Table of Contents
Designing a Dynamic Spell-Casting System in Godot
Creating a dynamic system in Godot for enhancing player character abilities through spell-casting requires a structured approach. The following steps outline how to achieve this:
1. Define Your Spell Data Structure
Start by designing a data structure for your spells. This can be done using Godot’s built-in Dictionary
or JSON
files to manage different spell attributes such as power, type, and cooldown. For instance:
Play free games on Playgama.com
var spells = { 'fireball': {'power': 20, 'cooldown': 5}, 'ice_shield': {'power': 15, 'cooldown': 7} }
2. Create a Spell Interface
Develop a user interface that allows players to select and cast spells. Use Godot’s UI nodes such as Button
and TextureButton
to receive player inputs:
# Example code for spell button func _on_FireballButton_pressed(): cast_spell('fireball')
3. Implement Dynamic Ability Enhancements
The core of the system is an ability enhancement mechanism. Each spell should modify the player’s stats or abilities in real-time. Consider using Signals
for dynamic responses:
signal spell_cast(spell_name) func enhance_ability(spell_name): var effect = spells[spell_name]['power'] player.strength += effect # Adjust player's ability dynamically
4. Manage Cooldowns and Timers
Incorporate cooldown periods using Godot’s Timer
node to ensure balanced gameplay:
# Setup cooldown timer for a spell func _ready(): $CooldownTimer.connect('timeout', self, '_on_Cooldown_timeout') func _on_Cooldown_timeout(): can_cast_spell = true
5. Enhance Through Playtesting
Continuously test and adjust your system. Validate that the enhancements and spell effects align well with game design goals. Use feedback to fine-tune balance between various abilities.
Conclusion
By following this structured approach, you can create a rich, interactive spell-casting system in Godot that dynamically enhances player abilities and enriches the gameplay experience.
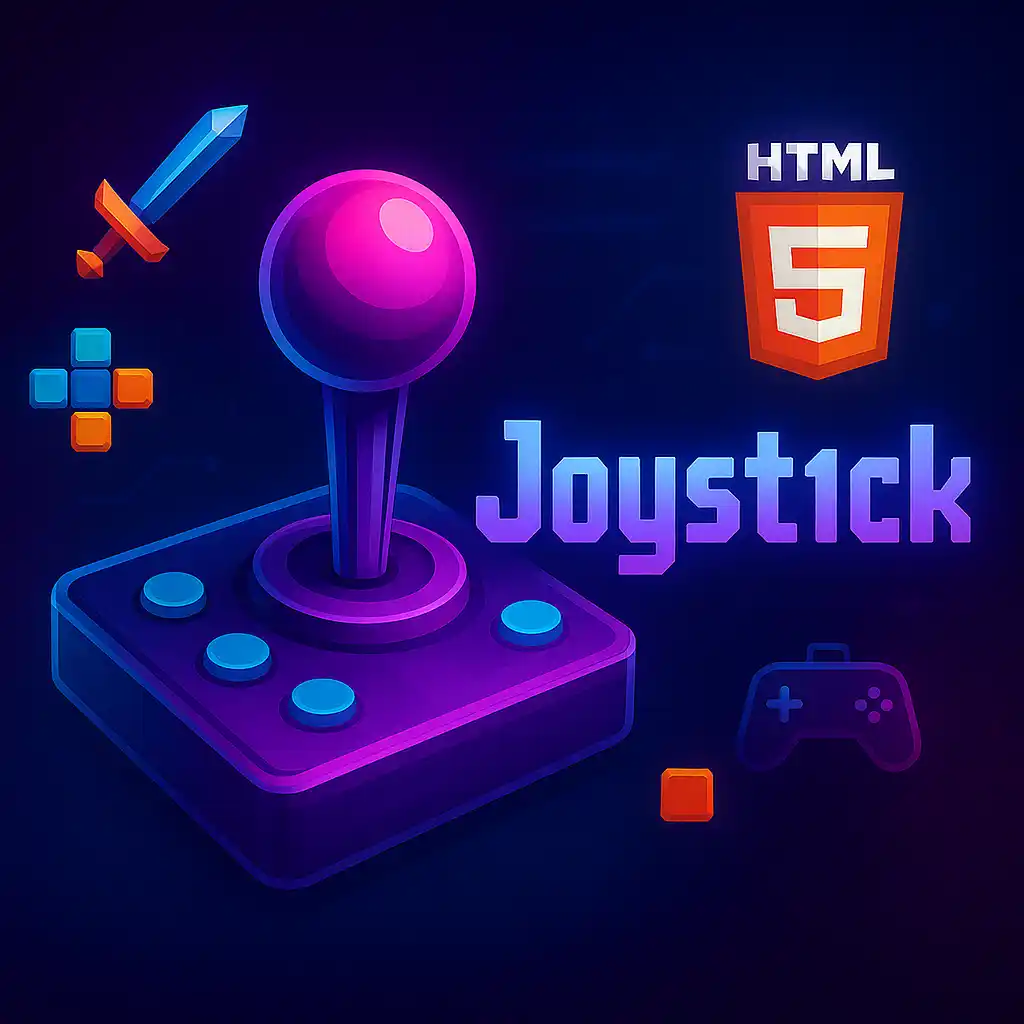