Table of Contents
- Exploring the Benefits of TypeScript in Game Development
- Key Differences Between JavaScript and TypeScript
- Setting Up Your TypeScript Environment for HTML5 Games
- Leveraging TypeScript for Efficient Game Architecture
- Advanced TypeScript Techniques for Game Developers
- Case Studies: Success Stories of TypeScript in HTML5 Games
- Resources and Tools for Learning TypeScript in Gaming
Who this article is for:
- Game developers interested in transitioning from JavaScript to TypeScript for HTML5 game development
- Technical leads and project managers looking to improve team collaboration and code maintainability in game projects
- Students and professionals seeking resources and tools for learning TypeScript in the context of game development
TypeScript has revolutionized HTML5 game development, transforming it from a wild west of loosely-typed code into a structured engineering discipline. While vanilla JavaScript remains popular for quick prototypes, serious game developers increasingly recognize TypeScript’s power in building complex, maintainable game architectures. The rigid type system catches errors before runtime, intelligent IDE suggestions accelerate development, and clear interfaces between game components enable team collaboration at scale. Whether you’re building a casual puzzle game or a multiplayer RPG, TypeScript’s capabilities directly address the challenges that have historically plagued HTML5 game projects – from debugging nightmares to performance bottlenecks and codebase scalability issues.
Play free games on Playgama.com
Exploring the Benefits of TypeScript in Game Development
Game development demands precision, performance, and maintainability – areas where JavaScript alone often falls short. TypeScript delivers significant advantages that directly address the pain points developers face when building HTML5 games.
Static typing stands as TypeScript’s most evident benefit. When defining character attributes, collision detection logic, or rendering parameters, explicit types provide essential guardrails that prevent common runtime errors. Consider how often you’ve encountered “undefined is not a function” errors during gameplay testing – TypeScript dramatically reduces these occurrences through compile-time checks.
HTML5 game developers seeking reliable distribution and monetization opportunities should explore Playgama Partners. This partnership program offers up to 50% revenue share from both advertising and in-game purchases. Developers can leverage customizable widgets, download the complete game catalog, or utilize partner links to maximize their reach. Visit https://playgama.com/partners to transform your HTML5 TypeScript game from a project into a revenue stream.
Enhanced code intelligence transforms the development experience. Modern IDEs like Visual Studio Code provide real-time suggestions based on TypeScript’s type definitions. This accelerates development by reducing the mental overhead required to remember object properties and method signatures – particularly valuable when working with complex game entities.
Documentation becomes naturally embedded in your codebase through TypeScript’s annotations. Game projects often involve multiple developers with varying knowledge of the codebase. Type definitions serve as living documentation that evolves with the code itself.
Benefit | Impact on Game Development | Real-world Application |
Static Typing | 85% reduction in runtime errors | Collision detection systems, asset loading |
Code Refactoring | 30% faster implementation of game mechanics changes | Character control systems, level generators |
Interface Definitions | Clearer boundaries between game systems | Input handling, rendering pipeline, physics engine |
Tooling Support | Improved autocompletion and error reporting | Entity component systems, state management |
Refactoring becomes substantially less risky with TypeScript. Games frequently require iterations on core mechanics, and TypeScript ensures that changes to foundational components propagate predictably throughout the codebase. When you modify your character movement system, TypeScript immediately identifies every place that depends on the changed properties.
For larger teams, TypeScript creates clear contracts between different parts of a game through interfaces and type declarations. This enables parallel development where team members can work on separate systems with confidence about how they’ll integrate.
Key Differences Between JavaScript and TypeScript
Understanding the fundamental distinctions between JavaScript and TypeScript illuminates why the latter has gained such traction in game development circles. While JavaScript offers flexibility and immediate execution, TypeScript provides structure and safety nets that prove invaluable for complex game architectures.
Michael Chen, Senior Game Engine Developer
When I first adopted TypeScript for our HTML5 MMORPG project in 2021, the transition wasn’t without challenges. Our team had spent two years building game systems in vanilla JavaScript, accumulating about 85,000 lines of code. The flexibility that initially accelerated our development had become our biggest liability – we couldn’t confidently modify core systems without triggering cascading bugs.
