Table of Contents
Changing the Mouse Cursor in Mac-based Game Development Environment
To change the mouse cursor in a Mac-based game development environment, particularly when using Unity, follow these steps:
1. Prepare Your Custom Cursor Image
- Ensure the image is of a suitable size, typically around 32×32 pixels or 64×64 pixels, depending on your requirements.
- Use a transparent PNG format to maintain the cursor shape with the necessary transparency.
2. Import the Cursor Image into Unity
- Drag your custom cursor image into the project’s ‘Assets’ folder in Unity.
- Set its Texture Type to ‘Cursor’ via the Inspector panel. This is crucial as it optimizes the texture settings for cursor usage.
3. Implement Cursor Change in Code
Add code to change the cursor when the game starts or under specific conditions. Use the following script:
Play free games on Playgama.com
using UnityEngine;
public class CursorManager : MonoBehaviour
{
public Texture2D customCursor;
private Vector2 hotSpot = Vector2.zero;
private CursorMode cursorMode = CursorMode.Auto;
void Start()
{
Cursor.SetCursor(customCursor, hotSpot, cursorMode);
}
}
- customCursor: Reference your cursor texture here.
- hotSpot: Define the cursor’s hotspot, which determines the click point. Adjust this based on the cursor image.
- cursorMode: Typically use ‘CursorMode.Auto’ to comply with the system’s hardware cursors for better performance.
4. Consider Cross-Platform Implementation
Ensure your cursor implementation considers platform-specific differences. Unity handles cross-platform compatibility well, but always test the cursor on different platforms if targeting Windows, Linux, and Mac.
5. Testing and Debugging
Test the cursor changes thoroughly. Check for issues in cursor visibility and functionality. Debug errors by ensuring all cursor settings and paths are correctly configured.
By following these steps, you can effectively deploy custom in-game cursors in a Mac-based environment, enhancing the player’s interactive experience.
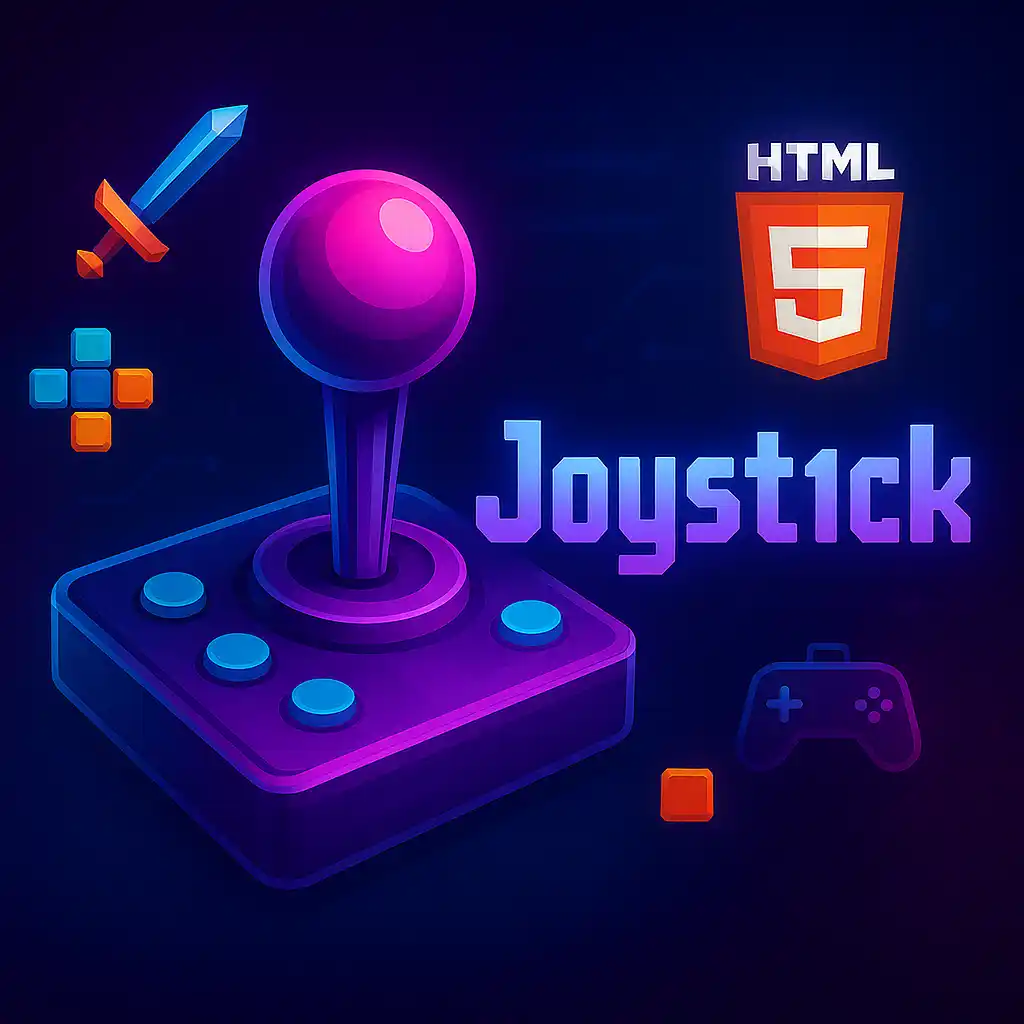