Table of Contents
Implementing Player Facing Direction in Unity
Understanding Directional Vectors
In Unity, character facing direction is often represented by a forward vector. Each object has a transform component, and transform.forward
gives the forward-facing direction in world space. You can use this to determine where the character is oriented.
Character Orientation Using Input
A common approach is to derive facing direction from player input. For instance, you may use the horizontal and vertical axes obtained from Unity’s Input System:
Play free games on Playgama.com
Vector3 movementInput = new Vector3(Input.GetAxis("Horizontal"), 0, Input.GetAxis("Vertical"));
if(movementInput != Vector3.zero) {
transform.forward = movementInput;
}
Here, transform.forward
is set to the normalized movement vector whenever there is movement input from the player.
Integrating Rotations with Animations
Once the player’s facing direction is determined, you may want to trigger animations based on this direction. A popular method is using Animator parameters:
- Create a float parameter, e.g.,
FacingAngle
. - Calculate the angle between the forward vector and the movement vector using
Vector3.SignedAngle
:
float angle = Vector3.SignedAngle(transform.forward, movementInput.normalized, Vector3.up);
animator.SetFloat("FacingAngle", angle);
Ensure the Animator transitions are set up to react to the FacingAngle
parameter shifts.
Effective Use of Quaternions
For smooth rotation transitions, consider using Quaternions:
Quaternion targetRotation = Quaternion.LookRotation(movementInput, Vector3.up);
transform.rotation = Quaternion.Slerp(transform.rotation, targetRotation, Time.deltaTime * rotationSpeed);
This guarantees a smooth and natural transition to the new facing direction by interpolating between rotations with Slerp (Spherical Linear Interpolation).
Conclusion
By linking user input with object rotations and animation parameters, a comprehensive and responsive character movement system is achievable in Unity.
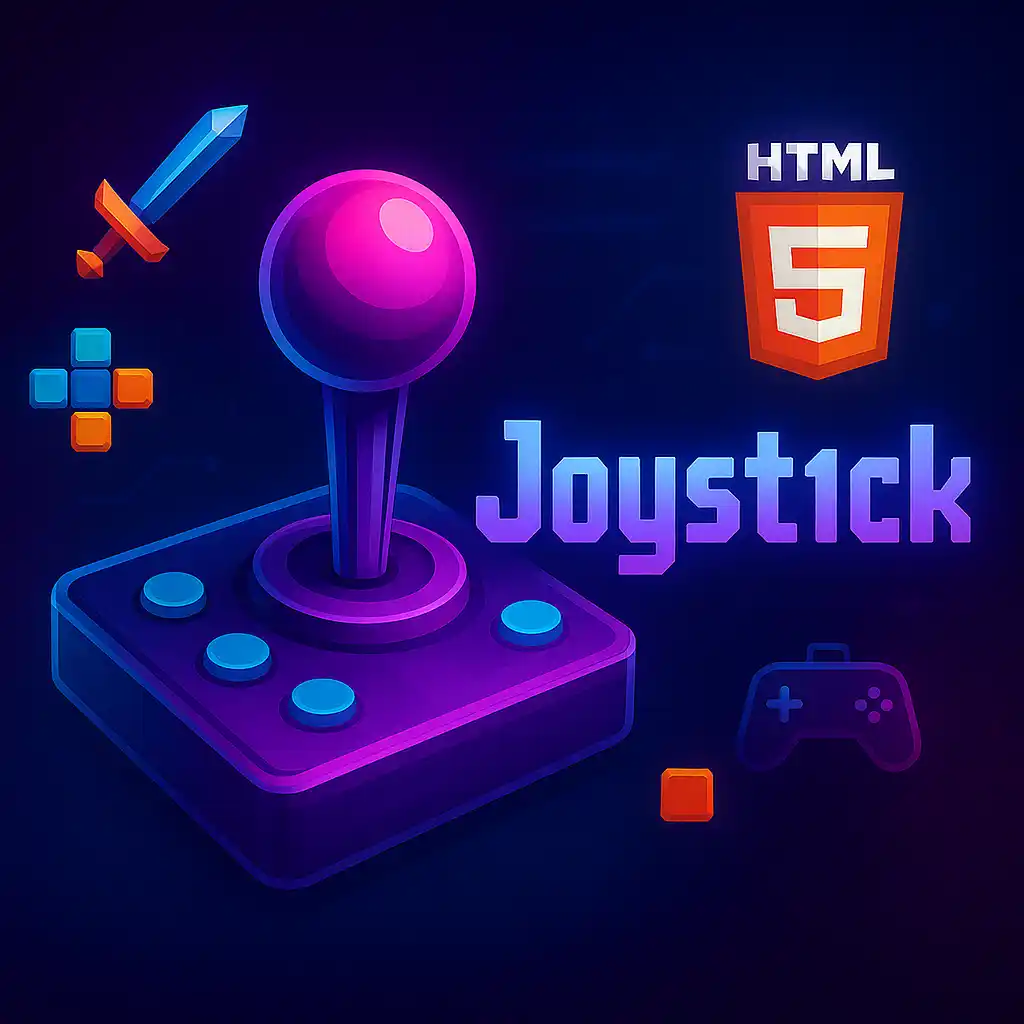