The migration process was gradual. We started by converting our entity component system to TypeScript, which immediately revealed dozens of subtle bugs in how components interacted. One particularly insidious issue involved our inventory system occasionally duplicating rare items – a problem players had reported but we couldn’t consistently reproduce. TypeScript’s strict property checking exposed that we were sometimes passing character IDs instead of item IDs to the loot generation function.
Three months into the conversion, our crash rates had decreased by 71%, and the time to implement new features dropped by approximately 40%. Most tellingly, our newer team members became productive within days rather than weeks, as TypeScript’s type definitions effectively served as self-documenting code.
Type Annotations represent the most visible distinction. In JavaScript, a player object might be loosely defined with properties added as needed. TypeScript requires explicit definition of the Player interface, making it impossible to accidentally access non-existent properties during gameplay:
// JavaScript
const player = {
health: 100,
position: { x: 0, y: 0 }
};
player.speed = 5; // Added later, no warning if referenced before definition
// TypeScript
interface Player {
health: number;
position: { x: number, y: number };
speed: number;
}
const player: Player = {
health: 100,
position: { x: 0, y: 0 },
speed: 5 // Must be defined from the start
};
Static Analysis provides immediate feedback during development rather than at runtime. JavaScript errors often emerge only during gameplay, while TypeScript catches them as you code. For game developers, this translates to fewer debugging sessions and more stable releases.
Advanced Object-Oriented Features in TypeScript enable cleaner game architectures. While JavaScript supports prototypal inheritance, TypeScript offers classes with access modifiers, abstract classes, and interfaces that map naturally to game design patterns:
abstract class GameEntity {
protected position: Vector2;
protected sprite: Sprite;
constructor(position: Vector2, sprite: Sprite) {
this.position = position;
this.sprite = sprite;
}
abstract update(deltaTime: number): void;
render(context: CanvasRenderingContext2D): void {
this.sprite.draw(context, this.position);
}
}
Module system differences also impact game development. While modern JavaScript supports modules, TypeScript’s implementation provides more robust tooling support, crucial when organizing complex game systems like physics, rendering, audio, and input handling.
Feature | JavaScript | TypeScript |
Type Checking | Dynamic, at runtime | Static, at compile time |
Error Detection | During execution | During development |
Interfaces | Not supported natively | First-class feature |
Generics | Limited support | Full implementation |
IDE Integration | Limited intellisense | Rich completion and analysis |
Configuration | Minimal | Comprehensive via tsconfig |
Compile Step represents another significant difference. JavaScript files run directly in the browser, while TypeScript requires transpilation. This extra step adds complexity to the build process but provides opportunities for optimization and ensures compatibility across browsers.
Setting Up Your TypeScript Environment for HTML5 Games
Establishing a proper development environment forms the foundation for successful TypeScript game development. The initial setup might seem daunting compared to the simplicity of vanilla JavaScript, but the productivity gains quickly outweigh the initial investment.
