Table of Contents
Utilizing Sphere Radius for Collision Detection Optimization in 3D Game Engines
Understanding Sphere Collision Detection
In 3D game development, optimizing collision detection is crucial for improving performance, especially in physics-intensive applications. By calculating a sphere’s radius, developers can use bounding spheres to simplify complex geometry, leading to faster collision checks.
Benefits of Bounding Sphere Collision Detection
- Performance Efficiency: Bounding spheres provide a simplified representation of an object’s space, allowing the physics engine to quickly determine potential collisions without evaluating detailed mesh data.
- Simplified Calculations: Determining intersection between spheres can be reduced to a distance calculation between centers, which is computationally cheaper compared to complex polygon intersection tests.
Algorithm for Sphere Radius Calculation
To calculate the radius of a bounding sphere:
Play free games on Playgama.com
- Identify the centroid of the 3D object to serve as the sphere’s center.
- Calculate the distance from the center to the furthest vertex of the object.
- This maximum distance becomes the sphere’s radius.
Vector3 center = CalculateCentroid(objectVertices);
float maxDistance = 0f;
foreach (Vector3 vertex in objectVertices) {
float distance = Vector3.Distance(center, vertex);
if (distance > maxDistance) {
maxDistance = distance;
}
}
float sphereRadius = maxDistance;
Implementing Bounding Sphere in Unity
In Unity, developers can utilize the calculated sphere radius to integrate efficient collision detection mechanisms:
- Physics Layers: Use Unity’s layer collision matrix to limit collision checks to relevant objects only.
- Scripting: Implement OnCollisionEnter or similar methods to check against the bounding sphere first before proceeding with polygon-level collision tests.
Conclusion
By incorporating bounding sphere calculations, developers can significantly improve the efficiency of collision detection algorithms within their 3D game engines, resulting in a smoother and more performant gameplay experience.
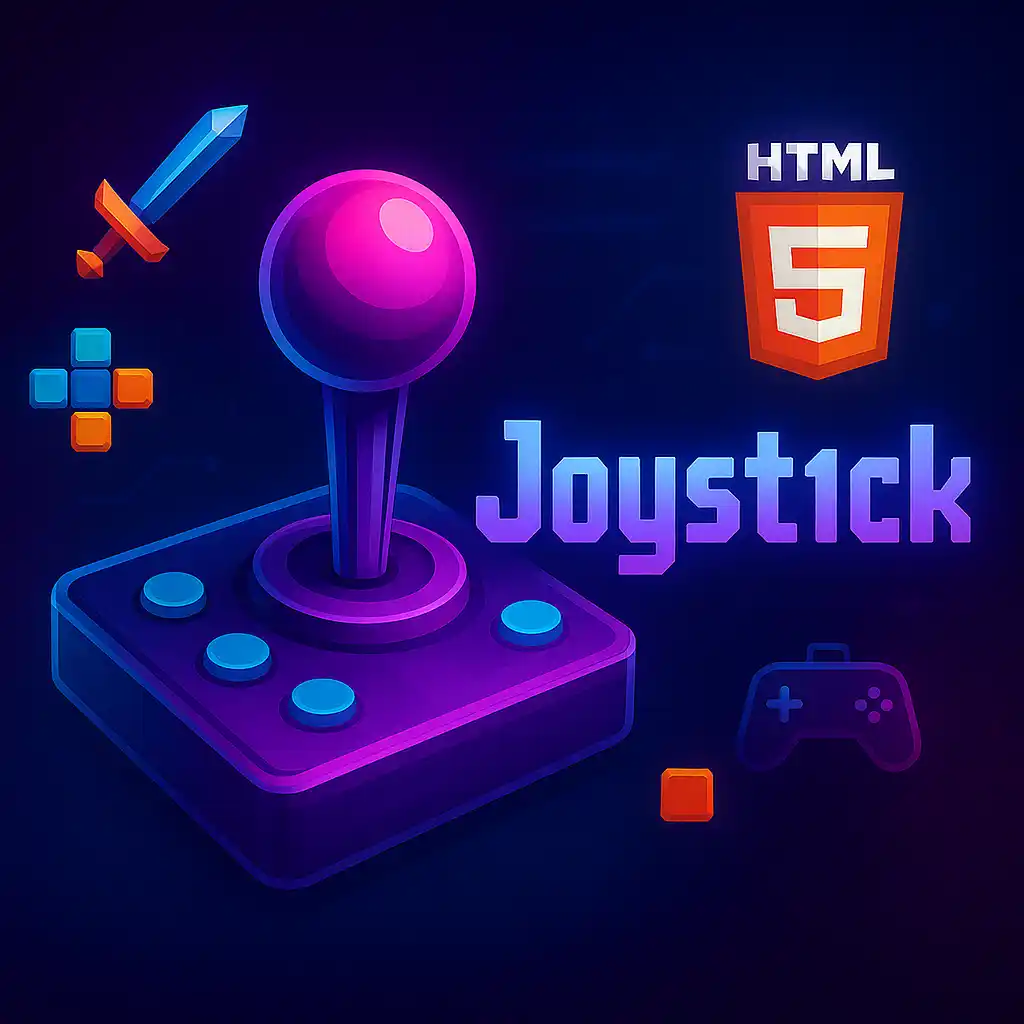