Table of Contents
Implementing a Screen Toggle Feature in Unity Using C#
To create a feature that allows toggling between different screens or menus in a Unity game, follow these steps using C#:
1. Setup Your UI Screens
- Create each screen as a separate
GameObject
within your Unity project. Make sure each screen has a different canvas or use panels that can be enabled/disabled. - Organize your hierarchy so that each screen
GameObject
can be easily identified and accessed later through C# scripts.
2. Create a Screen Manager Script
Create a ScreenManager
C# script to handle the toggling logic. This script will control the visibility of each screen based on user input.
Play free games on Playgama.com
using UnityEngine;
public class ScreenManager : MonoBehaviour {
public GameObject[] screens;
private int currentScreenIndex = 0;
void Start() {
ShowScreen(currentScreenIndex);
}
public void ToggleScreen() {
screens[currentScreenIndex].SetActive(false); // Hide current screen
currentScreenIndex = (currentScreenIndex + 1) % screens.Length;
screens[currentScreenIndex].SetActive(true); // Show next screen
}
private void ShowScreen(int index) {
for (int i = 0; i < screens.Length; i++) {
screens[i].SetActive(i == index);
}
}
}
3. Assign Screens and Trigger Events
- Attach the
ScreenManager
script to an emptyGameObject
in your scene. - Link the
screens
array in the Unity inspector to include all menu screens you want to toggle between. - Use UI buttons or key events to trigger the
ToggleScreen()
method, allowing users to switch screens.
4. Consider UI/UX Design Principles
- Smooth transition animations can enhance user experience. Consider using Unity’s animation tools or scripting for fade-in/out effects.
- Ensure buttons and toggle actions are clearly visible and intuitive to the user to improve navigation experience.
5. Debug and Test
- Test the toggle functionality extensively to ensure smooth navigation between screens with no bugs or glitches.
- Check the responsiveness of UI elements on different devices and screen sizes.
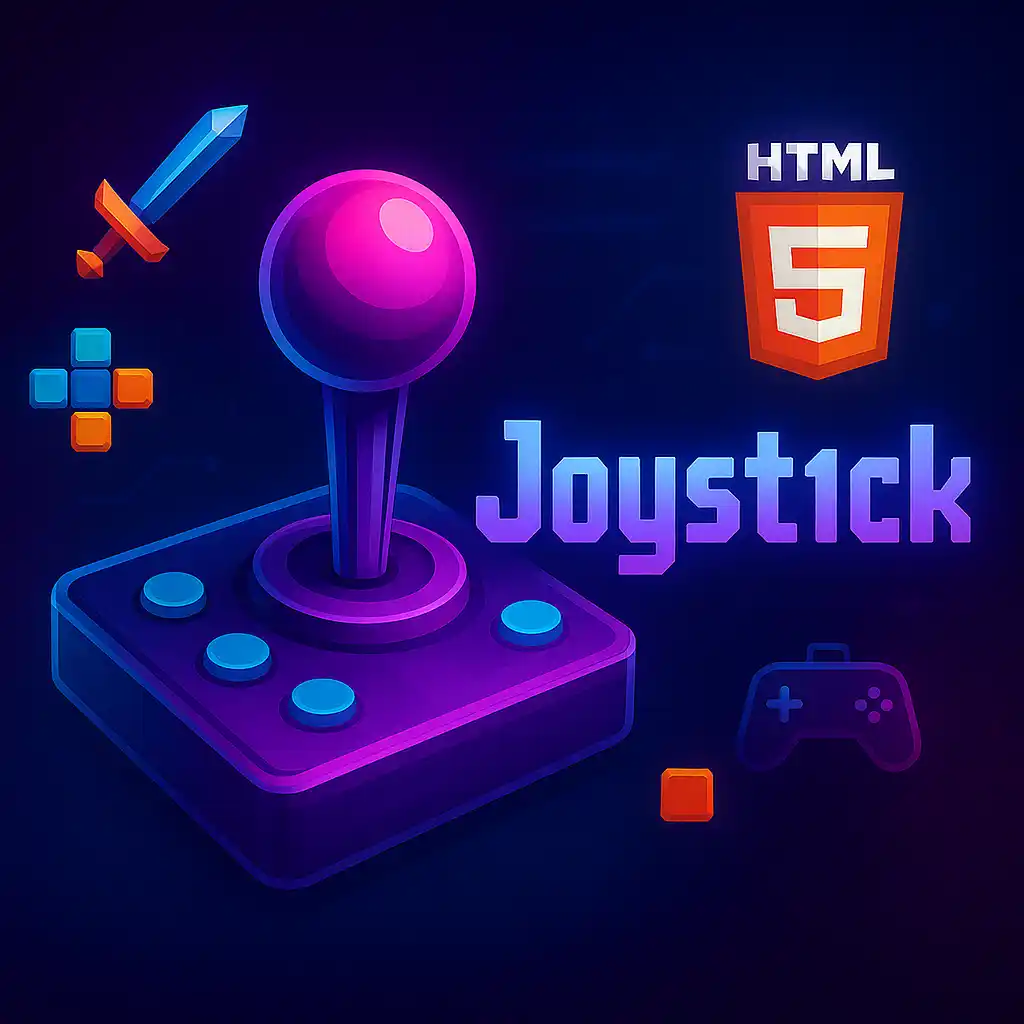