Table of Contents
Implementing a Function to Round Up Player Scores in Godot
Rounding up player scores that are stored as decimals in Godot’s game UI requires understanding the basic math functions available in the GDScript. Godot offers a straightforward way to handle mathematical operations, including ceiling functions for rounding up decimals.
Using the ceil() Function
The simplest way to round up decimal numbers in Godot is to use the built-in ceil()
function. This function rounds a floating-point number up to the nearest whole number.
Play free games on Playgama.com
var score = 10.3
var rounded_score = ceil(score)
print(rounded_score) # Outputs: 11
Implementing Rounding in the Game UI
When integrating this into your game’s UI, you can attach the function to a UI element like a score display label. First, ensure you have a Label node in your scene that will display the score.
func update_score_display(score):
var rounded_score = ceil(score)
$ScoreLabel.text = str(rounded_score)
Handling Floating-Point Precision
Be cautious of floating-point precision errors which can occur in any programming environment. Godot handles most scenarios effectively, but be aware of this when performing multiple arithmetic operations on floats.
Example Integration
An example workflow could involve updating the score display whenever the player’s score is modified:
func _on_ScoreChanged(new_score):
update_score_display(new_score)
You would connect this function to any signal that involves score changes, ensuring the UI is always updated with the rounded value.
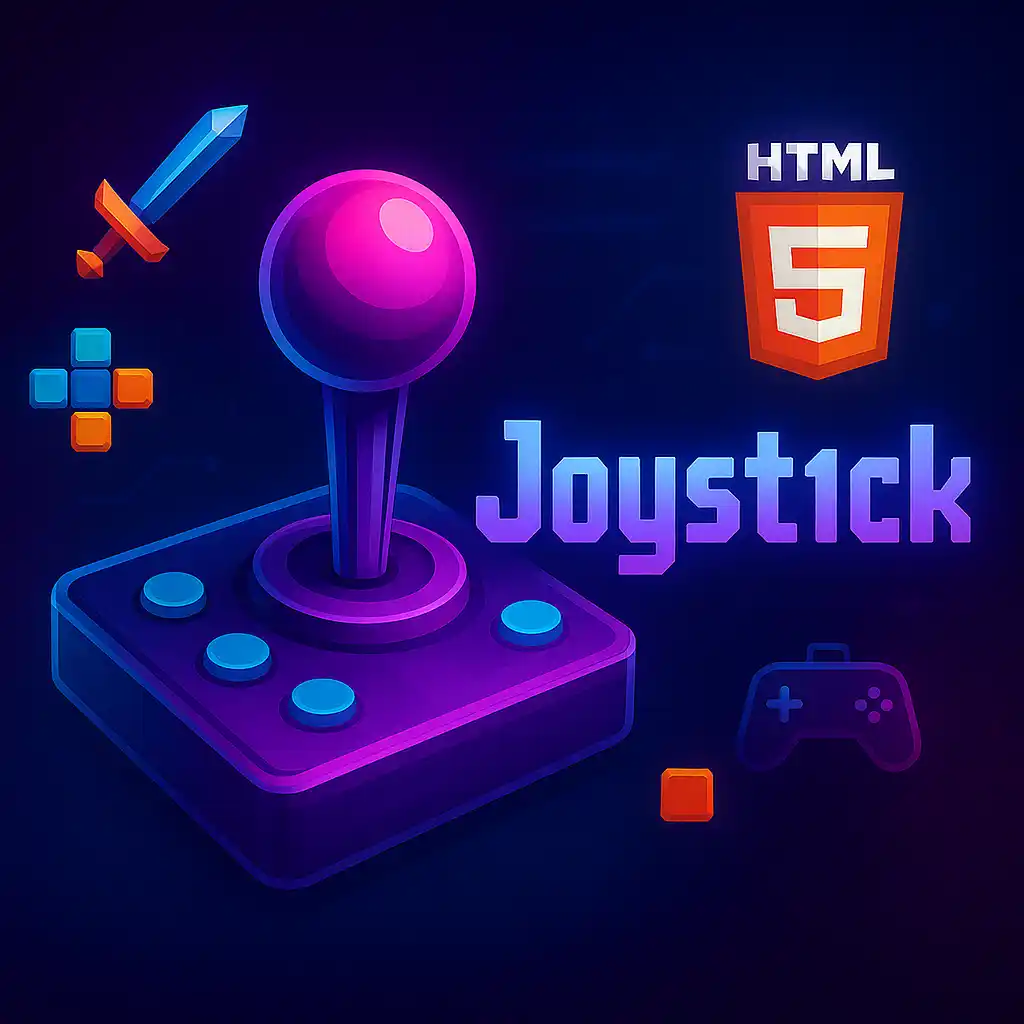