Table of Contents
Calculating the Closest Point on a Line for Collision Detection in Unity
Understanding the Problem
In collision detection, accurately determining the proximity of a game character to various geometric structures, like lines or planes, is crucial. Using mathematical algorithms to determine the closest point on a line to a character’s current position allows for precise collision responses and realistic interactions within the game world.
Mathematical Approach
- Definition of the Line: Assume your line is defined by two points in 3D space,
P1
andP2
. - Character Position: The character’s position is a point,
C
. - Vector Representation: Derive the direction vector of the line, which is
D = P2 - P1
. - Calculate Closest Point:
- Calculate
t = ((C - P1) · D) / (D · D)
. - Clamp
t
to the range [0, 1] to constrain the closest point within the segment’s endpoints if a segment is desired. - The closest point,
Q
, on the infinite line isQ = P1 + t * D
.
- Calculate
Implementing in Unity
using UnityEngine;public class LineCollision : MonoBehaviour{ public Vector3 LinePoint1; public Vector3 LinePoint2; public Transform Character; void Update() { Vector3 closestPoint = ClosestPointOnLine(LinePoint1, LinePoint2, Character.position); Debug.DrawLine(Character.position, closestPoint, Color.red); } Vector3 ClosestPointOnLine(Vector3 linePoint1, Vector3 linePoint2, Vector3 point) { Vector3 lineDir = linePoint2 - linePoint1; Vector3 lineToPoint = point - linePoint1; float t = Vector3.Dot(lineToPoint, lineDir) / Vector3.Dot(lineDir, lineDir); t = Mathf.Clamp01(t); // Ensures the point is on the segment return linePoint1 + t * lineDir; }}
Benefits of This Approach
- Precision: Offers a precise method for collision handling based on mathematical computation.
- Performance: Calculations are efficient and suitable for real-time applications in Unity.
- Flexibility: Easily adaptable to other geometric shapes and collision detection scenarios.
Further Enhancements
For complex environments, consider integrating other geometric algorithms like convex hull computations or advanced AI-driven collision strategies to handle dynamic scenarios accurately.
Play free games on Playgama.com
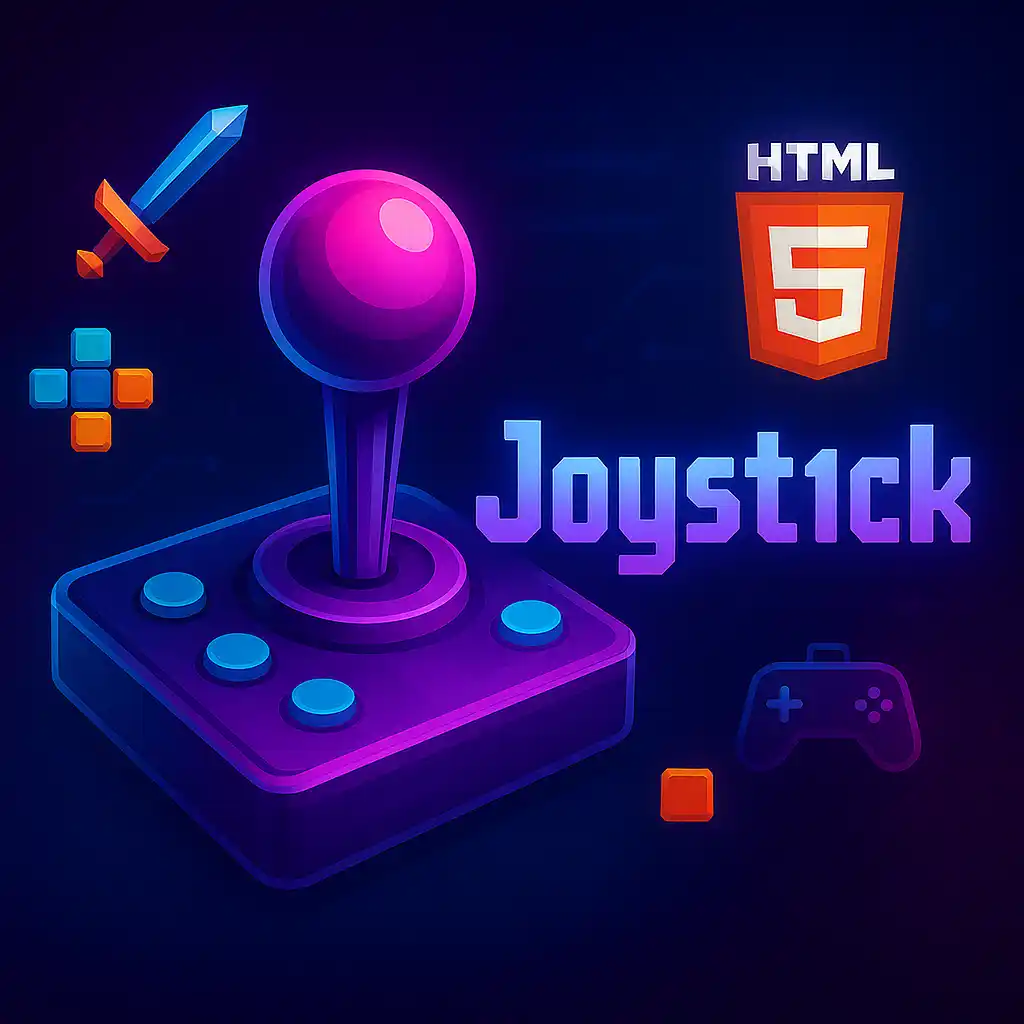