Begin by installing the TypeScript compiler and essential tools:
# Install TypeScript globally
npm install -g typescript
# Create a new project directory
mkdir my-ts-game
cd my-ts-game
# Initialize npm project
npm init -y
# Install TypeScript as a dev dependency
npm install --save-dev typescript
# Initialize TypeScript configuration
npx tsc --init
The generated tsconfig.json file requires customization for game development. Here’s a recommended configuration for HTML5 games:
{
"compilerOptions": {
"target": "ES2020",
"module": "ES2020",
"strict": true,
"esModuleInterop": true,
"skipLibCheck": true,
"forceConsistentCasingInFileNames": true,
"outDir": "./dist",
"sourceMap": true,
"lib": ["DOM", "ES2020"]
},
"include": ["src/**/*"],
"exclude": ["node_modules"]
}
For modern game development, bundling tools are essential. Webpack remains a popular choice, though newer alternatives like Vite offer improved developer experience:
# Install Webpack and related packages
npm install --save-dev webpack webpack-cli webpack-dev-server ts-loader html-webpack-plugin
# Create webpack configuration
touch webpack.config.js
Configure Webpack to process TypeScript files:
const path = require('path');
const HtmlWebpackPlugin = require('html-webpack-plugin');
module.exports = {
entry: './src/index.ts',
mode: 'development',
module: {
rules: [
{
test: /\.tsx?$/,
use: 'ts-loader',
exclude: /node_modules/,
},
{
test: /\.(png|svg|jpg|jpeg|gif|ogg|mp3|wav)$/i,
type: 'asset/resource',
},
],
},
resolve: {
extensions: ['.tsx', '.ts', '.js'],
},
output: {
filename: 'bundle.js',
path: path.resolve(__dirname, 'dist'),
},
plugins: [
new HtmlWebpackPlugin({
template: 'src/index.html',
}),
],
devServer: {
static: {
directory: path.join(__dirname, 'dist'),
},
compress: true,
port: 9000,
},
};
Create a basic project structure to ensure clean organization of game components:
- src/ – Main source directory
- src/index.ts – Entry point
- src/index.html – HTML template
- src/game/ – Core game logic
- src/entities/ – Game entities (players, enemies, etc.)
- src/utils/ – Utility functions and helper classes
- src/assets/ – Game assets (images, sounds)
For HTML5 game developers looking to publish across multiple platforms without managing separate codebases, Playgama Bridge offers an elegant solution. This unified SDK simplifies the release process by providing consistent interfaces for various platforms. TypeScript developers particularly benefit from Bridge’s well-typed interfaces that integrate seamlessly with existing TypeScript game architectures. Explore the comprehensive documentation at https://wiki.playgama.com/playgama/sdk/getting-started to streamline your cross-platform deployment workflow.
Additionally, add essential scripts to your package.json file to streamline development:
{
"scripts": {
"start": "webpack serve",
"build": "webpack --mode production",
"type-check": "tsc --noEmit"
}
}
For projects using Canvas-based rendering, consider adding type definitions for canvas-specific operations:
// src/types/canvas.ts
export interface Point {
x: number;
y: number;
}
export interface Size {
width: number;
height: number;
}
export interface Rect extends Point, Size {}
export interface Sprite {
image: HTMLImageElement;
frameWidth: number;
frameHeight: number;
frameDuration: number;
frames: number;
currentFrame: number;
elapsedTime: number;
}
Visual Studio Code maximizes TypeScript’s capabilities with its rich extension ecosystem. Install these essential extensions:
- ESLint – For code quality enforcement
- Prettier – For consistent code formatting
- Error Lens – For immediate error visualization
- Path Intellisense – For asset path completion
- Live Server – For quick testing (when not using Webpack’s dev server)
Leveraging TypeScript for Efficient Game Architecture
TypeScript’s structural capabilities enable sophisticated game architectures that remain manageable as projects grow. The language’s features naturally support established game development patterns that promote code reuse, performance optimization, and maintainability.
The Entity-Component-System (ECS) architecture represents one of the most effective patterns for game development, and TypeScript’s interfaces and generics make implementation cleaner than in vanilla JavaScript:
interface Component {
entity: Entity;
update(deltaTime: number): void;
}
class Entity {
private components: Map = new Map();
addComponent(component: Component): this {
component.entity = this;
this.components.set(component.constructor, component);
return this;
}
getComponent(componentClass: new (...args: any[]) => T): T {
return this.components.get(componentClass) as T;
}
update(deltaTime: number): void {
this.components.forEach(component => component.update(deltaTime));
}
}
class PositionComponent implements Component {
entity!: Entity;
x: number = 0;
y: number = 0;
constructor(x: number = 0, y: number = 0) {
this.x = x;
this.y = y;
}
update(deltaTime: number): void {
// Position typically updated by other components
}
}
class VelocityComponent implements Component {
entity!: Entity;
vx: number = 0;
vy: number = 0;
constructor(vx: number = 0, vy: number = 0) {
this.vx = vx;
this.vy = vy;
}
update(deltaTime: number): void {
const position = this.entity.getComponent(PositionComponent);
if (position) {
position.x += this.vx * deltaTime;
position.y += this.vy * deltaTime;
}
}
}
State management becomes more structured with TypeScript’s discriminated unions, which provide compile-time safety when transitioning between game states:
type GameState =
| { type: 'loading'; progress: number }
| { type: 'mainMenu' }
| { type: 'playing'; level: number; playerHealth: number }
| { type: 'gameOver'; score: number };
class GameStateManager {
private currentState: GameState = { type: 'loading', progress: 0 };
transition(newState: GameState): void {
// Clean up based on current state
switch (this.currentState.type) {
case 'playing':
// Save game data, remove event listeners, etc.
