Table of Contents
Designing a Visually Appealing Character Halo Effect Using Shaders
Introduction to Shaders for Halo Effects
Shaders are essential tools in game development for creating visually impressive effects. A halo effect around a character can enhance the visual appeal and user experience. This effect can be achieved using fragment and vertex shaders that manipulate how light interacts with the character’s surface to create the desired glow.
Shader Basics
- Vertex Shader: Processes each vertex of the 3D model. Use this to adjust positions and calculate lighting normals.
- Fragment Shader: Deals with pixel colors. This is where the magic of glow creation usually happens, by adding a glow color to the character’s texture based on specific criteria like distance from the light source.
Steps to Implement a Character Halo Effect
- Define the Halo Parameters: Determine the intensity, color, and size of the halo. These can be controlled via shader properties.
- Create Shader Code: Implement the vertex and fragment shader code. Use the fragment shader to calculate the final color output, adding the halo color based on the angle or distance from the viewer or light source.
Shader "Custom/HaloEffect" {
SubShader {
Pass {
CGPROGRAM
#pragma vertex vert
#pragma fragment frag
#include "UnityCG.cginc"
struct appdata {
float4 vertex : POSITION;
};
struct v2f {
float4 pos : SV_POSITION;
};
float4 _GlowColor;
v2f vert (appdata v) {
v2f o;
o.pos = UnityObjectToClipPos(v.vertex);
return o;
}
half4 frag (v2f i) : SV_Target {
return _GlowColor;
}
ENDCG
}
}
}
Shader Optimization Techniques
- Minimize Calculations: Pre-calculate parts of your shader logic outside the main processing loop whenever possible.
- Profile and Test: Use Unity’s Profiler to monitor shader performance and ensure it runs efficiently across various hardware configurations.
Tuning for Visual Appeal
Once implemented, adjust the halo effect parameters by testing in different lighting conditions and perspectives. Adjust the intensity and color dynamically based on gameplay states to maintain aesthetic appeal and gameplay clarity.
Play free games on Playgama.com
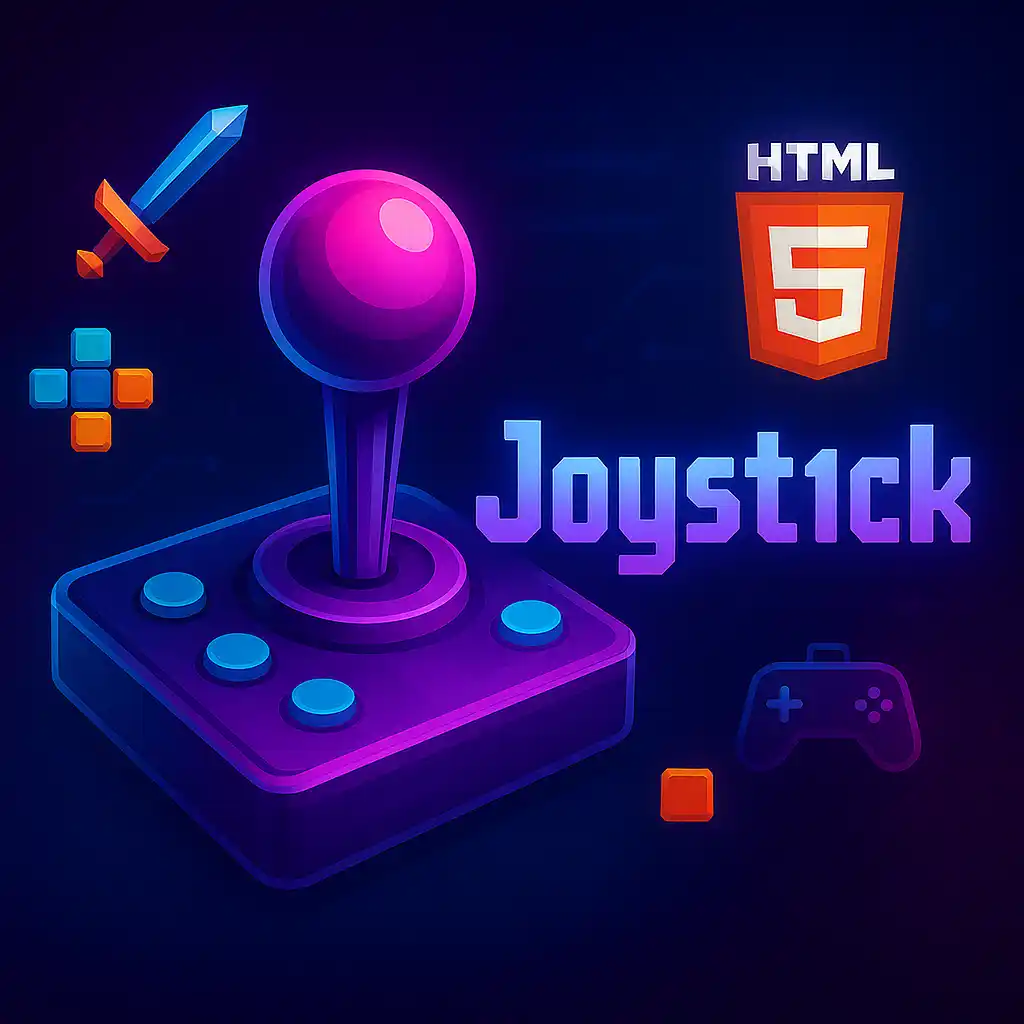