Table of Contents
Calculating Force Exerted by a Character Without Knowing Acceleration
In game development, sometimes you need to calculate the force a character exerts without directly using acceleration data. This situation often arises in physics-based games or simulations where parameters like velocity, mass, and friction are available. Here’s how you can achieve this in Unity using these parameters.
1. Understanding the Relationship Between Force, Mass, and Velocity
Physics tells us that force is the product of mass and acceleration (F = m * a). However, acceleration can be indirect in scenarios where it’s difficult to measure directly. Instead, we can use the change in velocity:
Play free games on Playgama.com
a = Δv / Δt
In this equation, ‘Δv’ represents the change in velocity over time ‘Δt’. Using this understanding, the force can be indirectly gathered through velocity changes.
2. Using Impulse
Impulse is a useful concept to apply here. It is the change in momentum, and in a discrete setting like a game frame, can be expressed as:
Impulse = Δp = m * Δv => Force * Δt = m * Δv
From this, you can isolate Force:
Force = (m * Δv) / Δt
So if you know the change in velocity and the character’s mass, you can compute the force exerted over the time period.
3. Applying in Unity
Here’s a short script snippet to calculate and display force based on velocity change:
using UnityEngine;
public class ForceCalculator : MonoBehaviour
{
public float mass;
private Vector3 lastVelocity;
void FixedUpdate()
{
Rigidbody rb = GetComponent<Rigidbody>();
Vector3 currentVelocity = rb.velocity;
Vector3 deltaVelocity = currentVelocity - lastVelocity;
float deltaTime = Time.fixedDeltaTime;
Vector3 force = (mass * deltaVelocity) / deltaTime;
Debug.Log("Force exerted: " + force);
lastVelocity = currentVelocity;
}
}
Explanation: This script assumes a Rigidbody is attached to the character. It calculates the change in velocity each frame and logs the force exerted. Note that this only considers direct forces and might not account for other factors like friction or drag.
4. Considerations
- Friction and Drag: While the above method gives a force calculated strictly from velocity changes, consider incorporating friction and drag if needed. Adjust the Rigidbody’s drag and angular drag properties to include these effects in Unity.
- Real-Time Adjustments: Game environments vary widely. Use this calculation as a base and adjust for specific environmental factors your character might interact with.
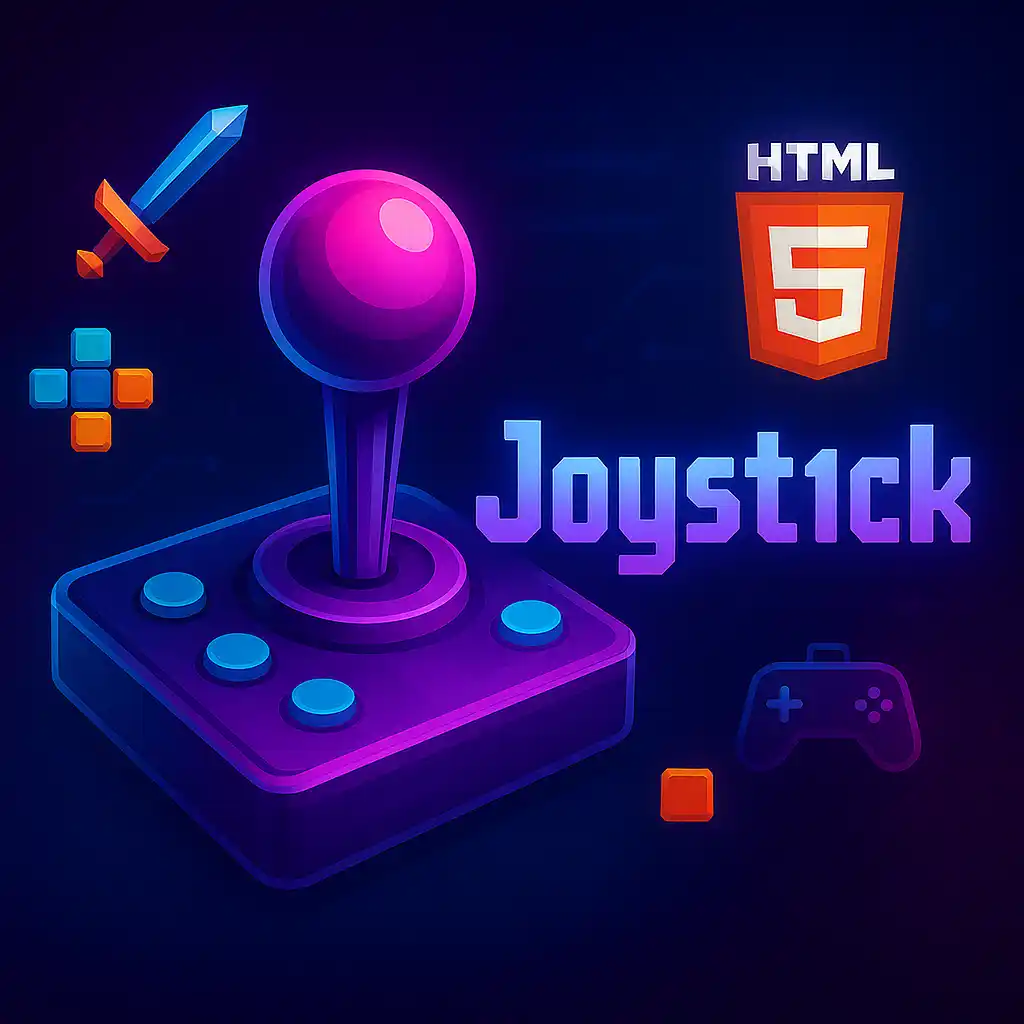