Table of Contents
Implementing Player-Facing Direction Mechanics in Unity
Understanding Character Orientation
To effectively implement a player-facing direction mechanic, it’s crucial to understand the character’s orientation data. In Unity, this typically involves manipulating the character’s transform component, particularly the transform.forward
vector, which indicates the forward direction of the character model.
Setting Up Player Input
Utilize Unity’s Input System to capture player controls for direction. Commonly, the input axes (e.g., Horizontal
and Vertical
) are used to determine intended movement direction.
Play free games on Playgama.com
float horizontalInput = Input.GetAxis("Horizontal");
float verticalInput = Input.GetAxis("Vertical");
Updating Character Direction
Once you have the input, compute the desired facing direction based on player input. Using the Quaternion.LookRotation
method can smoothly orient the character towards the input direction:
Vector3 inputDirection = new Vector3(horizontalInput, 0, verticalInput);
if (inputDirection.magnitude > 0.1f) {
Quaternion targetRotation = Quaternion.LookRotation(inputDirection);
transform.rotation = Quaternion.Slerp(transform.rotation, targetRotation, Time.deltaTime * rotationSpeed);
}
Ensuring Consistent Orientation
Carefully manage rotation speed to ensure smooth transitions and responsiveness. The Quaternion.Slerp
method is particularly useful for interpolating between rotations, providing a smooth and natural feel.
Debugging and Optimization
During development, continuously test character responsiveness and ensure no jitter occurs when changing directions. Profiling tools within Unity can help find and fix potential performance issues caused by complex calculations or integrated assets.
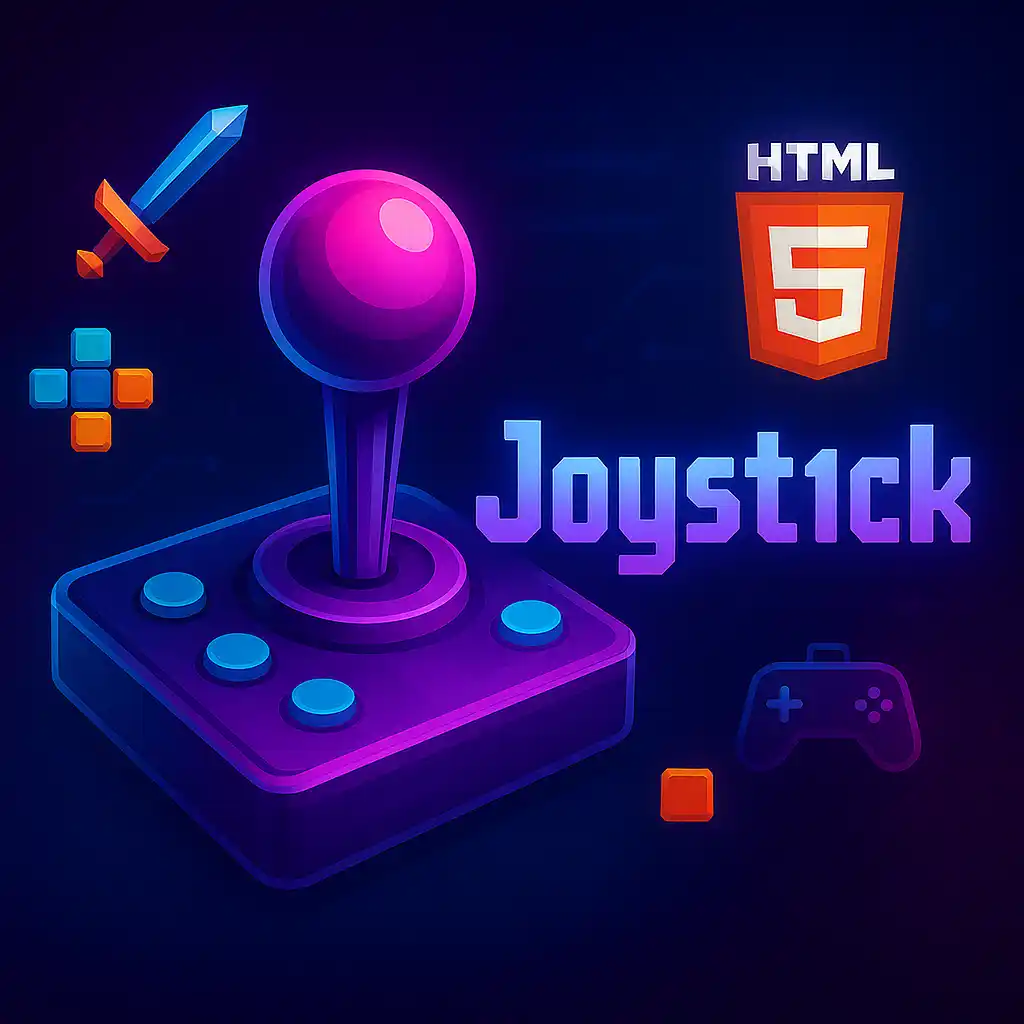