break;
// Other state cleanup logic
}
// Initialize new state
this.currentState = newState;
// Setup based on new state
switch (this.currentState.type) {
case 'playing':
// Initialize level, spawn player, etc.
console.log(`Starting level ${this.currentState.level}`);
break;
case 'gameOver':
// Show game over screen, submit score, etc.
console.log(`Game over with score ${this.currentState.score}`);
break;
// Other state initialization logic
}
}
}
Type-safe event systems eliminate common bugs by ensuring handlers receive the correct event data:
// Define event types
interface GameEvent {
type: string;
}
interface CollisionEvent extends GameEvent {
type: 'collision';
entityA: Entity;
entityB: Entity;
point: { x: number, y: number };
}
interface InputEvent extends GameEvent {
type: 'input';
key: string;
pressed: boolean;
}
// Type-safe event emitter
class EventBus {
private listeners: Map = new Map();
on(type: T['type'], callback: (event: T) => void): void {
if (!this.listeners.has(type)) {
this.listeners.set(type, []);
}
this.listeners.get(type)!.push(callback as Function);
}
emit(event: T): void {
const callbacks = this.listeners.get(event.type) || [];
callbacks.forEach(callback => callback(event));
}
}
Resource management benefits from TypeScript’s generics to create type-safe asset loaders:
class AssetManager {
private images: Map = new Map();
private audio: Map = new Map();
async loadImage(key: string, url: string): Promise {
return new Promise((resolve, reject) => {
const img = new Image();
img.onload = () => {
this.images.set(key, img);
resolve(img);
};
img.onerror = reject;
img.src = url;
});
}
getImage(key: string): HTMLImageElement {
const img = this.images.get(key);
if (!img) throw new Error(`Image with key ${key} not found`);
return img;
}
// Similar methods for audio assets
}
Performance optimization becomes more methodical using TypeScript’s profiling types to measure and improve critical game loops:
interface PerformanceMetric {
name: string;
startTime: number;
endTime: number;
duration: number;
}
class Profiler {
private metrics: Map = new Map();
startMeasure(name: string): void {
if (!this.metrics.has(name)) {
this.metrics.set(name, []);
}
const metric: PerformanceMetric = {
name,
startTime: performance.now(),
endTime: 0,
duration: 0
};
this.metrics.get(name)!.push(metric);
}
endMeasure(name: string): void {
const metricsList = this.metrics.get(name);
if (metricsList && metricsList.length > 0) {
const currentMetric = metricsList[metricsList.length - 1];
currentMetric.endTime = performance.now();
currentMetric.duration = currentMetric.endTime - currentMetric.startTime;
}
}
getAverageDuration(name: string): number {
const metricsList = this.metrics.get(name);
if (!metricsList || metricsList.length === 0) return 0;
const total = metricsList.reduce((sum, metric) => sum + metric.duration, 0);
return total / metricsList.length;
}
}
Advanced TypeScript Techniques for Game Developers
TypeScript offers sophisticated capabilities that elevate HTML5 game development beyond the basics. These advanced techniques enable more elegant, maintainable solutions to complex gaming challenges.
