Table of Contents
Using Boolean Variables to Manage Game States in RPG Development
Introduction to Boolean Logic in Game Development
Boolean variables are fundamental in game development for tracking conditions and states due to their binary nature—true or false. In the context of an RPG, they can be employed to monitor and control various aspects like quest progression, NPC interaction states, or game phases.
Implementing Boolean Variables in Unity
using UnityEngine; public class GameManager : MonoBehaviour { private bool isQuestCompleted = false; private bool isBossDefeated = false; private bool isNightTime = false; void Update() { HandleGameStates(); } void HandleGameStates() { if (isQuestCompleted) { OpenQuestReward(); } if (isBossDefeated) { StartEndGameSequence(); } if (isNightTime) { ActivateNightMode(); } } void OpenQuestReward() { Debug.Log("Quest Reward Granted"); } void StartEndGameSequence() { Debug.Log("End Game Cinematic Started"); } void ActivateNightMode() { Debug.Log("Nighttime effects enabled"); }}
Managing Game State Transitions
By toggling these boolean variables based on player actions or in-game events, developers can dictate the game’s flow. For instance, upon completing a mission, isQuestCompleted
can be set to true, triggering a quest reward. Similarly, game ambiance changes like transitioning between day and night can be controlled with the isNightTime
variable.
Play free games on Playgama.com
Best Practices
- Descriptive Naming: Ensure boolean variables have descriptive names that clearly define their purpose, aiding maintenance and expanding readability.
- Initialization: Initialize boolean variables to a default state to avoid unexpected behavior.
- Logical Grouping: Group related boolean checks into methods to encapsulate complex logic and keep the codebase clean and maintainable.
Conclusion
Boolean variables offer a concise and effective method for managing game states in RPGs. Their strategic use can significantly enhance gameplay experience, allowing developers to maintain robust state management systems within their games.
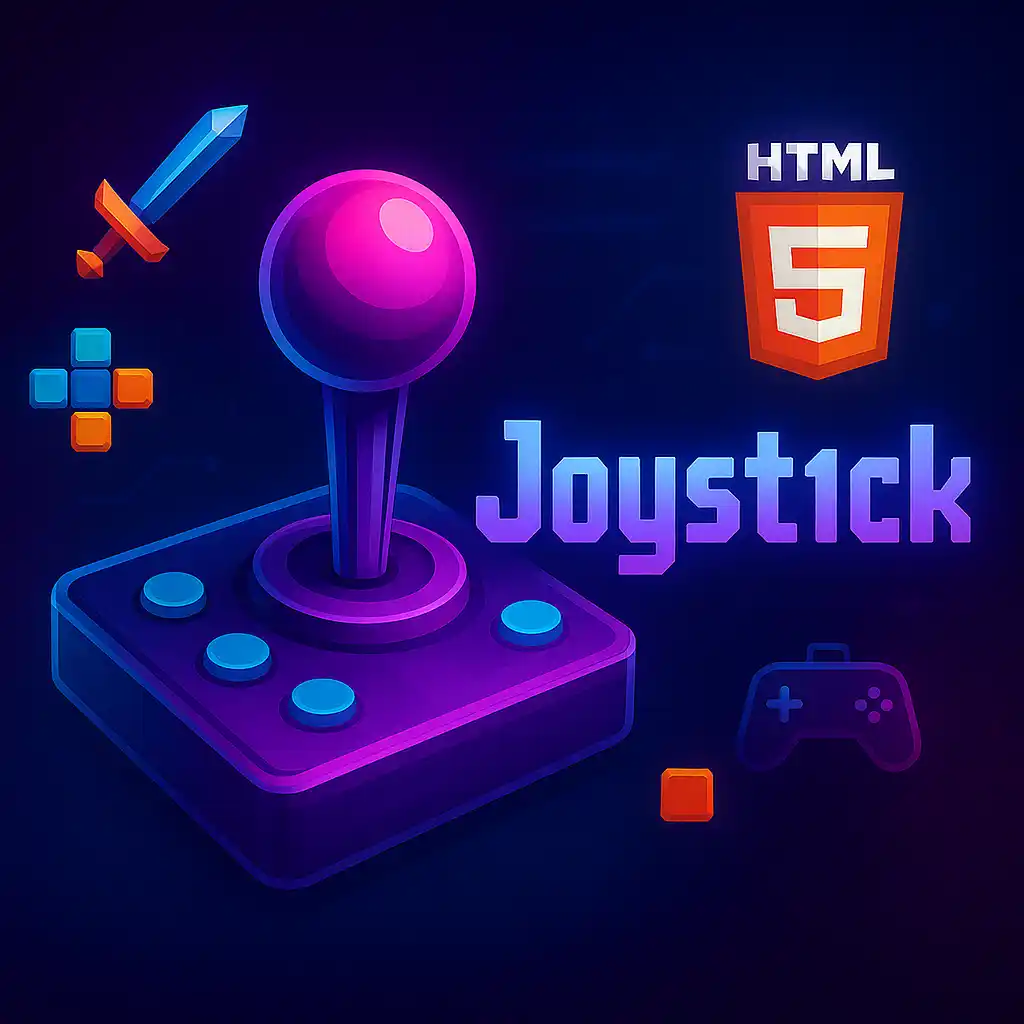