Table of Contents
Implementing Line Intersection Logic for Collision Detection in Unity
Understanding Collision Detection
Collision detection in game physics engines is crucial for ensuring accurate interactions between game objects. The line intersection method is widely used for detecting collisions in 2D and 3D environments.
Mathematical Foundations
To determine whether two line segments intersect, you can use the concept of vector algebra. The crucial part is to solve the intersection point of two lines, which can be represented parametrically.
Play free games on Playgama.com
Vector A1, A2; // Defines line 1
Vector B1, B2; // Defines line 2
Vector dA = A2 - A1;
Vector dB = B2 - B1;
float s = (-dA.y * (A1.x - B1.x) + dA.x * (A1.y - B1.y)) / (-dB.x * dA.y + dA.x * dB.y);
float t = ( dB.x * (A1.y - B1.y) - dB.y * (A1.x - B1.x)) / (-dB.x * dA.y + dA.x * dB.y);
if (s >= 0 && s <= 1 && t >= 0 && t <= 1) {
// Intersection detected
}
Implementing in Unity
- Use Unity’s
Physics2D
class for 2D games, which provides built-in methods for dealing with most physics work, but for custom logic, you might need to deal with vector calculations yourself. - For 3D environments, use
Physics.Raycast
combining it with the math logic you want to implement for specific custom intersection checks.
Optimization Techniques
To improve the efficiency of collision detection, consider Spatial Partitioning techniques such as Quad-trees or Octrees, which help reduce the number of collision checks between game objects.
Also, implement Bounding Volume Hierarchies (BVH) for more complex models to quickly reject segments that do not need precise intersection checks.
Conclusion
By embedding efficient line intersection logic directly into your physics engine, you can ensure precise and optimized collision detection that scales with your game’s complexity.
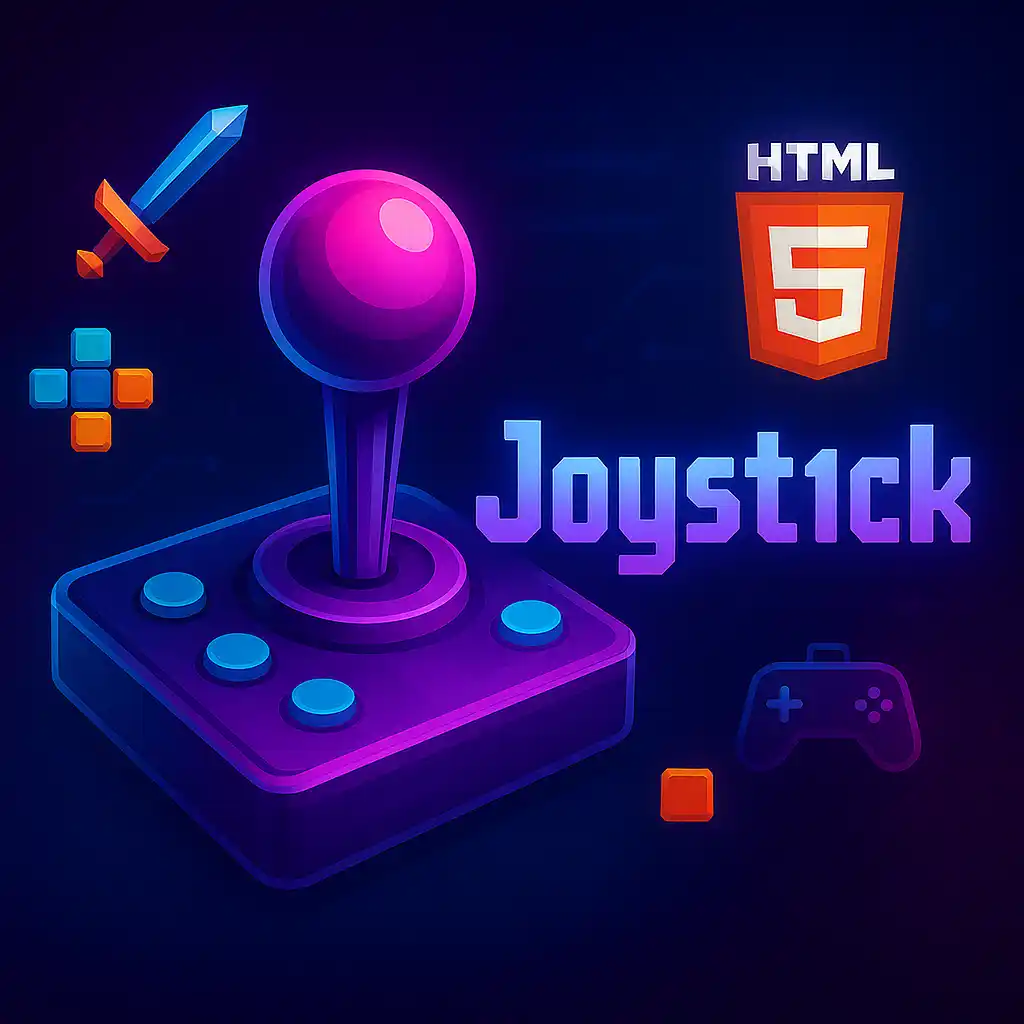