Table of Contents
Implementing Realistic Ball-Curving Physics in Unity
Creating an immersive soccer game requires realistic ball behavior, especially when simulating curved shots. Here’s how you can achieve this in Unity:
1. Understanding Ball Physics
The physics behind a curve ball involves the Magnus effect, which causes a spinning ball to curve due to differences in air pressure around it. To simulate this, you’ll need to modify the aerodynamic forces acting on the ball.
Play free games on Playgama.com
2. Calculating the Curve
void ApplyCurveForce(Rigidbody ball, Vector3 kickDirection, float spinFactor) {
Vector3 curveForce = Vector3.Cross(kickDirection, Vector3.up) * spinFactor;
ball.AddForce(curveForce, ForceMode.Force);
}
Here, kickDirection
represents the direction in which the ball is kicked, and spinFactor
represents how much spin is applied. The cross product calculates the curve force perpendicular to the kick.
3. Enhancing Realism with Drag
To further enhance realism, consider adding drag to your ball. This can be achieved by implementing:
void ApplyDrag(Rigidbody ball, float dragCoefficient) {
Vector3 dragForce = -ball.velocity.normalized * dragCoefficient * ball.velocity.sqrMagnitude;
ball.AddForce(dragForce, ForceMode.Force);
}
4. Fine-Tuning and Testing
- Use LSI phrases such as realistic ball flight dynamics and improved ball swerve mechanics to reflect adjustments that make your game more lifelike.
- Incorporate player inputs through swipes or joystick to determine the direction and magnitude of spin.
5. Simulation Testing
Test different scenarios and adjust spinFactor
and dragCoefficient
to ensure curved shots are realistic in various gameplay situations, including free kicks and long-range shots.
6. Enhancing Gameplay
Adding tutorials or indicators can help players understand how to execute curved shots successfully. Implementing feedback effects, like trail renderers, can visually enhance the curve motion.
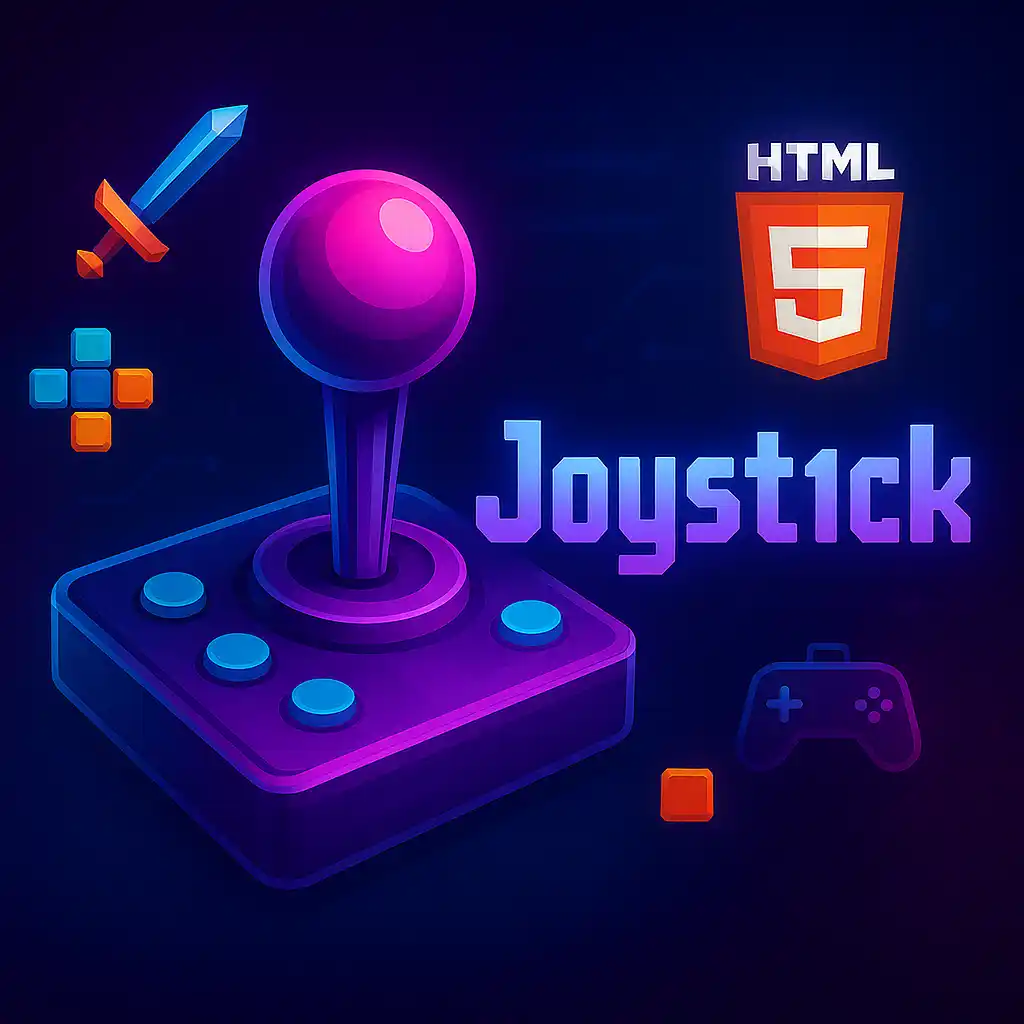