Table of Contents
Best Methods for Integer to String Conversion in C++
When developing a game, displaying scores accurately and efficiently is crucial for the user interface. C++ provides several effective methods for converting integers to strings, each with its advantages depending on the specific requirements.
1. Using std::to_string
Function
The std::to_string
function is part of the C++ standard library and offers a simple way to convert integers to strings:
Play free games on Playgama.com
int score = 12345;
std::string scoreStr = std::to_string(score);
This method is highly efficient and straightforward, making it a go-to choice for quick implementations.
2. Using std::stringstream
The std::stringstream
class provides a versatile method for conversion, especially useful when multiple data types need concatenation alongside formatting:
#include <sstream>
int score = 12345;
std::stringstream ss;
ss << score;
std::string scoreStr = ss.str();
This approach can be slightly less performant than std::to_string
, but offers flexibility for complex formatting needs.
3. Using sprintf
(C-style)
For those who prefer C-style coding, sprintf
can be used though it is generally less safe due to buffer overflow risks:
char buffer[10];
int score = 12345;
sprintf(buffer, "%d", score);
std::string scoreStr(buffer);
While efficient for small-scale apps, caution is advised due to potential security vulnerabilities.
Optimizing C++ UI Display
- Use Caching: Cache frequently used strings to reduce conversion overhead.
- Avoid Redundant Conversions: Convert only when necessary to maintain performance efficiency.
- Minimize Memory Allocation: Using
std::string
efficiently can prevent unnecessary allocation.
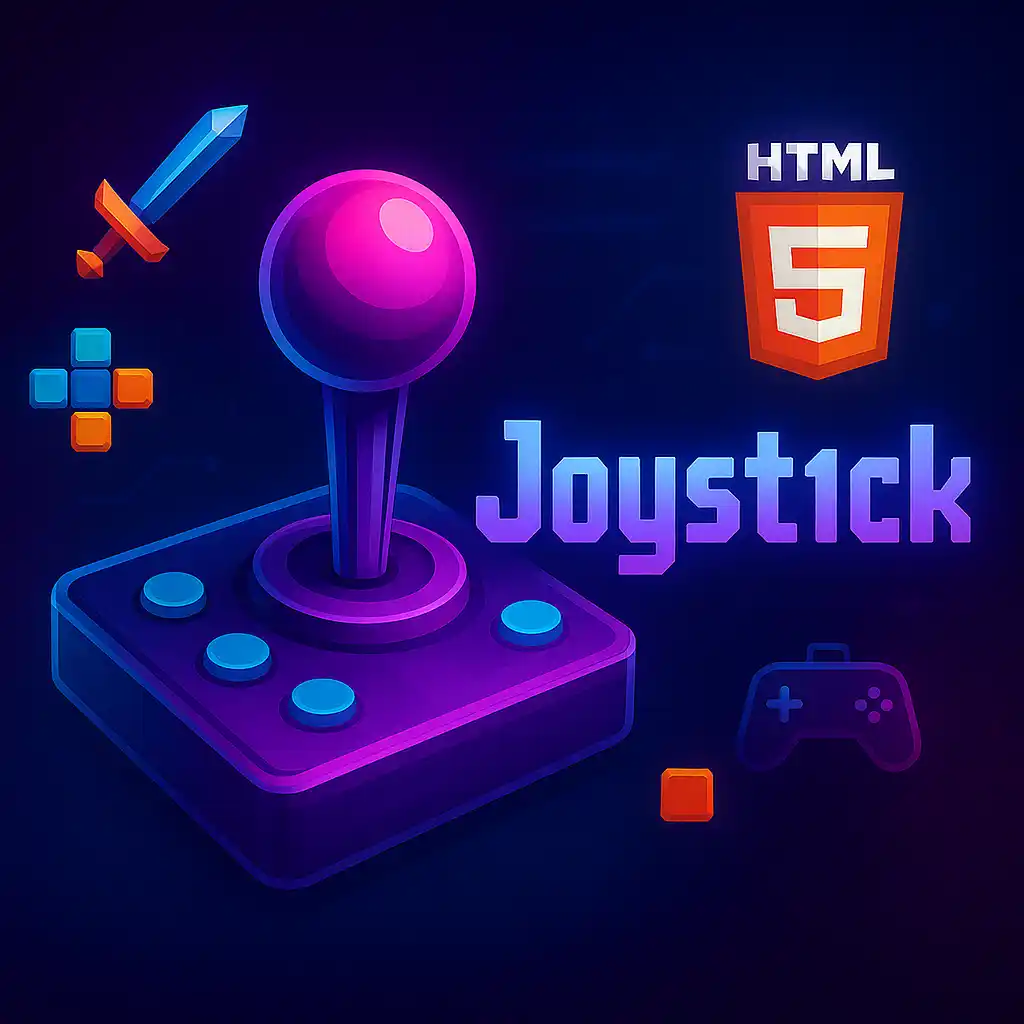