Table of Contents
Calculating and Using Normal Vectors for Light Reflection in OpenGL
Understanding Normal Vectors
Normal vectors are critical in graphics programming, especially for lighting calculations, as they define the direction perpendicular to a surface.
Calculating the Normal Vector
The normal vector for a plane can be calculated using the cross product of two edges of a triangle that forms the plane. Suppose you have three vertices of a triangle: P1, P2, P3
. The edges can be defined as:
Play free games on Playgama.com
Edge1 = P2 - P1
Edge2 = P3 - P1
The cross product of these edges will give the normal vector:
Normal = cross(Edge1, Edge2)
Ensure the vector is normalized:
Normal = normalize(Normal)
Using Normal Vectors for Light Reflection
In lighting, the reflection of light off a surface is influenced by the normal vector. The reflection vector can be determined using the incident light vector LightDir
and the normal Normal
:
Reflection = 2.0 * dot(Normal, LightDir) * Normal - LightDir
Implementing in OpenGL
- Use GLSL (OpenGL Shading Language) to calculate normals and reflections in your shaders.
- Pass vertex data, including vertices of your polygons, to the vertex shader.
- Calculate the normal in the shader using the cross product and store it in a varying variable for the fragment shader.
- In the fragment shader, use the normal and light direction to compute reflections and lighting effects.
Example Shader Snippet
void main() {
vec3 Edge1 = pos2 - pos1;
vec3 Edge2 = pos3 - pos1;
vec3 Normal = normalize(cross(Edge1, Edge2));
vec3 Reflection = reflect(-LightDir, Normal);
// Further lighting calculations
}
Debugging and Visualization
Utilize debugging tools to visualize normals and ensure accurate lighting in your 3D scenes.
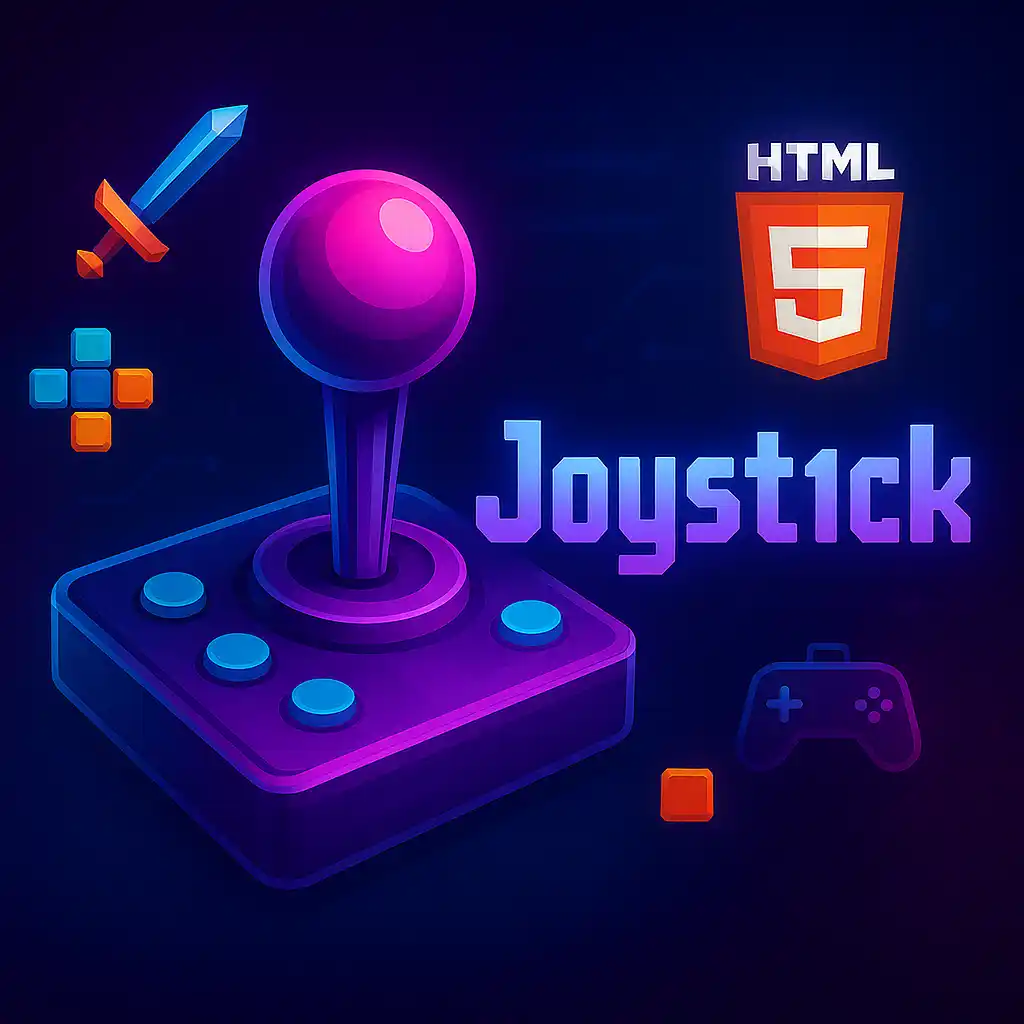