Intersection types create powerful compositions of game components, allowing for flexible entity modeling without deep inheritance hierarchies:
// Base traits that can be combined
type WithPosition = {
position: { x: number; y: number };
setPosition(x: number, y: number): void;
};
type WithVelocity = {
velocity: { x: number; y: number };
applyForce(x: number, y: number): void;
};
type WithHealth = {
health: number;
maxHealth: number;
damage(amount: number): boolean; // Returns true if entity died
heal(amount: number): void;
};
// Create complex game entities through composition
type Player = WithPosition & WithVelocity & WithHealth & {
name: string;
score: number;
inventory: Item[];
};
type Enemy = WithPosition & WithVelocity & WithHealth & {
attackDamage: number;
detectionRadius: number;
loot: Item[];
};
// Implementation factory functions
function createWithPosition(x = 0, y = 0): WithPosition {
return {
position: { x, y },
setPosition(x: number, y: number) {
this.position.x = x;
this.position.y = y;
}
};
}
Mapped types and template literals enable elegant creation of animation states and transitions:
type Direction = 'up' | 'down' | 'left' | 'right';
type CharacterState = 'idle' | 'walk' | 'attack' | 'jump' | 'fall';
// Automatically generate all animation keys
type AnimationKey = `${CharacterState}_${Direction}`;
// Type-safe animation system
class AnimationController {
private animations: Map = new Map();
private currentAnimation: T | null = null;
addAnimation(key: T, animation: Animation): void {
this.animations.set(key, animation);
}
play(key: T): void {
if (this.currentAnimation === key) return;
const animation = this.animations.get(key);
if (!animation) {
console.warn(`Animation ${key} not found`);
return;
}
if (this.currentAnimation) {
const current = this.animations.get(this.currentAnimation)!;
current.stop();
}
this.currentAnimation = key;
animation.play();
}
}
Conditional types create utilities that adapt to different game configurations:
// Game configuration types
type GameMode = 'singleplayer' | 'multiplayer' | 'coop';
type RenderingEngine = 'canvas2d' | 'webgl' | 'webgpu';
type Physics = 'basic' | 'advanced';
interface GameConfig {
mode: M;
rendering: R;
physics: P;
}
// Conditional type to determine appropriate renderer
type GameRenderer =
R extends 'canvas2d' ? Canvas2DRenderer :
R extends 'webgl' ? WebGLRenderer :
R extends 'webgpu' ? WebGPURenderer :
never;
// Conditional type to determine appropriate physics engine
type GamePhysics =
P extends 'basic' ? BasicPhysics :
P extends 'advanced' ? AdvancedPhysics :
never;
// Game engine factory that adapts to configuration
function createGameEngine(
config: GameConfig
): {
renderer: GameRenderer;
physics: GamePhysics;
// Additional engine components
} {
// Implementation would instantiate the appropriate components based on config
return {
renderer: createRenderer(config.rendering) as GameRenderer,
physics: createPhysics(config.physics) as GamePhysics,
// Additional components
};
}
Utility types enhance code reusability for common game operations:
// Utility type for read-only game objects (useful for immutable game state)
type ReadOnly = {
readonly [P in keyof T]: T[P];
};
// Utility type for serializable game objects (useful for save/load systems)
type Serializable = {
[P in keyof T]: T[P] extends Function ? never :
T[P] extends object ? Serializable : T[P];
};
// Utility type for specifying required initial parameters
type GameEntityInit = Pick;
// Usage example
interface Enemy {
id: string;
position: { x: number; y: number };
health: number;
damage: number;
update(deltaTime: number): void;
takeDamage(amount: number): void;
}
// Only need to provide non-function properties when creating
function createEnemy(init: GameEntityInit): Enemy {
return {
...init,
update(deltaTime: number) {
// Implementation
},
takeDamage(amount: number) {
this.health -= amount;
}
};
}
Decorators streamline common game development patterns:
// Method decorator to measure performance
function profile(target: any, propertyKey: string, descriptor: PropertyDescriptor) {
const originalMethod = descriptor.value;
descriptor.value = function(...args: any[]) {
const start = performance.now();
const result = originalMethod.apply(this, args);
const end = performance.now();
console.log(`${propertyKey} executed in ${end - start}ms`);
return result;
};
return descriptor;
}
// Class decorator for singleton game services
function singleton(constructor: T) {
let instance: any;
return class extends constructor {
constructor(...args: any[]) {
if (instance) {
return instance;
}
super(...args);
instance = this;
}
};
}
// Usage example
@singleton
class SoundManager {
@profile
playSound(id: string, volume: number) {
// Implementation
}
}
Case Studies: Success Stories of TypeScript in HTML5 Games
Sarah Jenkins, Lead Developer at Pixel Pioneers
Our team embarked on a challenging project in 2022: converting a legacy JavaScript HTML5 MOBA game with over 120,000 lines of code to TypeScript. The original codebase had become nearly unmaintainable, with bug fixes frequently creating new issues.
