Table of Contents
Integrating Offline Mini-Game Mechanics in Unity
Introduction
Integrating an offline mini-game mechanic during loading screens can enhance player engagement and decrease perceived loading times. Using Unity, you can create engaging mini-games like the Chrome Dinosaur game by leveraging the physics and UI frameworks the engine offers.
Steps to Implement an Offline Mini-Game in Unity
1. Design the Mini-Game
- Identify the core mechanics of the mini-game (e.g., endless runner mechanics similar to the Chrome Dinosaur game).
- Create simple assets or use placeholders to design the game environment, such as the ground and obstacles.
2. Setup the Unity Project
- Start a new scene in Unity and set up a 2D project if the game is intended to mimic the Chrome Dinosaur game’s style.
- Add the necessary GameObjects such as the player character, ground, and obstacles.
3. Implement Game Logic
Player Movement:
public class PlayerController : MonoBehaviour { public float jumpForce = 10f; private Rigidbody2D rb; void Start() { rb = GetComponent<Rigidbody2D>(); } void Update() { if (Input.GetKeyDown(KeyCode.Space)) { rb.AddForce(Vector2.up * jumpForce, ForceMode2D.Impulse); } }}
Obstacle Generation:
public class ObstacleSpawner : MonoBehaviour { public GameObject obstaclePrefab; public float spawnInterval = 2f; private float timer; void Update() { timer += Time.deltaTime; if (timer > spawnInterval) { Instantiate(obstaclePrefab, transform.position, Quaternion.identity); timer = 0; } }}
4. Integrate Mini-Game with the Main Game
- Pause the main game’s loading process and display the mini-game scene.
- Monitor the loading status asynchronously and gracefully transition back to the main game once loading is complete.
5. Optimize Game Performance
- Ensure game assets are optimized to not further contribute to load times.
- Utilize sprite atlases and object pooling to maintain high performance.
Conclusion
By integrating a mini-game during loading screens, players remain engaged and entertained, dramatically improving the overall gameplay experience.
Play free games on Playgama.com
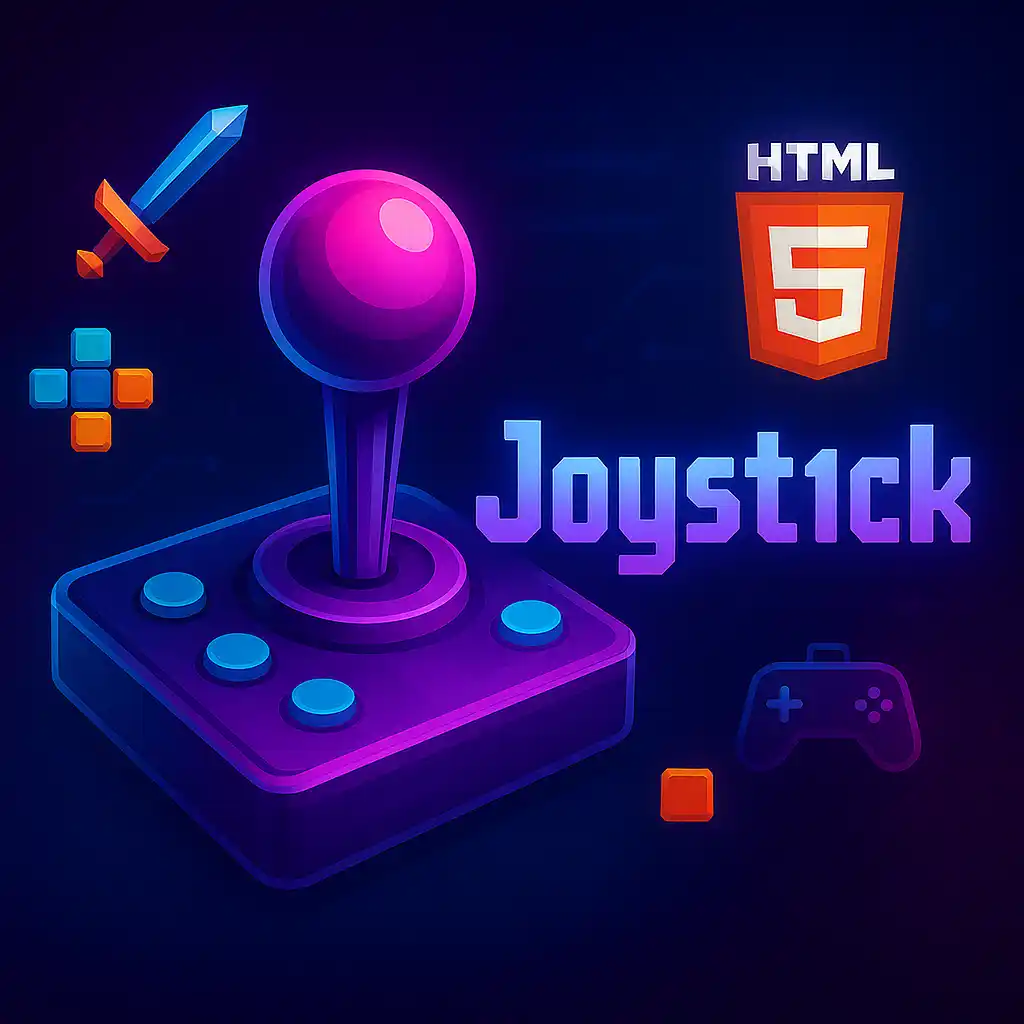