Table of Contents
Implementing a Countdown Timer in Unity
Accurate time tracking in a game’s UI can greatly enhance gameplay by providing players with the necessary pressure and pacing. Follow this guide to implement a countdown timer in Unity.
1. Set Up the Timer Text
- Create a UI Text object in your Canvas.
- Position the Text object where you want the timer to display on the screen.
2. Scripting the Countdown Logic
using UnityEngine;
using UnityEngine.UI;
public class CountdownTimer : MonoBehaviour {
public float startingTime = 60f;
private float timeRemaining;
public Text timerText;
void Start() {
timeRemaining = startingTime;
}
void Update() {
if (timeRemaining > 0) {
timeRemaining -= Time.deltaTime;
DisplayTime(timeRemaining);
} else {
// Handle end of timer (e.g., end game, alert player)
}
}
void DisplayTime(float timeToDisplay) {
timeToDisplay = Mathf.Max(timeToDisplay, 0);
float minutes = Mathf.FloorToInt(timeToDisplay / 60);
float seconds = Mathf.FloorToInt(timeToDisplay % 60);
timerText.text = string.Format("{0:00}:{1:00}", minutes, seconds);
}
}
3. Handling Timer Completion
Decide on the action when the countdown reaches zero, such as:
Play free games on Playgama.com
- Ending the game session.
- Triggering an in-game event.
- Notifying the player through UI updates.
4. Optimizing Performance
Ensure that the timer update logic is efficient by:
- Avoiding unnecessary UI updates when the time doesn’t change.
- Disabling unnecessary components when not in use.
5. Testing and Debugging
Thoroughly test the timer across various platforms to ensure accuracy. Adjust the time-based on game speed to maintain alignment with gameplay mechanics.
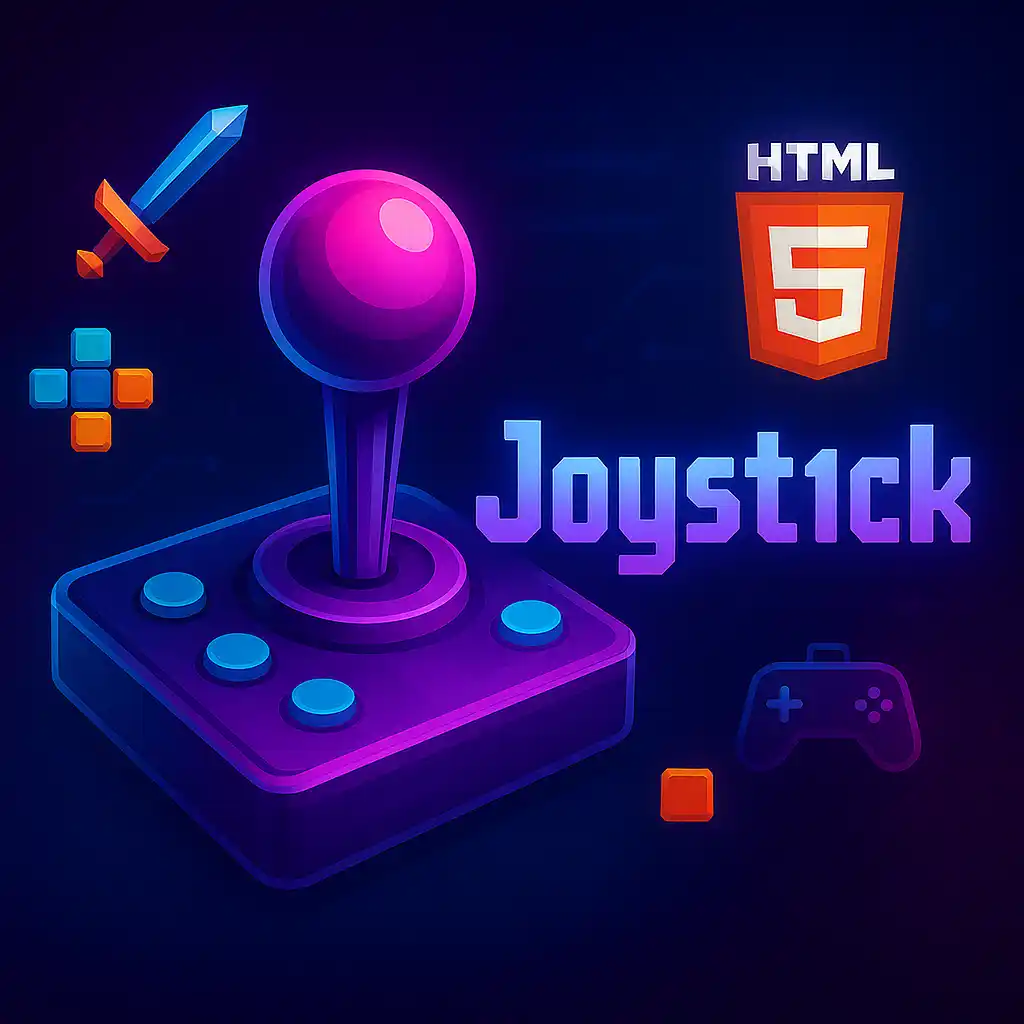