Table of Contents
Implementing Magical Circle Casting in RPG Games
Overview
Creating a magical circle casting mechanic involves multiple steps, including designing the visual elements, integrating collision detection, and triggering spell effects. Below are the recommended approaches and techniques for implementing this feature in an RPG game using Unity.
1. Designing the Magical Circle
- Visual Elements: Use Unity’s
LineRenderer
component to draw the circle dynamically based on user input or predetermined animations. Enhance the visuals using shaders from Shader Graph to give a glowing effect. - Circle Patterns: Create different patterns and styles using texture overlays to indicate different spell types or power levels.
2. Collision Detection
- Circle Collider: Use a
CircleCollider2D
orSphereCollider
in 3D space to detect objects within the circle radius. Adjust the collider scale to match the visual size of the magic circle. - Event Handling: Implement the
OnTriggerEnter
andOnTriggerExit
methods to manage interactions with other game objects when they enter or leave the circle.
3. Enhancing Spell-Casting Dynamics
- Real-time Feedback: Provide real-time feedback to players through visual cues (e.g., particle effects, sound) whenever the circle detects an object or triggers a spell.
- Spell Variations: Allow players to modify spells through circle patterns, increasing replayability and strategic depth.
- Dynamic Circles: Enable players to control the circle’s size or position in real-time using input methods like mouse drag or touch, offering more dynamic interactions.
4. Implementing Game Logic
Integrate the circle casting mechanic into your game’s spell system by associating each spell with specific circle patterns and effects. Use a script to manage spell execution once conditions within the circle are met (e.g., enemies present).
Play free games on Playgama.com
public class MagicCircle : MonoBehaviour {
public float radius = 5f;
private void OnTriggerStay(Collider other) {
if (other.CompareTag("Enemy")) {
TriggerSpellEffect(other);
}
}
private void TriggerSpellEffect(Collider target) {
// Implement spell effect
}
}
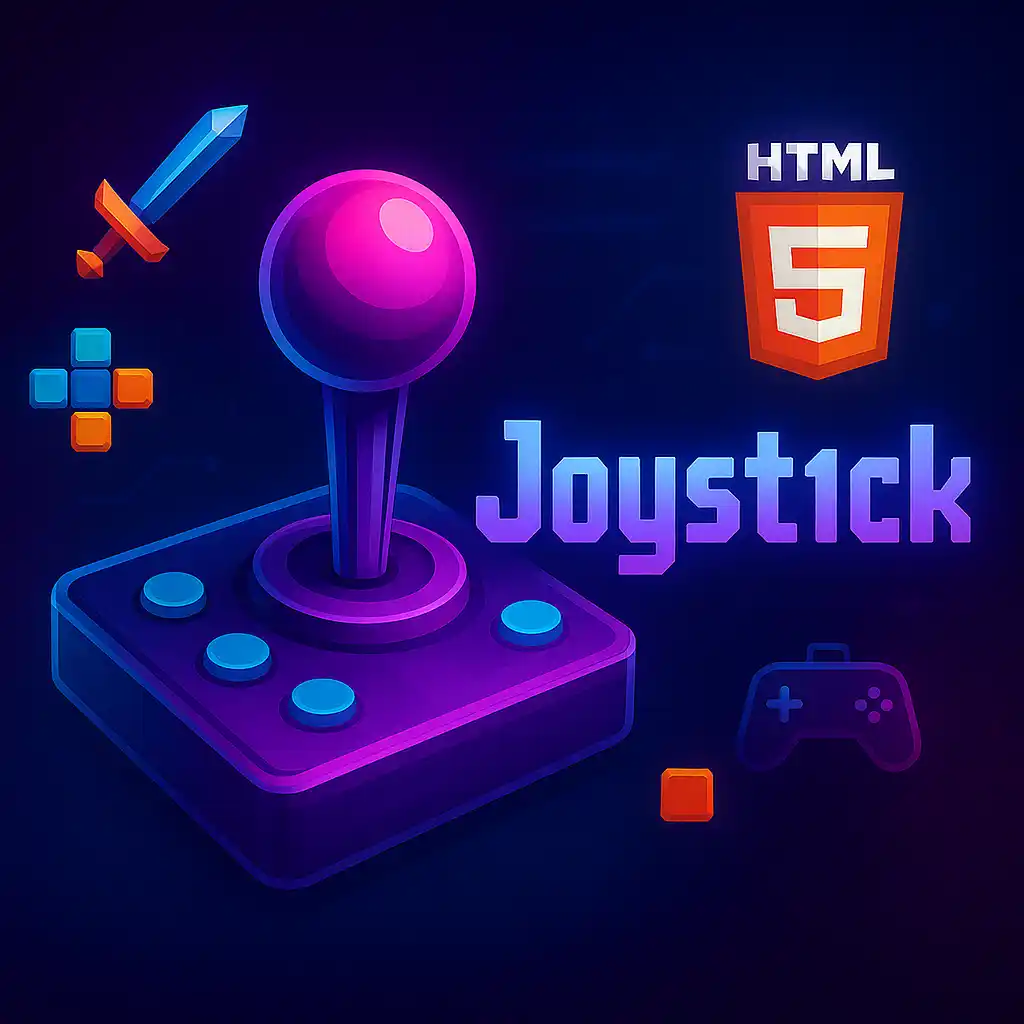