Table of Contents
Implementing a Secure User Authentication System for Mobile Games in Unity
Understanding User Authentication in Games
To implement a system that allows players to bypass restricted access, it’s essential to design a secure user authentication mechanism. Authentication ensures that only authorized users can access your game, protecting both the game’s integrity and player data.
Steps to Implement Authentication in Unity
- Select an Authentication Method: Choose between traditional username/password, social logins (Google, Facebook), or token-based authentication such as OAuth 2.0 for more secure systems.
- Integrate with Authentication Services: Use Unity’s Authentication SDK or integrate third-party solutions like Firebase Authentication, ensuring secure server communications.
- Client-side Integration: Implement authentication workflows in Unity using the UnityWebRequest or HttpClient classes for API communications.
- Backend Systems: Set up a secure server backend, possibly using AWS Cognito, Azure AD B2C, or custom built with Node.js, to handle authentication requests and validate user credentials.
Code Example
using UnityEngine;using UnityEngine.Networking;using System.Collections;public class AuthenticationManager : MonoBehaviour { IEnumerator AuthenticateUser(string username, string password) { WWWForm form = new WWWForm(); form.AddField("username", username); form.AddField("password", password); UnityWebRequest www = UnityWebRequest.Post("https://yourserver.com/auth/login", form); yield return www.SendWebRequest(); if (www.result == UnityWebRequest.Result.Success) { Debug.Log("Authentication Successful: " + www.downloadHandler.text); } else { Debug.Log("Authentication Failed: " + www.error); } }}
Security Considerations
- Use HTTPS: Ensure all data transmissions are encrypted using HTTPS.
- Secure Tokens: Utilize short-lived access tokens and refresh tokens to maintain secure sessions.
- Regular Updates: Keep authentication libraries and frameworks updated to mitigate vulnerabilities.
Play free games on Playgama.com
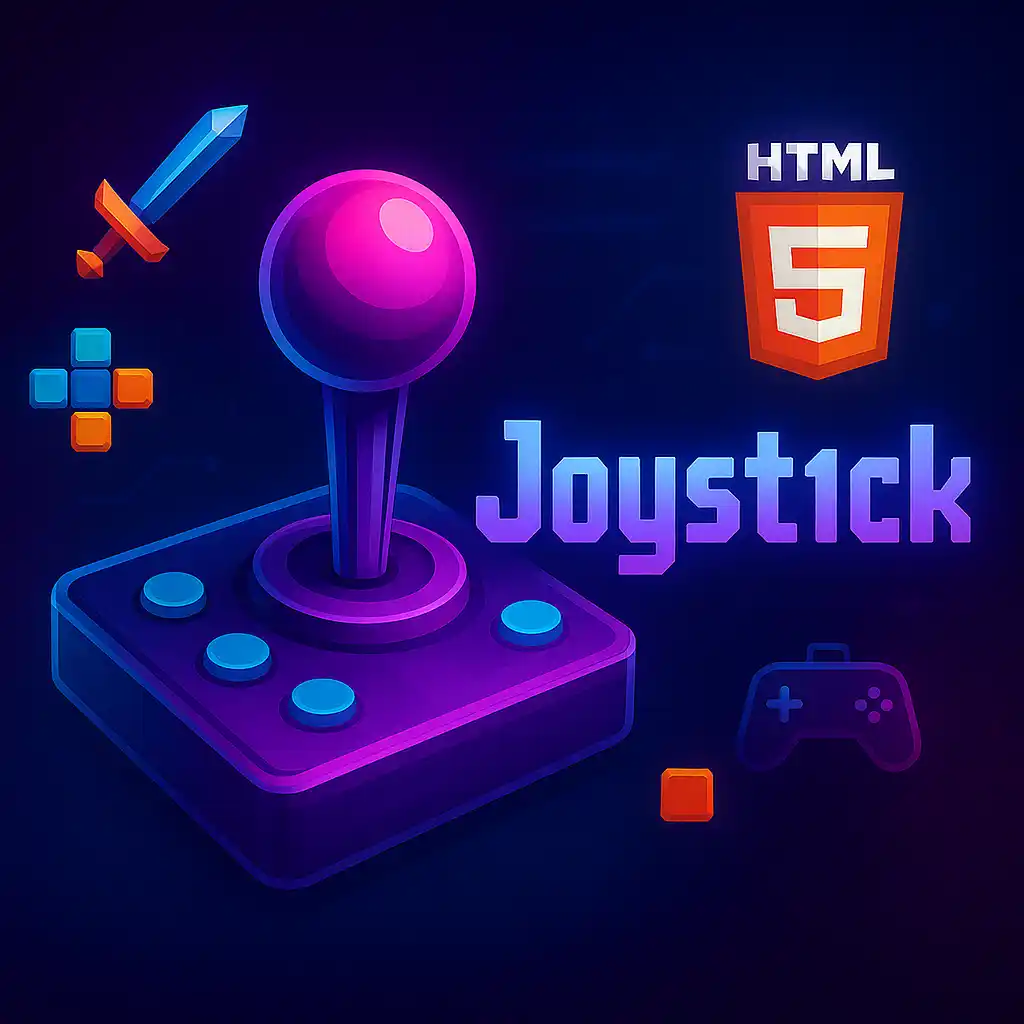