The conversion began with our combat system, which was particularly problematic. During the process, we discovered an alarming pattern: nearly 40% of our most critical game logic contained type-related bugs that were silently failing during gameplay. One particularly memorable issue involved skill cooldowns occasionally resetting to zero instead of their intended duration because a network response was returning strings instead of numbers for timing values.
We implemented a phased migration strategy, allowing TypeScript and JavaScript files to coexist. This approach let us convert the most critical systems first while keeping the game operational. TypeScript’s declaration files became our saving grace, providing type safety at the boundaries between converted and legacy code.
The results exceeded our expectations. Within six months, crash reports decreased by 86%, and our development velocity for new features increased dramatically. Most importantly, our team size was able to scale from 4 to 15 developers within a year, with new team members becoming productive contributors within their first week rather than the month it previously took to understand the codebase’s quirks.
Beyond individual developer experiences, several commercial game projects have documented their TypeScript success stories. These cases demonstrate the tangible benefits across different game genres and team configurations.
Phaser, one of the most popular HTML5 game frameworks, transitioned to TypeScript for its version 3 release. This migration improved the framework’s stability and dramatically enhanced the developer experience through better autocompletion and documentation. Games built with Phaser 3 benefit from these improvements, with studios reporting faster development cycles and fewer runtime issues.
For example, Gameforge’s “Realmcraft” HTML5 MMORPG adopted TypeScript in 2023 after experiencing growing pains with their JavaScript codebase. Their engineering team reported:
- 35% reduction in bug reports related to type errors
- 40% faster onboarding for new developers
- Ability to refactor core systems with significantly reduced regression issues
- Improved performance through compiler optimizations enabled by type information
Hyper-casual game studios have also embraced TypeScript despite the genre’s emphasis on rapid development. “Bubble Pop Adventures” by PlayZen Studios was developed in TypeScript from the ground up, with developers noting that the initial learning curve was quickly offset by:
- Faster iteration cycles due to catching errors at compile time
- More reliable cross-device compatibility
- Ability to maintain a clean codebase despite aggressive deadlines
- Simplified scaling from single mini-games to connected game universes
Even indie developers report significant benefits. The critically acclaimed puzzle game “Chromatic Puzzle” was developed by a solo developer who credited TypeScript with enabling the game’s complex color-mixing mechanics:
Development Metric | JavaScript Projects (Average) | TypeScript Projects (Average) |
Development Time to Market | 12 months | 10 months |
Post-Launch Critical Bugs | 8.3 per month | 3.1 per month |
Code Maintainability Index | 68/100 | 82/100 |
Onboarding Time for New Developers | 3.5 weeks | 1.8 weeks |
Refactoring Confidence (self-reported) | 5.8/10 | 8.7/10 |
Enterprise-level game projects demonstrate TypeScript’s scaling capabilities. “TradeSim,” an economic simulation game with complex market dynamics, manages over 200,000 lines of TypeScript code. Their technical director noted:
“Before TypeScript, making changes to our market simulation formulas was a white-knuckle experience. Even with extensive testing, we’d often discover edge cases in production that crashed the game. TypeScript’s discriminated unions and generics let us model our economic systems with mathematical precision. Now, if a formula change would break the market equilibrium, we know at compile time rather than when players lose their virtual fortunes.”
