Table of Contents
Implementing an Infinite Scroller in Godot
Understanding Infinite Scrolling Mechanics
Infinite scrolling is a mechanic where the game world seamlessly loops, providing the illusion of an endless environment. It is especially popular in games like endless runners and certain puzzle genres. To achieve this in Godot, it involves setting up a repeatable segment of the game world and programmatically managing the re-positioning and recycling of these segments.
Step-by-Step Implementation
- Set Up Scene Segments: Design one or more segments of your game world to be repeated. These could be tiles, platforms, or environment sections.
- Create a Container Node: Use a Node2D to contain the segments. This node will manage the instantiation and placement of segments as they are recycled.
- Script the Scrolling Logic: Attach a script to the Container Node to handle the logic of moving the segments. This involves:
- Setting the movement speed of segments.
- Detecting when a segment exits the visible area of the screen.
- Re-positioning segments that have moved off-screen back to the starting point.
GDScript
extends Node2D
var scroll_speed = 200
var segment_width = 1024 # Example width - Recycling Segments: The core of infinite scrolling is recycling segments. When a segment moves out of the visible area, change its position to the beginning of the queue.
Optimization Considerations
Efficiently handling an infinite scroll system is crucial for performance in mobile games:
Play free games on Playgama.com
- Instance Limitation: Keep the number of active segments to a minimum necessary to fill the visible space, typically one extra to ensure seamless scrolling.
- Texture Atlases: Use atlases for your segments to minimize draw calls.
- Pooling Segments: Instead of creating and freeing segments dynamically, use an object pool to recycle existing segment instances, reducing memory allocation overhead.
With these steps, you’ll be able to implement an optimized and efficient infinite scrolling mechanic in your mobile game using Godot, enhancing the user experience and maintaining performance standards.
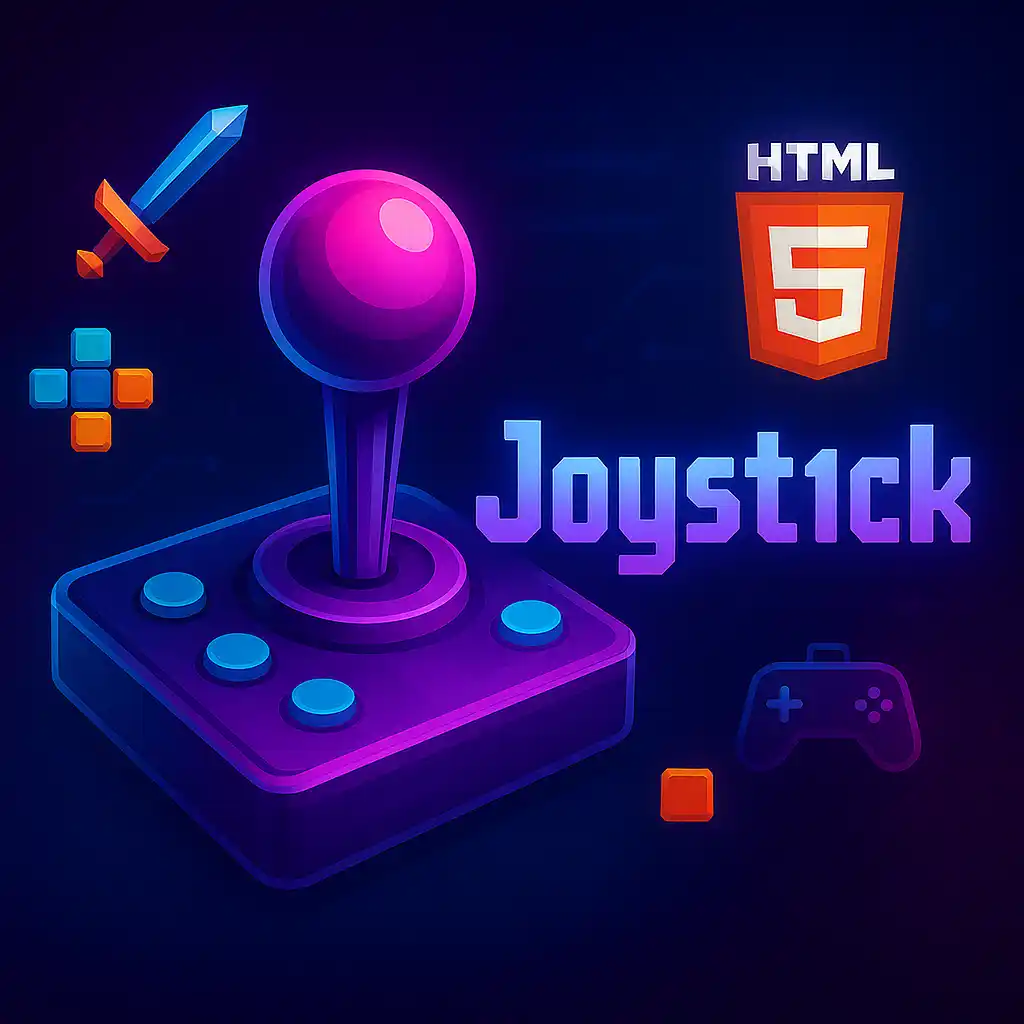