Table of Contents
Implementing Partial Screenshot Features in Game Development
Integrating a partial screenshot feature in a game’s photo mode requires a combination of in-game capture utilities and image processing tools. Here are several strategies and libraries that can assist in implementing this feature:
1. Unity’s Camera and Texture2D Capture
In Unity, you can use the Camera
class for capturing screenshots. By rendering the camera’s output to a RenderTexture
, you can create a custom size capture region:
Play free games on Playgama.com
Camera camera = Camera.main; // Or any other camera
RenderTexture renderTexture = new RenderTexture(1024, 768, 24);
camera.targetTexture = renderTexture;
camera.Render();
RenderTexture.active = renderTexture;
Texture2D image = new Texture2D(256, 256); // Define the required partial capture area
image.ReadPixels(new Rect(10, 10, 256, 256), 0, 0); // Define the capture coordinates
image.Apply();
camera.targetTexture = null;
RenderTexture.active = null;
byte[] bytes = image.EncodeToPNG(); // Convert to bytes to save image
2. Third-party Libraries
- CgToolkit: A great option for advanced image manipulation, which can be leveraged for post-processing screenshots.
- Unity Screenshot API: Consider using this if you need more complex functionalities like capturing from multiple cameras or capturing with effects applied.
3. Snipping & Clipboard Tools
For games running on Windows or requiring OS-level integration, leveraging native capabilities like Snipping Tool can automate part of the job:
- Windows + Shift + S: Utilizes the OS-level screenshot tool for games, supporting clipboard-to-file operations.
4. User Interface (UI) for Selection
Offer players a UI overlay allowing them to select the area they wish to capture. Use Unity’s Canvas
component to draw a draggable rectangle, calculate the screen coordinates, and intersect it with the rendered texture.
Conclusion
By leveraging in-game rendering capabilities alongside native OS tools, developers can provide a robust and flexible partial screenshot feature in their games. Whether using Unity’s built-in methods or extending functionality with third-party libraries, the focus should always be on maximizing user convenience and capture precision.
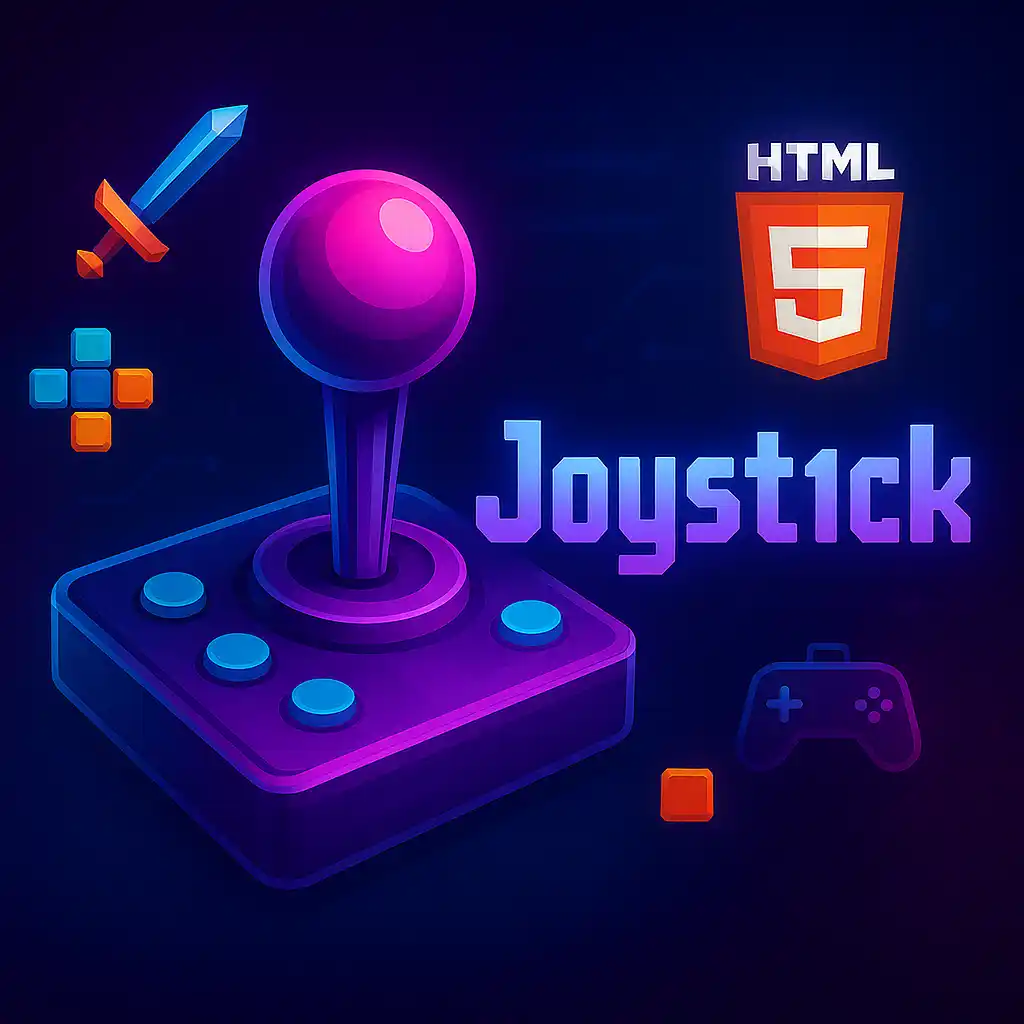