Table of Contents
Rendering a 3D Cube in OpenGL
1. Setting Up OpenGL Context
Before rendering, ensure that your OpenGL context is correctly set up. This involves initializing a window with a library like GLFW or SDL and setting up a rendering loop. Use glfwInit()
for GLFW and SDL_Init()
for SDL to start the process.
2. Defining Vertex Data
A 3D cube in OpenGL is defined by its vertices. You need to define the vertices for each corner of the cube and the indices that determine how these vertices form the cube’s faces. Here’s a sample of how you might specify this data:
Play free games on Playgama.com
float vertices[] = { // Vertex data for a cube
-0.5f, -0.5f, -0.5f,
0.5f, -0.5f, -0.5f,
0.5f, 0.5f, -0.5f,
-0.5f, 0.5f, -0.5f,
-0.5f, -0.5f, 0.5f,
0.5f, -0.5f, 0.5f,
0.5f, 0.5f, 0.5f,
-0.5f, 0.5f, 0.5f
};
unsigned int indices[] = { // Indices for drawing cube faces
0, 1, 2, 2, 3, 0,
4, 5, 6, 6, 7, 4,
0, 1, 5, 5, 4, 0,
2, 3, 7, 7, 6, 2,
0, 3, 7, 7, 4, 0,
1, 2, 6, 6, 5, 1
};
3. Setting Up Shaders
Shaders are used to control the rendering pipeline. You need to write vertex and fragment shaders to handle the transformations and coloring. Typically, GLSL is used for OpenGL shader programs.
4. Creating Buffers and Arrays
- Use Vertex Buffer Objects (VBOs) and Vertex Array Objects (VAOs) to store your vertex data and indices. Call
glGenBuffers()
to create VBOs andglGenVertexArrays()
to create VAOs. - Upload the vertex data to the GPU using functions like
glBufferData()
.
5. Rendering the Cube
Within the game loop, bind the VAO and call glDrawElements()
to render the cube using the defined vertex and index data.
glBindVertexArray(VAO);
glDrawElements(GL_TRIANGLES, 36, GL_UNSIGNED_INT, 0);
glBindVertexArray(0);
6. Adding Transformations
Apply model, view, and projection transformations to position and view the cube correctly. Use GLM or similar libraries for matrix manipulation.
- Model Matrix: Places the object in the world.
- View Matrix: Simulates a camera.
- Projection Matrix: Applies perspective.
7. Optimizing Performance
Ensure efficient rendering by minimizing state changes and batching draw calls. Implement back-face culling using glEnable(GL_CULL_FACE)
to ignore the cube’s hidden faces.
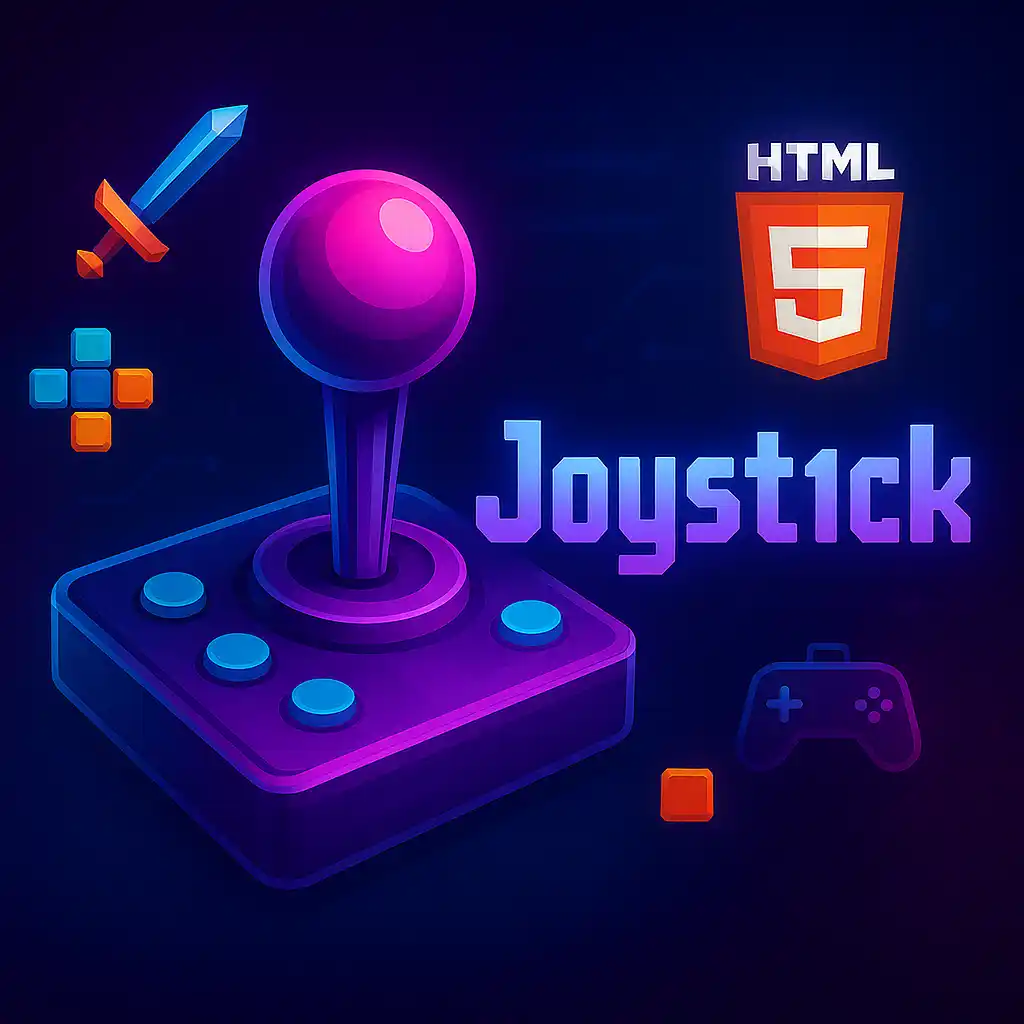