Table of Contents
Implementing a Hitbox Visibility Toggle in Voxel-Based Games
Overview
Adding a hitbox visibility toggle feature akin to Minecraft’s F3+B functionality can enhance the debugging process and improve the player’s gaming experience. Here’s a detailed breakdown of how you can implement this feature using Unity.
Steps to Implementing Hitbox Visibility
- Identify the Game Objects: Begin by identifying which objects in your game will have visible hitboxes. Common objects include NPCs, players, and interactive items.
- Create an Input Command: Use Unity’s Input System to create a new input command, such as
ToggleHitbox
. Assign this command to a suitable key (e.g., ‘H’). - Design Hitbox Visuals: Use
Gizmos
to draw the hitboxes in theOnDrawGizmos
method. This can be achieved by iterating over all relevant game objects and drawing cubes or wireframes around their collider components. - Implement the Toggle Logic: Create a boolean variable (e.g.,
isHitboxVisible
) to track the state of the hitbox visibility. The input event handler for your toggle command should flip the state of this variable. - Update the Rendering Logic: Update the
OnDrawGizmos
method to respect theisHitboxVisible
flag. Render hitboxes only when this flag istrue
.
Sample Code Snippet
void Update() {
if (Input.GetKeyDown(KeyCode.H)) {
isHitboxVisible = !isHitboxVisible;
}
}
void OnDrawGizmos() {
if (!isHitboxVisible) return;
foreach (GameObject obj in gameObjects) {
Collider collider = obj.GetComponent<Collider>();
if (collider != null) {
Gizmos.color = Color.green;
Gizmos.DrawWireCube(collider.bounds.center, collider.bounds.size);
}
}
}
Considerations
- Performance Overheads: Continuously rendering gizmos can increase load, especially with a large number of objects. Optimize by limiting visible hitboxes only to objects within the camera’s frustum.
- User Interface: Consider adding an in-game menu option to toggle the hitbox visibility, making it accessible for users not familiar with keyboard shortcuts.
Conclusion
By following these steps, you can successfully add a hitbox visibility toggle to your voxel-based game, providing both developers and players with a useful debugging tool.
Play free games on Playgama.com
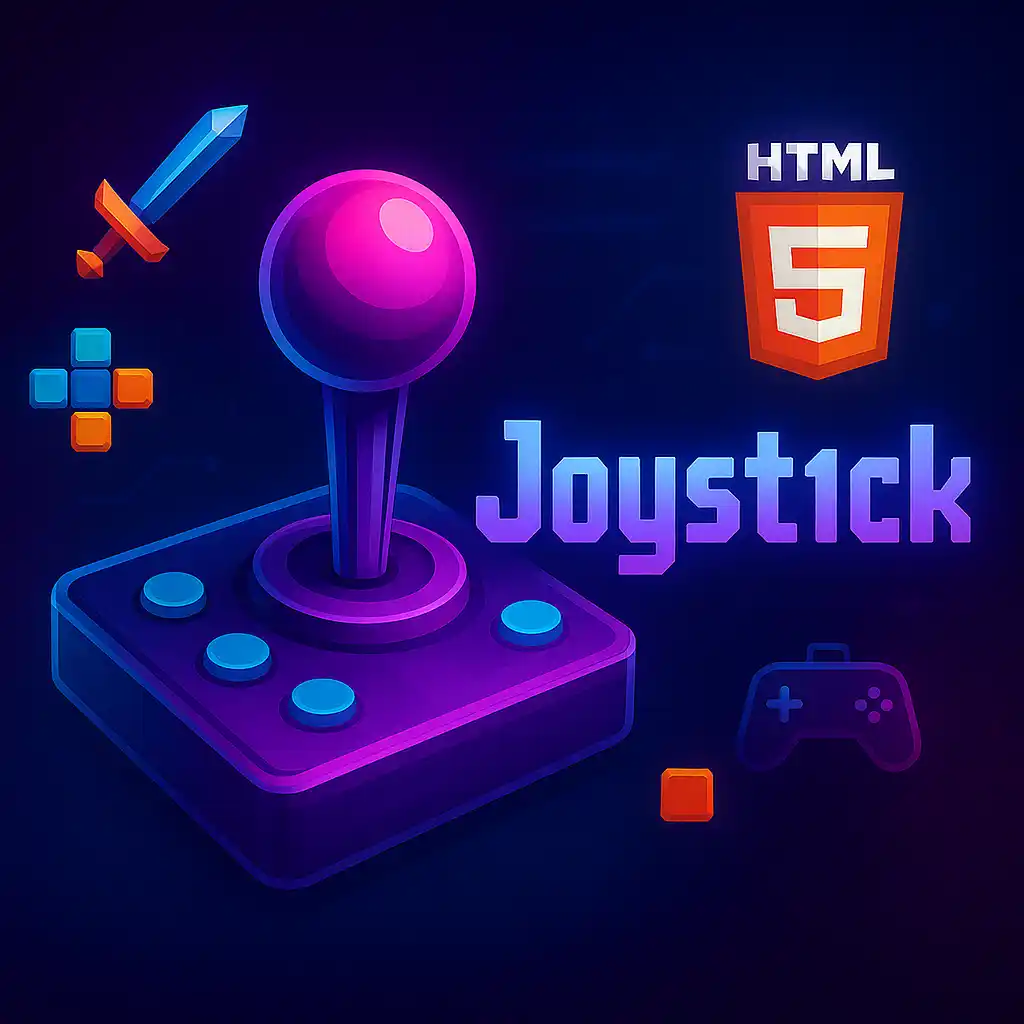