Table of Contents
Simulating Buoyancy in a Water Physics System
Understanding Buoyancy Principles
Before diving into implementation, it’s crucial to understand the principle of buoyancy, which states that an object submerged in a fluid experiences an upward force equal to the weight of the fluid displaced by the object. This principle, known as Archimedes’ principle, is the foundation for simulating buoyancy in games.
Implementing Buoyancy in a Game Engine
To simulate buoyancy, follow these steps:
Play free games on Playgama.com
- Define Object Properties: Calculate or define the volume of the object (e.g., an apple) and its mass. These properties will be crucial for calculating buoyancy forces.
- Calculate Displacement: For partially submerged objects, calculate the submerged volume. This can be complex if the object can rotate or has an irregular shape, often requiring subdividing the object into smaller manageable pieces.
- Apply Buoyancy Force: The buoyancy force is calculated as the product of the fluid density (water), gravitational acceleration, and the submerged volume of the object. In a physics engine, apply this force at the object’s center of mass to simulate upward buoyancy.
- Integrate with Physics Engine: Ensure that your implementation works seamlessly with the physics engine you’re using (e.g., Unity’s Rigidbody component). This involves continuously updating the buoyancy application each frame to account for changes in the object’s position or orientation.
Code Example in Unity
using UnityEngine; public class Buoyancy : MonoBehaviour { public float fluidDensity = 997f; // density of water in kg/m^3 public float objectVolume = 0.001f; // adjust to fit your object size void FixedUpdate() { float submergedVolume = CalculateSubmergedVolume(); Vector3 buoyancyForce = fluidDensity * Physics.gravity.magnitude * submergedVolume * Vector3.up; GetComponent().AddForce(buoyancyForce); } float CalculateSubmergedVolume() { // Here you can compute the volume based on object position relative to water surface return objectVolume; } }
Considerations for Realism
- Dynamic Adjustments: Continuously adjust the submergedVolume based on real-time interaction with the water’s surface to emulate realistic buoyancy.
- Water Resistance: Add drag to simulate water resistance that slows down the object’s motion through water, enhancing realism.
By finely tuning these parameters and ensuring accurate calculations of submerged volumes and forces, you can achieve realistic buoyancy simulations in your game.
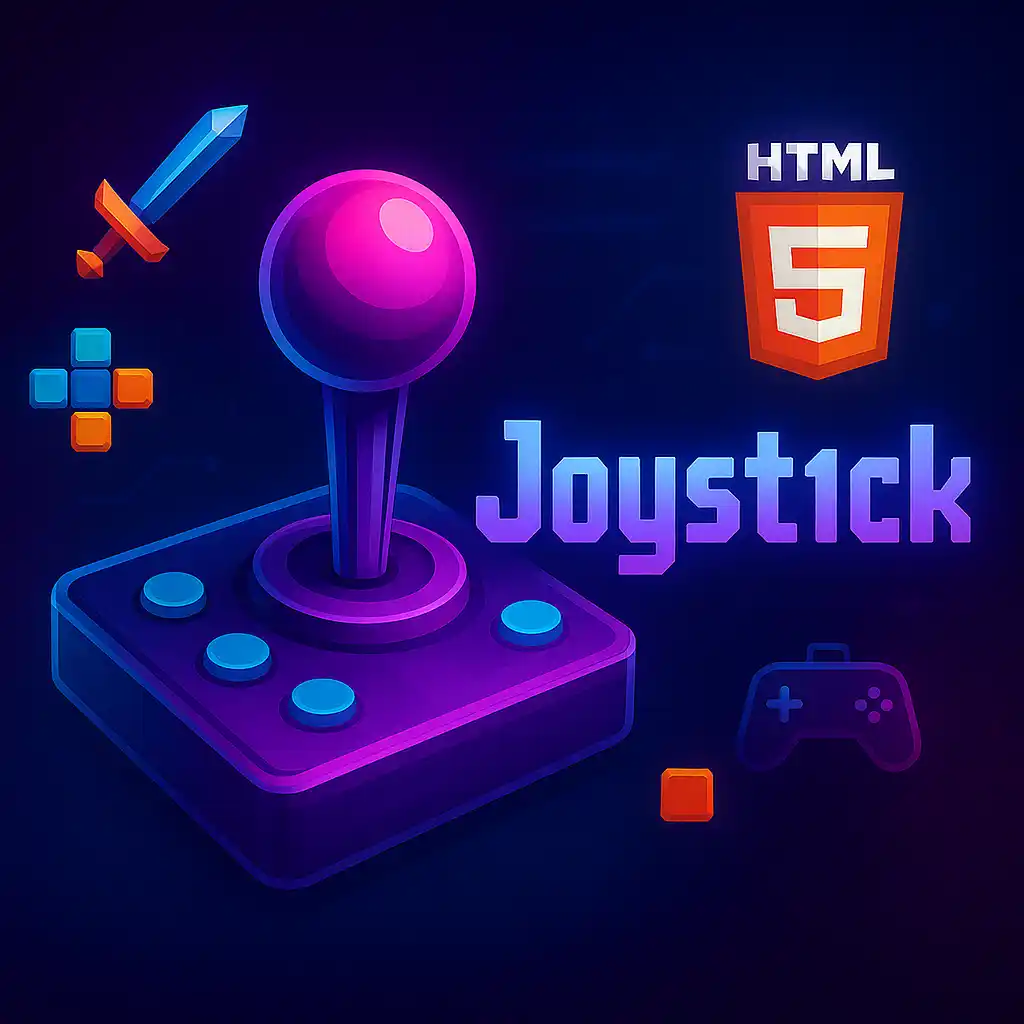