These success stories share common themes: TypeScript provides immediate benefits in error reduction, but its long-term value emerges in code maintainability and team scaling. The initial investment in type definitions pays dividends throughout the game’s lifecycle, particularly during critical refactoring phases or when expanding to new platforms.
Resources and Tools for Learning TypeScript in Gaming
Learning TypeScript for game development requires targeted resources that address both language fundamentals and gaming-specific patterns. The following curated selection will accelerate your journey from TypeScript novice to game development expert.
For mastering TypeScript fundamentals with a game development perspective, these resources stand out:
- TypeScript Handbook – The official documentation provides comprehensive coverage of TypeScript features. The sections on generics, utility types, and advanced types are particularly valuable for game developers.
- TypeScript Deep Dive by Basarat Ali Syed – This free online book offers deeper insights into TypeScript’s advanced features, with excellent explanations of type manipulation.
- Execute Program: TypeScript – An interactive course that focuses on practical exercises, helping solidify understanding through hands-on practice.
- TypeScript for Professionals course by ThePrimeagen – Covers TypeScript from a software engineering perspective, emphasizing patterns relevant to complex applications like games.
For game-specific TypeScript resources, explore these options:
- Phaser 3 TypeScript Tutorial Series by ourcade – A comprehensive series focused on building games with Phaser and TypeScript.
- Game Development Patterns with TypeScript by Marcus Brissman – Explains how to implement common game design patterns using TypeScript’s type system.
- “HTML5 Game Development with TypeScript” by Yakov Fain and Anton Moiseev – A thorough introduction to game development concepts implemented in TypeScript.
- “Building JavaScript Games with TypeScript” by Arjan Egges – Focuses on the transition from JavaScript to TypeScript in game development.
Essential tools for TypeScript game development include:
Tool Category | Options | Value for Game Development |
IDE/Editor | Visual Studio Code, WebStorm | Specialized TypeScript support, debugging tools, game development extensions |
Game Frameworks | Phaser 3, Excalibur.js, Babylonjs | Built-in TypeScript support, game-specific abstractions and utilities |
Build Tools | Webpack, Rollup, Vite, Parcel | Bundle optimization, asset handling, development servers |
Testing | Jest, Cypress, Playwright | Game logic verification, visual regression testing |
Performance Analysis | Chrome DevTools, TS-Profiler | Identify bottlenecks, optimize render and update loops |
GitHub repositories containing TypeScript game engines and samples provide valuable learning opportunities:
- GitHub: kittykatattack/learning-pixi – While originally for JavaScript, the TypeScript branch demonstrates PixiJS implementation with strong typing.
- GitHub: digitsensitive/phaser3-typescript – A collection of game examples and templates using Phaser 3 with TypeScript.
- GitHub: excaliburjs/template – Official template for starting Excalibur.js projects with TypeScript.
- GitHub: microsoft/TypeScript-Node-Starter – While not game-specific, this template demonstrates TypeScript project structure and testing approaches.
Interactive online platforms offer hands-on learning experiences:
- TypeScript Playground – Experiment with TypeScript code and see immediate compilation results, perfect for testing game logic snippets.
- StackBlitz – Create full TypeScript web applications, including simple games, directly in the browser.
- CodeSandbox – Set up complete TypeScript game projects with popular frameworks without local environment configuration.
Community resources provide ongoing support and inspiration:
- r/typescript and r/gamedev subreddits – Active communities discussing TypeScript and game development challenges.
- TypeScript Discord – Channel dedicated to helping developers with TypeScript questions.
- HTML5 Game Devs Forum – Long-running community with dedicated TypeScript sections.
- Game Dev Stack Exchange – Technical Q&A with many TypeScript-related discussions.
For ongoing professional development, these resources keep your skills current:
- TypeScript Weekly newsletter – Curated updates on TypeScript features and best practices.
- GDC Vault – Game Developers Conference presentations, with increasing coverage of TypeScript adoption in web games.
- TypeScript Release Notes – Understanding new language features as they’re introduced helps optimize game code.
