Table of Contents
Implementing a Speed Boost Mechanic in Unity
Understanding Speed Boost Mechanics
A speed boost mechanic can greatly enhance the high-speed gameplay experience in racing games like those developed in Unity. This involves temporarily increasing a vehicle’s speed and/or adjusting the physics to give players a momentary advantage.
Steps to Implement Speed Boost
- Adjust Vehicle Speed: Temporarily increase the vehicle’s speed variable. For example:
public class SpeedBoost : MonoBehaviour {
public float boostDuration = 5f;
public float boostMultiplier = 2f;
private float originalSpeed;
private void Start(){
originalSpeed = GetComponent().speed;
}
public void ActivateSpeedBoost(){
StartCoroutine(SpeedBoostCoroutine());
}
private IEnumerator SpeedBoostCoroutine(){
GetComponent().speed *= boostMultiplier;
yield return new WaitForSeconds(boostDuration);
GetComponent().speed = originalSpeed;
}
}
Optimization Tips
- Ensure the boost mechanic doesn’t decrease performance by testing different values for speed adjustments.
- Utilize Unity’s Profiler to monitor any unwanted spikes during boost activation.
- Consider using object pooling for any temporary effects or objects instantiated for boosts.
Play free games on Playgama.com
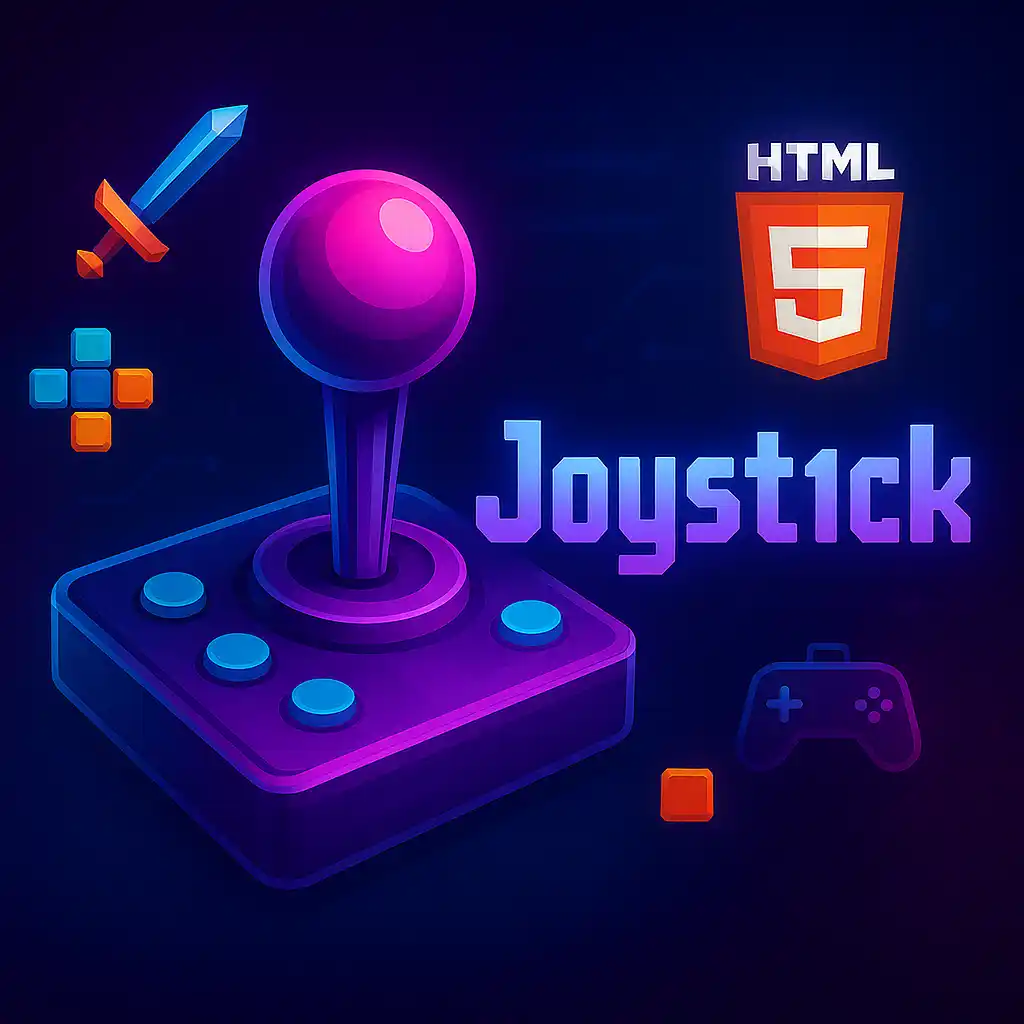