Table of Contents
Calculating Directional Vectors for Pathfinding in Unity
Understanding Directional Vectors
A directional vector describes the direction and magnitude from one point to another. In the context of a 3D space, a directional vector can help determine the movement required to get from a starting position to a target position.
Computing Directional Vectors
In Unity, you can compute the directional vector between two Vector3
points as follows:
Play free games on Playgama.com
Vector3 startPoint = new Vector3(0, 0, 0);
Vector3 endPoint = new Vector3(5, 0, 5);
Vector3 directionalVector = endPoint - startPoint;
This operation subtracts the starting point from the endpoint. The resulting directionalVector
gives you the path from the start to the endpoint.
Normalizing Vectors
Often, you’ll want a normalized directional vector with a magnitude of 1 to represent direction without considering distance. Use the normalized
property:
Vector3 normalizedDirection = directionalVector.normalized;
A normalized vector is particularly useful in pathfinding algorithms where a unit direction is needed.
Applying Directional Vectors in Pathfinding
- Gameplay Movement: You can apply directional vectors to move characters smoothly toward a target. Use the
Translate
orMoveTowards
method to update position based on the directional vector. - Dynamic Path Adjustments: For dynamic pathfinding, you may continuously recalculate the direction as targets move or as obstacles are detected.
Direction and Navigation
In addition to simple directional calculation, Unity’s navigation system can benefit from using directional vectors in more complex environments, especially when integrated with components like NavMeshAgents. By combining these vectors with pathfinding algorithms, you enhance the effectiveness and realism of navigation in your games.
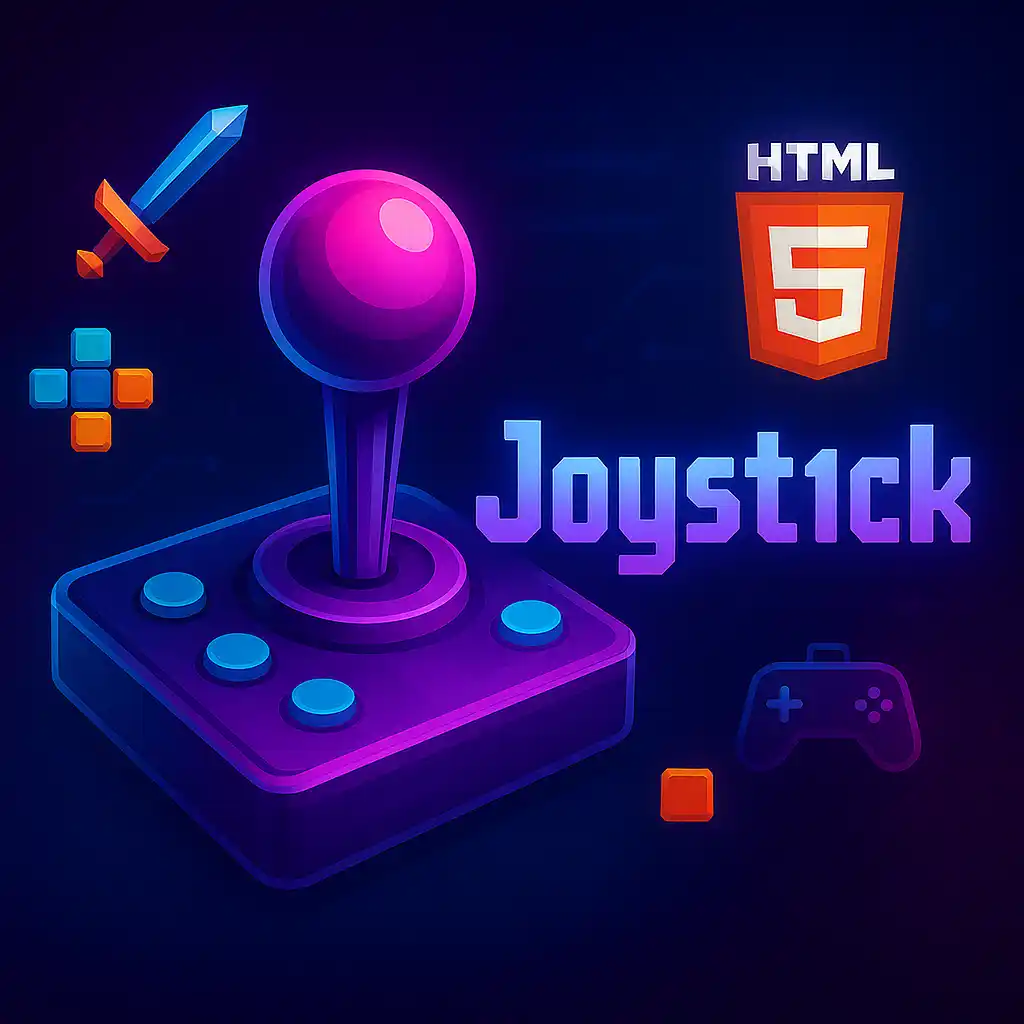