Table of Contents
Extracting and Reading ZIP Files in Unity using C#
In Unity, handling compressed ZIP files for game assets can be efficiently achieved using the System.IO.Compression namespace, which provides classes for compressing and decompressing streams.
Step-by-Step Guide
1. Importing Necessary Libraries
First, ensure you have the correct using directives at the top of your script file:
Play free games on Playgama.com
using System.IO.Compression;
2. Extracting ZIP Files
To extract a ZIP file, you can utilize the ZipFile.ExtractToDirectory method. This method extracts all files in the specified ZIP archive to a directory on the filesystem. Here’s an example:
public void ExtractZipFile(string zipPath, string extractPath) {ZipFile.ExtractToDirectory(zipPath, extractPath);}
In this function, zipPath is the path to your ZIP file, and extractPath is the directory where files will be extracted.
3. Reading Extracted Files
Once files are extracted, you can load them into your game. For instance, if you are working with textures, use Unity’s Texture2D class to load images:
byte[] fileData = File.ReadAllBytes(extractPath + "/yourImage.png");Texture2D tex = new Texture2D(2, 2);tex.LoadImage(fileData);
This method reads the image as a byte array and uses LoadImage to create a texture.
Best Practices
- Asset Management: Keep your extracted asset directory organized, separating different asset types for easier loading and management.
- Error Handling: Implement error catching around file I/O operations to manage exceptions like missing files or access violations.
Resource Management
Integrating extracted resources dynamically requires efficient memory management. Ensure that unused resources are unloaded when not needed, possibly using Resources.UnloadUnusedAssets() for memory optimization. This is crucial for maintaining performance in real-time applications.
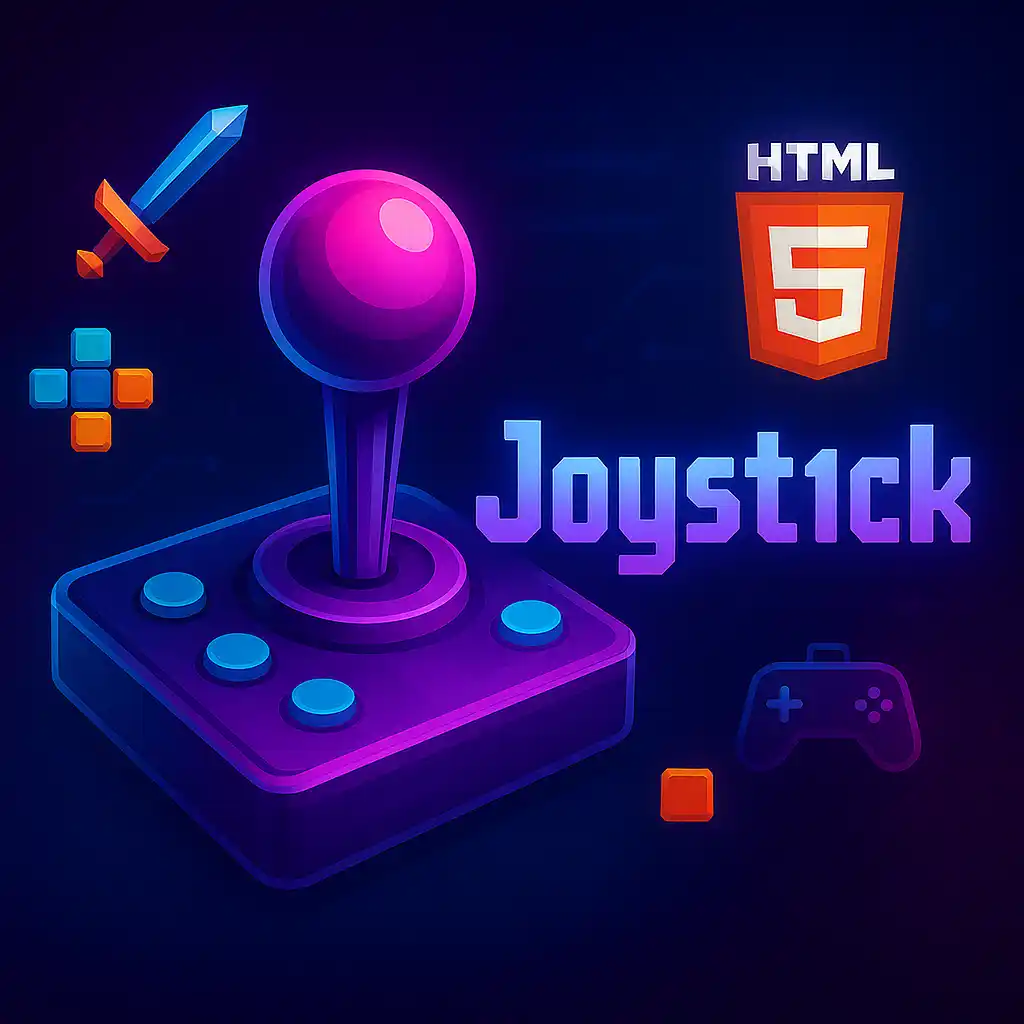