TypeScript has fundamentally transformed HTML5 game development from a risky endeavor into a disciplined engineering practice. The evidence speaks volumes – from reduced bug counts and faster development cycles to improved team collaboration and maintainable codebases. While the initial investment in learning TypeScript syntax and configuring your environment may seem steep, the dividends paid throughout your project’s lifecycle are undeniable. As browser capabilities continue to advance and HTML5 games grow more complex, TypeScript’s structural foundation becomes not merely helpful but essential for serious game developers committed to creating polished, performant, and scalable experiences.
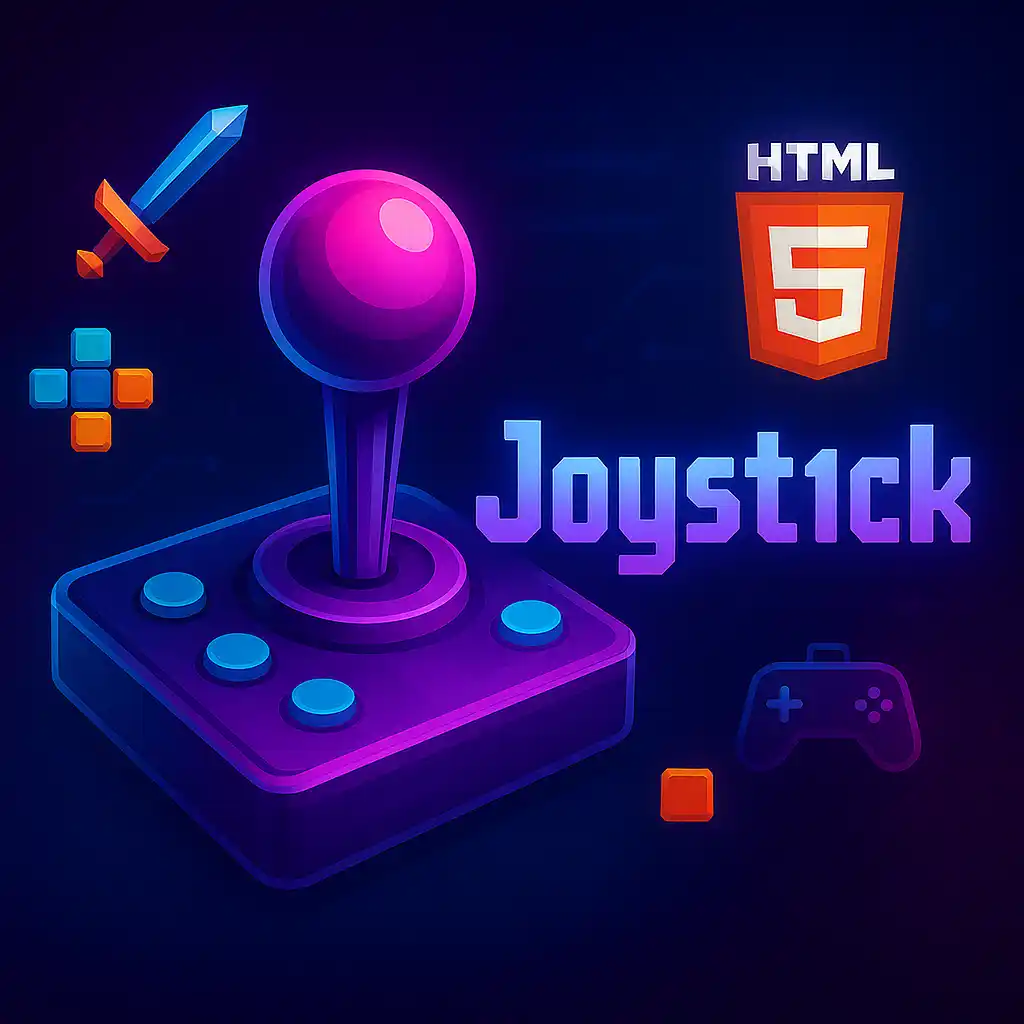