Table of Contents
Implementing Speed Units in Unity for Consistent Movement Calculations
Understanding the Need for Speed Units
In Unity, consistent movement calculations require a well-defined system for handling speed units. Speed units are critical in providing predictable and stable movement behaviors in game physics, especially when working with real-time simulations.
Defining Speed Units
- Choose a base speed unit, such as meters per second (m/s) or units per frame, which aligns with how your game handles physics calculations.
- Ensure that all components of your game physics system use this base unit to prevent discrepancies in movement calculations.
Implementing in Unity
public class MovementController : MonoBehaviour { public float speed = 5.0f; // in meters per second private Rigidbody rb; void Start() { rb = GetComponent<Rigidbody>(); } void Update() { float moveHorizontal = Input.GetAxis("Horizontal"); float moveVertical = Input.GetAxis("Vertical"); Vector3 movement = new Vector3(moveHorizontal, 0.0f, moveVertical); rb.velocity = movement * speed; }}
This code snippet demonstrates applying consistent speed units in a Unity script consistent with the physics engine’s expectations.
Play free games on Playgama.com
Ensuring Consistency Across Systems
- Verify that all forces and movements apply the same unit conventions.
- Adopt unit tests to validate physics calculations and ensure they adhere to designated units.
Handling Frame Rate Independence
To ensure consistent movement across different hardware setups, consider frame rate-independent techniques:
- Use
Time.deltaTime
for smooth frame rate-independent movement:
rb.velocity = movement * speed * Time.deltaTime;
Leveraging Physics Materials
In Unity, use physics materials to enhance consistent movement by controlling dynamic and static friction, affecting object interaction behaviors.
Optimizing Movement Algorithms
Regularly profile and optimize your movement code to ensure efficiency, especially in complex scenes.
For more insights on optimizing algorithms, consider exploring the Performance Improvements in .NET 8 which describes compiler optimizations applicable to game development contexts.
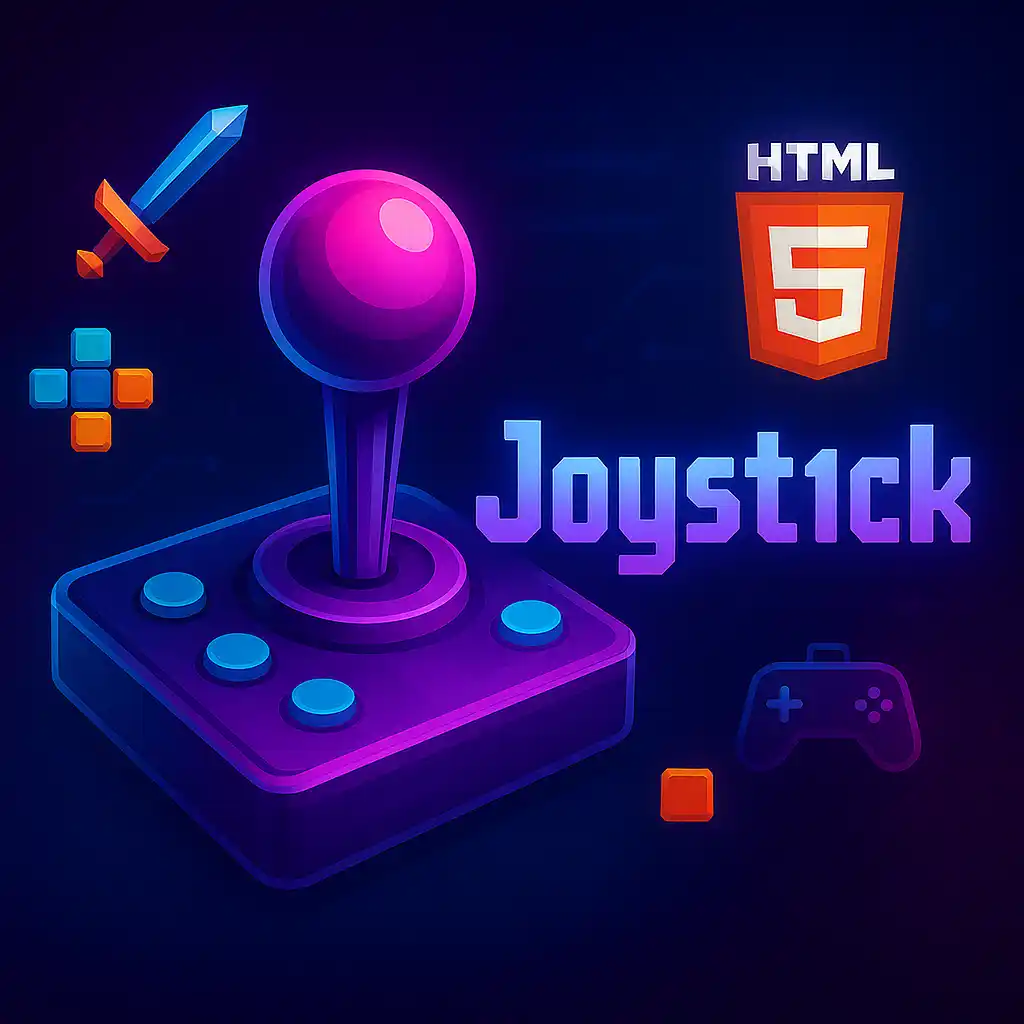