Table of Contents
Creating and Using JSON Files for Game Settings in Godot
Step 1: Define Your Settings Structure
Start by determining the settings you need to store. For example:
{
"resolution": "1920x1080",
"volume": 75,
"fullscreen": true,
"language": "en"
}
Step 2: Creating JSON in Godot
Use Godot’s File class to create and write JSON files. Here’s a simple example:
Play free games on Playgama.com
var settings = {
"resolution": "1920x1080",
"volume": 75,
"fullscreen": true,
"language": "en"
}
var file = File.new()
file.open("user://settings.json", File.WRITE)
file.store_string(to_json(settings))
file.close()
Step 3: Reading JSON at Startup
To read the settings at startup, you can do the following:
func _ready():
var file = File.new()
if file.file_exists("user://settings.json"):
file.open("user://settings.json", File.READ)
var settings = parse_json(file.get_as_text())
file.close()
apply_settings(settings)
func apply_settings(settings):
OS.set_window_fullscreen(settings["fullscreen"])
AudioServer.set_bus_volume_db(0, settings["volume"].to_db())
# Apply other settings as needed
Step 4: Managing JSON Data
Utilize JSON schemas or other validation methods to ensure data integrity and avoid common pitfalls in JSON handling.
Best Practices
- Always validate JSON data before using it in the game to prevent errors.
- Consider providing a default settings file in case the user file is corrupted or missing.
- Regularly back up settings files to avoid loss of user preferences.
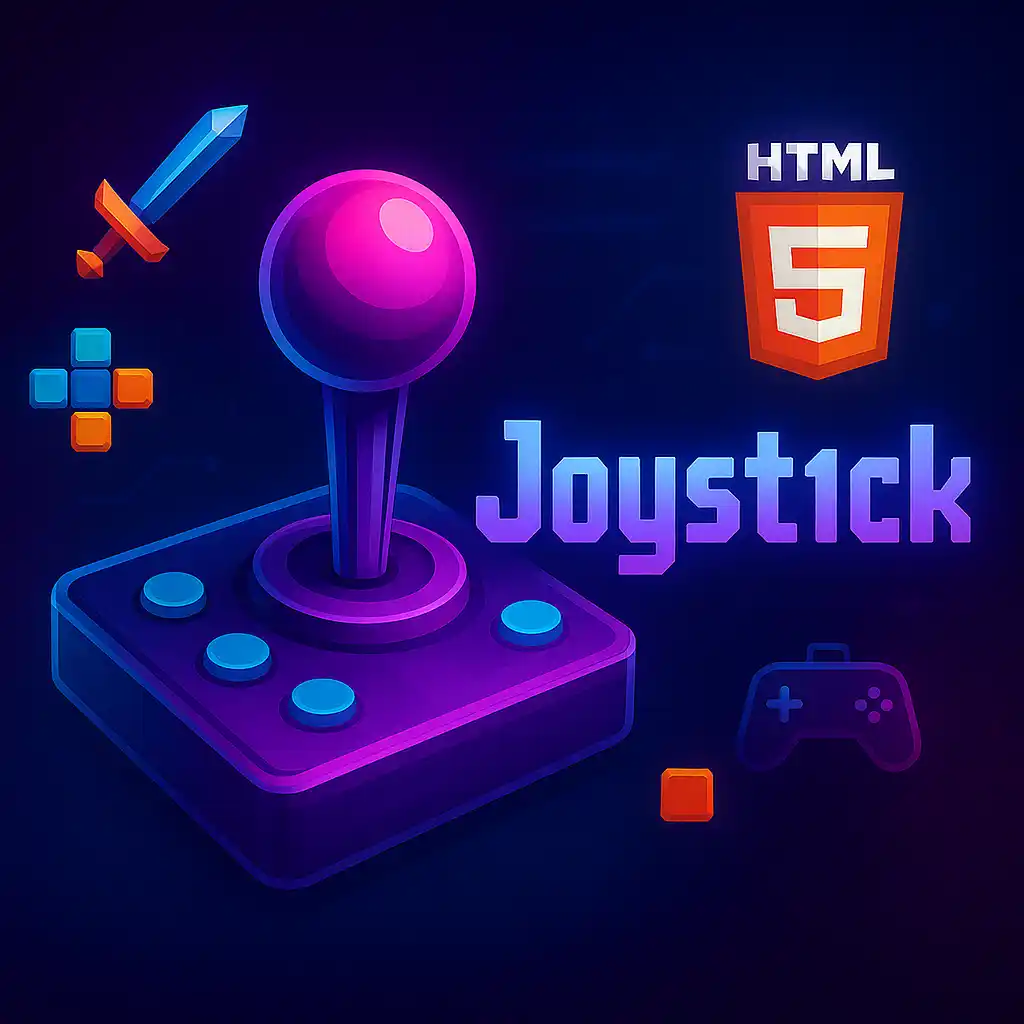