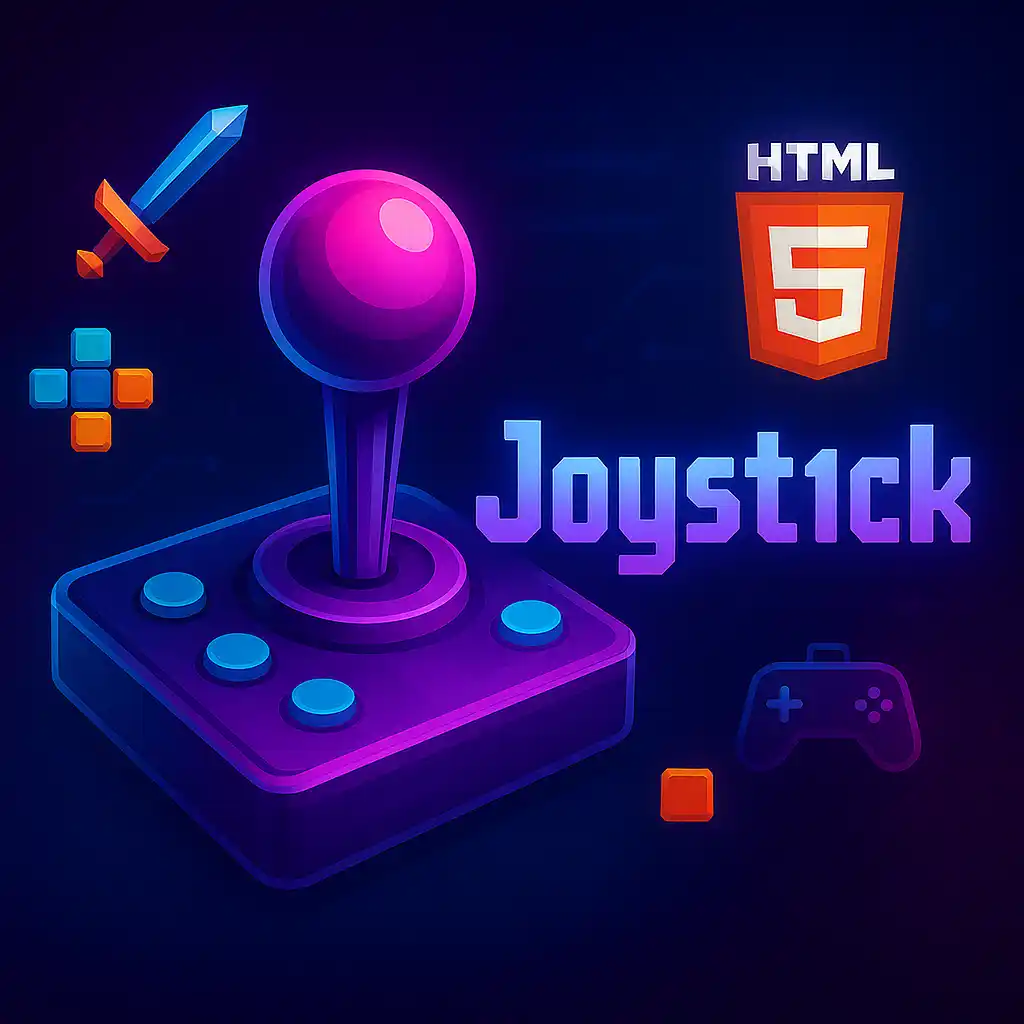